class: center, middle, inverse, title-slide # A quick tour of R ### Abhijit Dasgupta, PhD --- layout: true <div class="my-header"> <span>BIOF 439: Data Visualization using R</span></div> --- ## The bare essentials - R is a **language** - Everything in R is an object with a name -- - Things are nouns - Nouns in R are _data objects_, like scalars, matrices, data.frames/tibbles, strings, vectors - Nouns are acted upon by verbs - Verbs in R are _functions_, like `mean(x)`, `nrow(d)`, `dim(d)`, `ggplot` and so on - You can modify verbs with adverbs - Adverbs in R are _function options_, like `mean(x, na.rm=T)`, `geom_point(color='green')` --- ## The bare essentials - If you want to save and re-use an object (noun or verb), you have to name it - This is done with the `<-` operator, e.g. - `beaches <- read_csv('data/sydneybeaches3.csv')` (dataset) - `mn <- mean(x, na.rm=T)` (a number) - `my_theme <- function() {theme_bw() + theme(axis.title=element_text(size=14))}` stores the function, which you'll call as `my_theme()` > You can see the objects you have created either by typing `ls()` in the console, or looking in the Environment pane > Note, built-in objects don't show up in the Environment pane or using `ls()` --- ## The bare essentials ### Naming rules 1. Identifiers can be a combination of letters, digits, period (.) and underscore (_). 1. It must start with a letter or a period. If it starts with a period, it cannot be followed by a digit. 1. Reserved words in R cannot be used as identifiers. -- ### Naming conventions 1. Make names expressive 1. Write multi-word names in CamelCase or snake_case --- ## The bare essentials ### Allowing non-conventional names If a name doesn't follow the rules *per se*, it can still be used by quoting them using backticks. So the following names are valid, as long as backticks are present. ``` `Pelvic girdle` `1` `Sample 03/23/2019` ``` This is rather difficult to type on a regular basis, and can lead to syntactical errors ---- The **janitor** package has a `clean_names` function that can fix the names of columns when you import datasets, transforming them into snake case by default. --- ## The bare essentials (brackets) `[]` are used for extracting elements from arrays, matrices, data frames. - `x[3]` is the 3rd element of an array `x` - `d[1,3]` is the element in the 1st row and 3rd column of a matrix/data frame `d` - `d[2,]` is the entire first __row__ of a matrix/data frame `d` - `d[,4]` is the entire 4th __column__ of a matrix/data frame `d` - `d[,'gender']` is the column named _gender_ in a _data frame_ `d` For a list object (we'll meet them in a few slides) you use a double bracket to extract elements - `lst[[3]]` is the third element of the list `lst` - `lst[['apple']]` is the element named _apple_ in a named list `lst` --- ## The bare essentials (brackets) There are three kinds of brackets in R `()` are used for specifying arguments to functions - `mean(x)` gives the mean of an array of numbers `x` - `summary(d)` gives a summary representation of a data frame `d` - There are several functions used to make the following visualization ```r ggplot(beaches, aes(x = temperature, y = rainfall)) + geom_point() + geom_smooth() + theme_classic() ``` --- ## The bare essentials (brackets) There are three kinds of brackets in R `{}` are used to contain groups of commands/statements .pull-left[ A conditional statement ```r if (age < 18){ person <- 'Minor' } else if (age > 65) { person <- 'Senior' } else { person <- 'Adult' } ``` ] .pull-right[ A function definition ```r my_mean <- function(x, na.rm = T){ if(na.rm){ x <- x[!is.na(x)] } s <- sum(x) n <- length(x) mn <- s / n # There is a built-in function mean, so I don't use that word return(mn) } ``` ] See reading and supplementary materials for more details about these constructs --- ## Data structures A __scalar__ (data taking a single value) - `29` - `"cherry"` - `TRUE` --- ## Data structures A __scalar__ (data taking a single value) - `29` : _numeric_ - `"cherry"`: _character_ - `TRUE`: _logical_ ---- These are different data *types*, which determine how they are stored in memory. You may also see _integer_ and _double_, which are both covered under _numeric_. You can check an object's type using `class`: `class(29)` (resulting in `numeric`) <br/> You can check if an object is of a particular type: `is.character(29)` (resulting in `FALSE`)<br/> You can transform an object to a different type, if allowed: `as.numeric("29")` (resulting in `29`)<p> The `is.___` and `as.___` functions are extremely useful for data cleaning and transformations<p> You typically use the variable names rather than the actual values for these functions --- ## Data structures ### Vectors/Arrays These are constructed using the `c()` function (for _concatenate_). .pull-left[ ```r c(1,2,5,6,7,8) ``` ``` #> [1] 1 2 5 6 7 8 ``` ```r c('apple','berry','melon','citrus') ``` ``` #> [1] "apple" "berry" "melon" "citrus" ``` ] .pull-right[ Vectors must all contain objects of the same type. Can't mix and match<p> If you check the `class` of an array, it will give the common data type of the elements of the array ] --- ## Data structures ### Matrices (2-d arrays) These are typically built from vectors ```r x <- c(1,2,4,5,6,7) y <- 10:16 # Shortcut for c(10,11,12,13,14,15,16) ``` .pull-left[ ```r cbind(x, y) # Vectors as columns ``` ``` #> x y #> [1,] 1 10 #> [2,] 2 11 #> [3,] 4 12 #> [4,] 5 13 #> [5,] 6 14 #> [6,] 7 15 #> [7,] 1 16 ``` ] .pull-right[ ```r rbind(x, y) # Vectors as rows ``` ``` #> [,1] [,2] [,3] [,4] [,5] [,6] [,7] #> x 1 2 4 5 6 7 1 #> y 10 11 12 13 14 15 16 ``` ] --- ## Data structures ### Matrices (2-d arrays) You can also build matrices directly using the `matrix` function. ```r matrix(seq(1, 6), nrow = 3) # Automatically determines number of columns ``` ``` #> [,1] [,2] #> [1,] 1 4 #> [2,] 2 5 #> [3,] 3 6 ``` ---- Play around with different ways of creating vectors and matrices --- ## Data structures ### Lists Lists are basically buckets or containers. Each element of a list can be anything, even other lists .pull-left[ ```r my_list <- list('a', c(2,3,5,6), head(ggplot2::mpg)) my_list ``` This has a scalar, a vector and a data set<p> Since lists can be composed of heterogeneous objects, the `class` of a list is `list`. ] .pull-right[ ``` #> [[1]] #> [1] "a" #> #> [[2]] #> [1] 2 3 5 6 #> #> [[3]] #> # A tibble: 6 x 11 #> manufacturer model displ year cyl trans drv cty hwy fl class #> <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> #> 1 audi a4 1.8 1999 4 auto(l5) f 18 29 p compa… #> 2 audi a4 1.8 1999 4 manual(m5) f 21 29 p compa… #> 3 audi a4 2 2008 4 manual(m6) f 20 31 p compa… #> 4 audi a4 2 2008 4 auto(av) f 21 30 p compa… #> 5 audi a4 2.8 1999 6 auto(l5) f 16 26 p compa… #> 6 audi a4 2.8 1999 6 manual(m5) f 18 26 p compa… ``` ] --- ## Data structures ### Lists You can create named lists, where every element has a name ```r my_list2 <- list('scalar' = 'a', 'vector' = c(2,3,5,6), 'data' = head(ggplot2::mpg)) my_list2[['vector']] ``` ``` #> [1] 2 3 5 6 ``` ```r my_list2[['data']] ``` ``` #> # A tibble: 6 x 11 #> manufacturer model displ year cyl trans drv cty hwy fl class #> <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> #> 1 audi a4 1.8 1999 4 auto(l5) f 18 29 p compa… #> 2 audi a4 1.8 1999 4 manual(m5) f 21 29 p compa… #> 3 audi a4 2 2008 4 manual(m6) f 20 31 p compa… #> 4 audi a4 2 2008 4 auto(av) f 21 30 p compa… #> 5 audi a4 2.8 1999 6 auto(l5) f 16 26 p compa… #> 6 audi a4 2.8 1999 6 manual(m5) f 18 26 p compa… ``` --- ## Data structures ### data.frame/tibble This is the typical container for tabular data - must be rectangular - each column can be of a different type - elements within each column have to be of the same type ---- The data frame is probably the single most important structure in R for data analysis. It allows you to keep different kinds of data for each observation together, regardless of type. Many other scripting systems couldn't keep heterogeneous data together, which made practical data analyses very difficult. <p> This kind of structure is now present in almost every serious data analytic platform, including Python, Julia, SAS, SPSS. Matlab remains a significant exception. --- ## Data structures ### data.frame/tibble ```r # use the import function from the package rio beaches <- rio::import('data/sydneybeaches3.csv') class(beaches) ``` ``` #> [1] "data.frame" ``` ```r dim(beaches) ``` ``` #> [1] 344 12 ``` ```r head(beaches) ``` ``` #> date year month day season rainfall temperature enterococci day_num #> 1 2013-01-02 2013 1 2 1 0.0 23.4 6.7 2 #> 2 2013-01-06 2013 1 6 1 0.0 30.3 2.0 6 #> 3 2013-01-12 2013 1 12 1 0.0 31.4 69.1 12 #> 4 2013-01-18 2013 1 18 1 0.0 46.4 9.0 18 #> 5 2013-01-24 2013 1 24 1 0.0 27.5 33.9 24 #> 6 2013-01-30 2013 1 30 1 0.6 26.6 26.5 30 #> month_num month_name season_name #> 1 1 January Summer #> 2 1 January Summer #> 3 1 January Summer #> 4 1 January Summer #> 5 1 January Summer #> 6 1 January Summer ``` --- ## Data structures ### data.frame/tibble ```r library(tidyverse) # Activate the tidyverse package beaches_t <- as_tibble(beaches) class(beaches_t) ``` ``` #> [1] "tbl_df" "tbl" "data.frame" ``` ```r beaches_t ``` ``` #> # A tibble: 344 x 12 #> date year month day season rainfall temperature enterococci day_num #> <date> <int> <int> <int> <int> <dbl> <dbl> <dbl> <int> #> 1 2013-01-02 2013 1 2 1 0 23.4 6.7 2 #> 2 2013-01-06 2013 1 6 1 0 30.3 2 6 #> 3 2013-01-12 2013 1 12 1 0 31.4 69.1 12 #> 4 2013-01-18 2013 1 18 1 0 46.4 9 18 #> 5 2013-01-24 2013 1 24 1 0 27.5 33.9 24 #> 6 2013-01-30 2013 1 30 1 0.6 26.6 26.5 30 #> 7 2013-02-05 2013 2 5 1 0.1 25.7 66.9 36 #> 8 2013-02-11 2013 2 11 1 8 22.2 118. 42 #> 9 2013-02-17 2013 2 17 1 13.6 26.3 75 48 #> 10 2013-02-23 2013 2 23 1 7.2 24.8 311. 54 #> # … with 334 more rows, and 3 more variables: month_num <int>, #> # month_name <chr>, season_name <chr> ``` --- ## Data structures ### data.frame/tibble We can take a quick look at the data types of each column .pull-left[ ```r str(beaches) ``` ``` #> 'data.frame': 344 obs. of 12 variables: #> $ date : IDate, format: "2013-01-02" "2013-01-06" ... #> $ year : int 2013 2013 2013 2013 2013 2013 2013 2013 2013 2013 ... #> $ month : int 1 1 1 1 1 1 2 2 2 2 ... #> $ day : int 2 6 12 18 24 30 5 11 17 23 ... #> $ season : int 1 1 1 1 1 1 1 1 1 1 ... #> $ rainfall : num 0 0 0 0 0 0.6 0.1 8 13.6 7.2 ... #> $ temperature: num 23.4 30.3 31.4 46.4 27.5 26.6 25.7 22.2 26.3 24.8 ... #> $ enterococci: num 6.7 2 69.1 9 33.9 ... #> $ day_num : int 2 6 12 18 24 30 36 42 48 54 ... #> $ month_num : int 1 1 1 1 1 1 2 2 2 2 ... #> $ month_name : chr "January" "January" "January" "January" ... #> $ season_name: chr "Summer" "Summer" "Summer" "Summer" ... ``` ] .pull-right[ ```r glimpse(beaches) ``` ``` #> Rows: 344 #> Columns: 12 #> $ date <date> 2013-01-02, 2013-01-06, 2013-01-12, 2013-01-18, 2013-01-… #> $ year <int> 2013, 2013, 2013, 2013, 2013, 2013, 2013, 2013, 2013, 201… #> $ month <int> 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 3, 4, 4, 4, 4, … #> $ day <int> 2, 6, 12, 18, 24, 30, 5, 11, 17, 23, 1, 7, 13, 19, 25, 2,… #> $ season <int> 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 2, 2, 2, 2, 2, … #> $ rainfall <dbl> 0.0, 0.0, 0.0, 0.0, 0.0, 0.6, 0.1, 8.0, 13.6, 7.2, 28.6, … #> $ temperature <dbl> 23.4, 30.3, 31.4, 46.4, 27.5, 26.6, 25.7, 22.2, 26.3, 24.… #> $ enterococci <dbl> 6.700000, 2.000000, 69.100000, 9.000000, 33.900000, 26.50… #> $ day_num <int> 2, 6, 12, 18, 24, 30, 36, 42, 48, 54, 60, 66, 72, 78, 84,… #> $ month_num <int> 1, 1, 1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 3, 4, 4, 4, 4, … #> $ month_name <chr> "January", "January", "January", "January", "January", "J… #> $ season_name <chr> "Summer", "Summer", "Summer", "Summer", "Summer", "Summer… ``` ] --- ## Data structures ### data.frame/tibble Extracting columns from a data frame: 1. .fatinline[`beaches$temperature`] (works during exploration or when only single column needed) 1. .heatinline[`beaches[,'temperature']`] (*This is my preferred method*) 1. `beaches[['temperature']]` 1. `beaches[,7]` 1. `beaches[[7]]` --- ## Packages in R R is a modular environment with some base functionality that is augmented by __packages__ (think of them as modules) - Packages can contain _functions_ and _data_ - There are over 15K packages on CRAN, the Comprehensive R Archive Network - There are over 1600 packages on Bioconductor, the main repository for bioinformatics resources - Analytic, Annotation, Experimental data and Workflow packages -- #### Finding packages 1) CRAN [Task views](https://cran.r-project.org/web/views/) 2) Bioconductor [BiocViews](http://bioconductor.org/packages/release/BiocViews.html) 3) GitHub (open source collaboration and version control environment) --- ## Installing packages .pull-left[ ### From CRAN ```r install.packages("tidyverse") ``` ### From Bioconductor ```r install.packages("BiocManager") # do once BioCManager::install('limma') ``` ### From GitHub ```r install.packages('remotes') # do once remotes::install_github("rstudio/rmarkdown") # usual format is username/packagename ``` ] .pull-right[ > GitHub often hosts development version of packages published on CRAN or Bioconductor > Both CRAN and Bioconductor have stringent checks to make sure packages can run properly, with no obvious program flaws. There are typically no guarantees about analytic or theoretical correctness, but most packages have been crowd-validated and there are several reliable developer groups including RStudio ] --- ## Using packages You have to first "activate" the package in your current working session using the `library` function. .pull-left[ ```r ggplot(beaches, aes(temperature, rainfall)) + geom_point() ``` ``` #> Error in ggplot(beaches, aes(temperature, rainfall)): could not find function "ggplot" ``` The first thing you look for in this error message is which package isn't loaded. Then either load it, or install it, as the case may be<p> Recall, you have to install packages once .fatinline[_per computer_], but load packages once .heatinline[_per session_] ] -- .pull-right[ ```r library(ggplot2) # or library(tidyverse) ggplot(beaches, aes(temperature, rainfall)) + geom_point() ``` 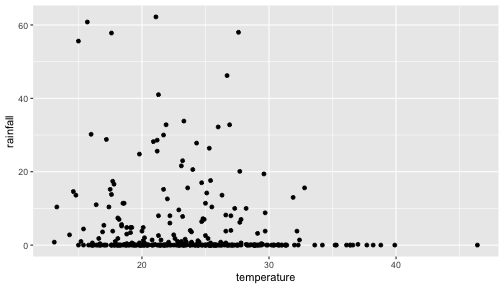<!-- --> ] --- class: center, middle # Tidying data using the tidyverse --- ## What is the ["Tidyverse"](http://www.tidyverse.org)? <div style="display:flex;align-items:center;font-size:28pt;font-family:'Roboto Slab',serif;width:100%;height:300px;background-color:wheat;text-align:center; border: 1px solid red; position: relative;"> The tidyverse is an opinionated collection of R packages designed for data science. All packages share an underlying design philosophy, grammar, and data structures </div> --- ## What is the "Tidyverse"? A set of R packages that: <ul> <li> help make data more computer-friendly <li>while making your code more human-friendly </ul> - Most of these packages are (co-)written by Dr. Hadley Wickham, who has rockstar status in the R world - They are supported by the company RStudio ---- The [tidyverse.org](https://www.tidyverse.org) site and the [R4DS book](https://r4ds.had.co.nz) are the definitive sources for tidyverse information.<p> The packages are united in a common philosophy of how data analysis should be done. --- class:middle, center # Tidying data --- ## Tidy data <div style="display:flex;align-items:center;font-size:30pt;font-family:'Roboto Slab';width:100%;height:300px;background-color:wheat;text-align:center; padding-left:30px;border: 1px solid red; position: relative;"> Tidy datasets are all alike, <br/> but every messy data is messy in its own way </div> --- ## Tidy data Tidy data is a **computer-friendly** format based on the following characteristics: - Each row is one observation - Each column is one variable - Each set of observational unit forms a table All other forms of data can be considered **messy data**. --- ## Let us count the ways There are many ways data can be messy. An incomplete list.... + Column headers are values, not variables + Multiple variables are stored in a single column + Variables are stored in both rows and columns + Multiple types of observational units are saved in the same table + A single observational unit is stored in multiple tables --- ## Ways to have messy (i.e. not tidy) data 1. Column headers contain values Country | < $10K | $10-20K | $20-50K | $50-100K | > $100K ----------|-------------|------------|-----------|-------------|--------- India | 40 | 25 | 25 | 9 | 1 USA | 20 | 20 | 20 | 30 | 10 --- ## Ways to have messy (i.e. not tidy) data Column headers contain values Country | Income | Percentage ----------|-----------|------------ India | < $10K | 40 USA | < $10K | 20 This is a case of reshaping or melting --- ## Ways to have messy (i.e. not tidy) data Multiple variables in one column Country | Year | M_0-14 | F_0-14 | M_ 15-60 | F_15-60 | M_60+ | F_60+ ---------|--------|---------|---------|-----------|----------|--------|------- UK | 2010 | | | | | | UK | 2011 | | | | | | <p> Separating columns into different variables Country | Year | Gender | Age | Count ---------|--------|---------|--------|------- --- ## Tidying data The typical steps are + Transforming data from wide to tall (`pivot_longer`) and from tall to wide (`pivot_wider`) + Separating columns into different columns (`separate`) + Putting columns together into new variables (`unite`) ---- >The functions `pivot_longer` and `pivot_wider` supercede the older functions `gather` and `spread`, which I have used in previous iterations of this class. However, if you are familiar with `gather` and `spread`, they aren't gone and can still be used in the current **tidyr** package. --- class: middle, center # Cleaning data --- ## Some actions on data + Creating new variables (`mutate`) + Choose some columns (`select`) + Selecting rows based on some criteria (`filter`) + Sort data based on some variables (`arrange`) + Reduce multiple values to a single summary (`summarize`/`summarise`) + Change the order of rows (`arrange`) --- ## Example data ```r head(mtcars, 3) ``` ``` #> mpg cyl disp hp drat wt qsec vs am gear carb #> Mazda RX4 21.0 6 160 110 3.90 2.620 16.46 0 1 4 4 #> Mazda RX4 Wag 21.0 6 160 110 3.90 2.875 17.02 0 1 4 4 #> Datsun 710 22.8 4 108 93 3.85 2.320 18.61 1 1 4 1 ``` - Car names are in an attribute of the data.frame called `rownames`. So it's not in a column - We might want to convert fuel economy to metric - We might just want to look at the relationship between displacement and fuel economy based on number of cylinders ---- The function `tibble::rownames_to_column` makes short work of the first point. <p> Row names are an older construct to give labels to each row. They are strongly discouraged outside of indices since using them as data created problems. --- ## Example data ([link](https://dl.dropboxusercontent.com/s/pqavhcckshqxtjm/brca.csv)) ```r link <- 'https://dl.dropboxusercontent.com/s/pqavhcckshqxtjm/brca.csv' brca_data <- rio::import(link) ``` 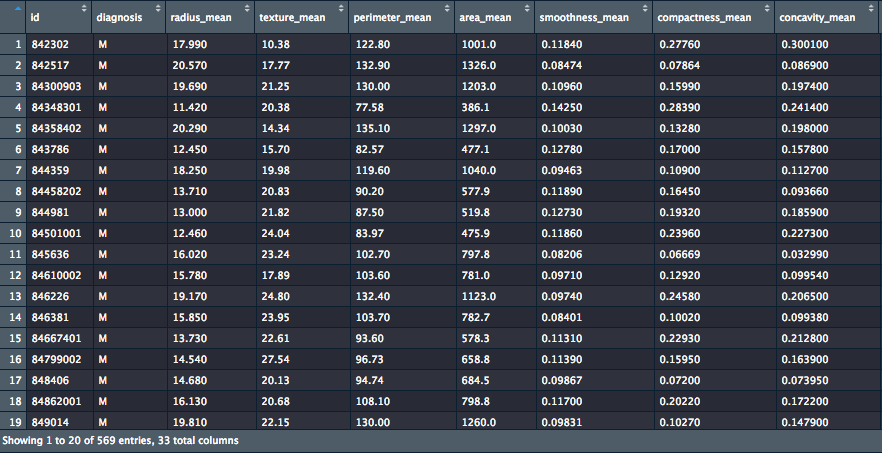 --- ## The `tidyverse` package The `tidyverse` package is a meta-package that installs a set of packages that are useful for data cleaning, data tidying and data munging (manipulating data to get a computationally "attractive" dataset) --- ## The `tidyverse` package ```r # install.packages('tidyverse') library(tidyverse) ``` #### Core `tidyverse` packages <table> <thead> <tr> <th style="text-align:left;"> Package </th> <th style="text-align:left;"> Description </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> ggplot2 </td> <td style="text-align:left;"> Data visualization </td> </tr> <tr> <td style="text-align:left;"> tibble </td> <td style="text-align:left;"> data.frame on steroids </td> </tr> <tr> <td style="text-align:left;"> tidyr </td> <td style="text-align:left;"> Data tidying (today) </td> </tr> <tr> <td style="text-align:left;"> readr </td> <td style="text-align:left;"> Reading text files (CSV) </td> </tr> <tr> <td style="text-align:left;"> purrr </td> <td style="text-align:left;"> Applying functions to data iteratively </td> </tr> <tr> <td style="text-align:left;"> dplyr </td> <td style="text-align:left;"> Data cleaning and munging (today) </td> </tr> <tr> <td style="text-align:left;"> stringr </td> <td style="text-align:left;"> String (character) manipulation </td> </tr> <tr> <td style="text-align:left;"> forcats </td> <td style="text-align:left;"> Manipulating categorical variables </td> </tr> </tbody> </table> --- ## Additional `tidyverse` packages <table> <thead> <tr> <th style="text-align:left;"> Package </th> <th style="text-align:left;"> Description </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> readxl </td> <td style="text-align:left;"> Read Excel files </td> </tr> <tr> <td style="text-align:left;"> haven </td> <td style="text-align:left;"> Read SAS, SPSS, Stata files </td> </tr> <tr> <td style="text-align:left;"> lubridate </td> <td style="text-align:left;"> Deal with dates and times </td> </tr> <tr> <td style="text-align:left;"> magrittr </td> <td style="text-align:left;"> Provides the pipe operator %>% </td> </tr> <tr> <td style="text-align:left;"> glue </td> <td style="text-align:left;"> Makes pasting text and data easier </td> </tr> </tbody> </table> ### Additional useful packages <table> <thead> <tr> <th style="text-align:left;"> Package </th> <th style="text-align:left;"> Description </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> broom </td> <td style="text-align:left;"> Turns the results of models or analysis into tidy datasets </td> </tr> <tr> <td style="text-align:left;"> fs </td> <td style="text-align:left;"> Allows directory and file manipulation in OS-agnostic manner </td> </tr> <tr> <td style="text-align:left;"> here </td> <td style="text-align:left;"> Allows robust specification of directory structure in a Project </td> </tr> </tbody> </table> --- class: middle, center # Pipes --- ## Pipes Pipes (denoted `%>%`, spoken as "then") are to analytic pipelines as `+` is to `ggplot` layers ```r mpg1 <- mpg %>% mutate(id=1:n()) %>% select(id, year, trans, cty, hwy) mpg_metric <- mpg1 %>% mutate(across(c(cty, hwy), function(x) {x * 1.6/3.8})) ``` .pull-left[ Original data <table> <thead> <tr> <th style="text-align:right;"> id </th> <th style="text-align:right;"> year </th> <th style="text-align:left;"> trans </th> <th style="text-align:right;"> cty </th> <th style="text-align:right;"> hwy </th> </tr> </thead> <tbody> <tr> <td style="text-align:right;"> 1 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> auto(l5) </td> <td style="text-align:right;"> 18 </td> <td style="text-align:right;"> 29 </td> </tr> <tr> <td style="text-align:right;"> 2 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> manual(m5) </td> <td style="text-align:right;"> 21 </td> <td style="text-align:right;"> 29 </td> </tr> <tr> <td style="text-align:right;"> 3 </td> <td style="text-align:right;"> 2008 </td> <td style="text-align:left;"> manual(m6) </td> <td style="text-align:right;"> 20 </td> <td style="text-align:right;"> 31 </td> </tr> <tr> <td style="text-align:right;"> 4 </td> <td style="text-align:right;"> 2008 </td> <td style="text-align:left;"> auto(av) </td> <td style="text-align:right;"> 21 </td> <td style="text-align:right;"> 30 </td> </tr> <tr> <td style="text-align:right;"> 5 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> auto(l5) </td> <td style="text-align:right;"> 16 </td> <td style="text-align:right;"> 26 </td> </tr> </tbody> </table> ] .pull-right[ Transformed data <table> <thead> <tr> <th style="text-align:right;"> id </th> <th style="text-align:right;"> year </th> <th style="text-align:left;"> trans </th> <th style="text-align:right;"> cty </th> <th style="text-align:right;"> hwy </th> </tr> </thead> <tbody> <tr> <td style="text-align:right;"> 1 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> auto(l5) </td> <td style="text-align:right;"> 7.578947 </td> <td style="text-align:right;"> 12.21053 </td> </tr> <tr> <td style="text-align:right;"> 2 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> manual(m5) </td> <td style="text-align:right;"> 8.842105 </td> <td style="text-align:right;"> 12.21053 </td> </tr> <tr> <td style="text-align:right;"> 3 </td> <td style="text-align:right;"> 2008 </td> <td style="text-align:left;"> manual(m6) </td> <td style="text-align:right;"> 8.421053 </td> <td style="text-align:right;"> 13.05263 </td> </tr> <tr> <td style="text-align:right;"> 4 </td> <td style="text-align:right;"> 2008 </td> <td style="text-align:left;"> auto(av) </td> <td style="text-align:right;"> 8.842105 </td> <td style="text-align:right;"> 12.63158 </td> </tr> <tr> <td style="text-align:right;"> 5 </td> <td style="text-align:right;"> 1999 </td> <td style="text-align:left;"> auto(l5) </td> <td style="text-align:right;"> 6.736842 </td> <td style="text-align:right;"> 10.94737 </td> </tr> </tbody> </table> ] Note I'm assigning a name to the transformed data. Otherwise it'll be lost --- ## Verbs to use in pipes The verbs in `tidyverse` are specially useful in pipes <table> <thead> <tr> <th style="text-align:left;"> Verb </th> <th style="text-align:left;"> Functionality </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> mutate </td> <td style="text-align:left;"> Transform a column with some function </td> </tr> <tr> <td style="text-align:left;"> select </td> <td style="text-align:left;"> Select some columns in the data </td> </tr> <tr> <td style="text-align:left;"> arrange </td> <td style="text-align:left;"> Order the data frame by values of a column(s) </td> </tr> <tr> <td style="text-align:left;"> filter </td> <td style="text-align:left;"> Keep only rows that meet some data criterion </td> </tr> <tr> <td style="text-align:left;"> group_by </td> <td style="text-align:left;"> Group by levels of a variable </td> </tr> <tr> <td style="text-align:left;"> pivot_longer </td> <td style="text-align:left;"> Transform a wide dataset to a long dataset </td> </tr> <tr> <td style="text-align:left;"> pivot_wider </td> <td style="text-align:left;"> Transform a long dataset to a wide dataset </td> </tr> <tr> <td style="text-align:left;"> separate </td> <td style="text-align:left;"> Separate one column into several columns </td> </tr> <tr> <td style="text-align:left;"> unite </td> <td style="text-align:left;"> Concatenate several columns into 1 column </td> </tr> </tbody> </table> Pipes almost always start with a data.frame/tibble object, and then "pipes" that data through different transformations (functions) At each `%>%`, the results of the previous step are used as input for the next step. --- ## A new verb: `across` **dplyr** version 1.0 introduced a new verb, `across`. It supercedes the older scoped verbs `*_at`, `*_if` and `*_all`, where `*` might be `mutate` or `summarise`. Use whichever makes sense to you. .pull-left[ ```r beaches %>% summarise_if(is.numeric, mean) ``` ``` #> year month day season rainfall temperature enterococci day_num #> 1 2015.494 6.377907 15.88953 2.52907 NA 23.57994 NA 178.7994 #> month_num #> 1 36.30814 ``` ```r beaches %>% group_by(season_name) %>% summarise_at(vars(rainfall, enterococci), median) ``` ``` #> # A tibble: 4 x 3 #> season_name rainfall enterococci #> * <chr> <dbl> <dbl> #> 1 Autumn 0 NA #> 2 Spring NA NA #> 3 Summer 0 NA #> 4 Winter 0 NA ``` ] .pull-right[ ```r beaches %>% summarise(across(where(is.numeric), mean)) ``` ``` #> year month day season rainfall temperature enterococci day_num #> 1 2015.494 6.377907 15.88953 2.52907 NA 23.57994 NA 178.7994 #> month_num #> 1 36.30814 ``` ```r beaches %>% group_by(season_name) %>% summarise(across(c(rainfall, enterococci), median)) ``` ``` #> # A tibble: 4 x 3 #> season_name rainfall enterococci #> * <chr> <dbl> <dbl> #> 1 Autumn 0 NA #> 2 Spring NA NA #> 3 Summer 0 NA #> 4 Winter 0 NA ``` ] --- ## `across` (Updated Spring 2021) The `across` function is now the more flexible standard way to apply most dplyr verbs across multiple columns, which can be selected the same way you would in `select`. In addition to what's on the previous slide, here are some more examples. .pull-left[ ```r summaries <- list( mean = ~mean(.x, na.rm=T), median = ~median(.x, na.rm=T) ) # both the ~ and .x are required beaches %>% summarise(across(.cols = where(is.numeric), .fns = summaries)) ``` ``` #> year_mean year_median month_mean month_median day_mean day_median season_mean #> 1 2015.494 2016 6.377907 6 15.88953 16 2.52907 #> season_median rainfall_mean rainfall_median temperature_mean #> 1 3 4.191228 0 23.57994 #> temperature_median enterococci_mean enterococci_median day_num_mean #> 1 23.3 34.59322 9.372727 178.7994 #> day_num_median month_num_mean month_num_median #> 1 177 36.30814 37 ``` ] .pull-right[ ```r beaches %>% summarise( across(where(is.numeric), ~mean(.x, na.rm=T), .names='mean_{.col}'), across(where(is.numeric), ~median(.x, na.rm=T), .anems='median_{.col}')) ``` ``` #> mean_year mean_month mean_day mean_season mean_rainfall mean_temperature #> 1 2015.494 6.377907 15.88953 2.52907 4.191228 23.57994 #> mean_enterococci mean_day_num mean_month_num year month day season rainfall #> 1 34.59322 178.7994 36.30814 2016 6 16 3 0 #> temperature enterococci day_num month_num #> 1 23.3 9.372727 177 37 ``` This groups the columns by function rather than by variable ] --- ## `across` (Updated Spring 2021) .pull-left[ ```r beaches %>% filter(across(everything(), ~!is.na(.x))) %>% head() # rows with no missing values ``` ``` #> date year month day season rainfall temperature enterococci day_num #> 1 2013-01-02 2013 1 2 1 0.0 23.4 6.7 2 #> 2 2013-01-06 2013 1 6 1 0.0 30.3 2.0 6 #> 3 2013-01-12 2013 1 12 1 0.0 31.4 69.1 12 #> 4 2013-01-18 2013 1 18 1 0.0 46.4 9.0 18 #> 5 2013-01-24 2013 1 24 1 0.0 27.5 33.9 24 #> 6 2013-01-30 2013 1 30 1 0.6 26.6 26.5 30 #> month_num month_name season_name #> 1 1 January Summer #> 2 1 January Summer #> 3 1 January Summer #> 4 1 January Summer #> 5 1 January Summer #> 6 1 January Summer ``` ] .pull-right[ #### For more examples see https://dplyr.tidyverse.org/articles/colwise.html ] --- class: middle, center # Modeling and the broom package --- ## Representing model relationships In R, there is a particularly convenient way to express models, where you have - one dependent variable - one or more independent variables, with possible transformations and interactions ``` y ~ x1 + x2 + x1:x2 + I(x3^2) + x4*x5 ``` --- ## Representing model relationships ``` y ~ x1 + x2 + x1:x2 + I(x3^2) + x4*x5 ``` `y` depends on ... - `x1` and `x2` linearly - the interaction of `x1` and `x2` (represented as `x1:x2`) - the square of `x3` (the `I()` notation ensures that the `^` symbol is interpreted correctly) - `x4`, `x5` and their interaction (same as `x4 + x5 + x4:x5`) --- # Representing model relationships ``` y ~ x1 + x2 + x1:x2 + I(x3^2) + x4*x5 ``` This interpretation holds for the vast majority of statistical models in R - For decision trees and random forests and neural networks, don't add interactions or transformations, since the model will try to figure those out on their own --- # Our first model ```r library(survival) myLinearModel <- lm(chol ~ bili, data = pbc) ``` Note that everything in R is an **object**, so you can store a model in a variable name. This statement runs the model and stored the fitted model in `myLinearModel` R does not interpret the model, evaluate the adequacy or appropriateness of the model, or comment on whether looking at the relationship between cholesterol and bilirubin makes any kind of sense. --- # Our first model ```r myLinearModel ``` ``` #> #> Call: #> lm(formula = chol ~ bili, data = pbc) #> #> Coefficients: #> (Intercept) bili #> 303.20 20.24 ``` > Not very informative, is it? --- # Our first model ```r summary(myLinearModel) ``` ``` #> #> Call: #> lm(formula = chol ~ bili, data = pbc) #> #> Residuals: #> Min 1Q Median 3Q Max #> -565.39 -89.90 -35.36 44.92 1285.33 #> #> Coefficients: #> Estimate Std. Error t value Pr(>|t|) #> (Intercept) 303.204 15.601 19.435 < 2e-16 *** #> bili 20.240 2.785 7.267 3.63e-12 *** #> --- #> Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1 #> #> Residual standard error: 213.2 on 282 degrees of freedom #> (134 observations deleted due to missingness) #> Multiple R-squared: 0.1577, Adjusted R-squared: 0.1547 #> F-statistic: 52.8 on 1 and 282 DF, p-value: 3.628e-12 ``` > A little better ??? Talk about the different metrics in this slide --- # Our first model ```r broom::tidy(myLinearModel) ``` ``` #> # A tibble: 2 x 5 #> term estimate std.error statistic p.value #> <chr> <dbl> <dbl> <dbl> <dbl> #> 1 (Intercept) 303. 15.6 19.4 5.65e-54 #> 2 bili 20.2 2.79 7.27 3.63e-12 ``` ```r broom::glance(myLinearModel) ``` ``` #> # A tibble: 1 x 12 #> r.squared adj.r.squared sigma statistic p.value df logLik AIC BIC #> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> #> 1 0.158 0.155 213. 52.8 3.63e-12 1 -1925. 3856. 3867. #> # … with 3 more variables: deviance <dbl>, df.residual <int>, nobs <int> ``` --- ## Displaying model results Let's start with this model: ```r myModel <- lm(log10(enterococci) ~ rainfall + temperature + season_name + factor(year), data = beaches) broom::tidy(myModel) ``` ``` #> # A tibble: 11 x 5 #> term estimate std.error statistic p.value #> <chr> <dbl> <dbl> <dbl> <dbl> #> 1 (Intercept) 1.23 0.208 5.92 8.39e- 9 #> 2 rainfall 0.0284 0.00296 9.58 2.72e-19 #> 3 temperature -0.00830 0.00769 -1.08 2.81e- 1 #> 4 season_nameSpring -0.325 0.0840 -3.87 1.31e- 4 #> 5 season_nameSummer 0.0862 0.0909 0.948 3.44e- 1 #> 6 season_nameWinter -0.332 0.0889 -3.74 2.22e- 4 #> 7 factor(year)2014 0.0498 0.102 0.486 6.27e- 1 #> 8 factor(year)2015 0.0807 0.100 0.804 4.22e- 1 #> 9 factor(year)2016 0.0815 0.0975 0.836 4.04e- 1 #> 10 factor(year)2017 -0.0676 0.0972 -0.696 4.87e- 1 #> 11 factor(year)2018 0.0440 0.107 0.411 6.81e- 1 ``` --- # Displaying model results Let's start with this model: ```r plt_data <- broom::tidy(myModel) plt_data <- plt_data %>% * filter(term != '(Intercept)') %>% mutate(term = str_replace(term, * 'season_name','')) plt_data ``` ``` #> # A tibble: 10 x 5 #> term estimate std.error statistic p.value #> <chr> <dbl> <dbl> <dbl> <dbl> #> 1 rainfall 0.0284 0.00296 9.58 2.72e-19 #> 2 temperature -0.00830 0.00769 -1.08 2.81e- 1 #> 3 Spring -0.325 0.0840 -3.87 1.31e- 4 #> 4 Summer 0.0862 0.0909 0.948 3.44e- 1 #> 5 Winter -0.332 0.0889 -3.74 2.22e- 4 #> 6 factor(year)2014 0.0498 0.102 0.486 6.27e- 1 #> 7 factor(year)2015 0.0807 0.100 0.804 4.22e- 1 #> 8 factor(year)2016 0.0815 0.0975 0.836 4.04e- 1 #> 9 factor(year)2017 -0.0676 0.0972 -0.696 4.87e- 1 #> 10 factor(year)2018 0.0440 0.107 0.411 6.81e- 1 ``` --- # Displaying model results Let's start with this model: ```r plt_data <- broom::tidy(myModel) plt_data <- plt_data %>% filter(term != '(Intercept)') %>% mutate(term = str_replace(term, 'season_name','')) %>% mutate(term = str_replace(term, * 'factor\\(year\\)','')) # Brackets are "escaped" using \\ plt_data ``` ``` #> # A tibble: 10 x 5 #> term estimate std.error statistic p.value #> <chr> <dbl> <dbl> <dbl> <dbl> #> 1 rainfall 0.0284 0.00296 9.58 2.72e-19 #> 2 temperature -0.00830 0.00769 -1.08 2.81e- 1 #> 3 Spring -0.325 0.0840 -3.87 1.31e- 4 #> 4 Summer 0.0862 0.0909 0.948 3.44e- 1 #> 5 Winter -0.332 0.0889 -3.74 2.22e- 4 #> 6 2014 0.0498 0.102 0.486 6.27e- 1 #> 7 2015 0.0807 0.100 0.804 4.22e- 1 #> 8 2016 0.0815 0.0975 0.836 4.04e- 1 #> 9 2017 -0.0676 0.0972 -0.696 4.87e- 1 #> 10 2018 0.0440 0.107 0.411 6.81e- 1 ``` --- # Displaying model results Let's start with this model: .pull-left[ ```r plt_data <- broom::tidy(myModel) plt_data %>% filter(term != '(Intercept)') %>% mutate(term = str_replace(term, 'season_name','')) %>% mutate(term = str_replace(term, 'factor\\(year\\)','')) %>% # Brackets need to be "escaped" using \\ ggplot(aes(x = term, y = estimate, ymin = estimate - 2 * std.error, ymax = estimate + 2 * std.error))+ geom_pointrange() ``` ] .pull-right[ 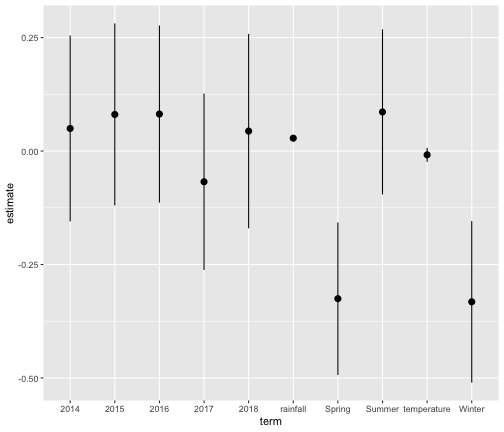<!-- --> ] --- # Displaying model results Let's start with this model: .pull-left[ ```r plt_data <- broom::tidy(myModel) plt_data %>% filter(term != '(Intercept)') %>% mutate(term = str_replace(term, 'season_name','')) %>% mutate(term = str_replace(term, 'factor\\(year\\)','')) %>% # Brackets need to be "escaped" using \\ ggplot(aes(x = term, y = estimate, ymin = estimate - 2 * std.error, ymax = estimate + 2 * std.error))+ geom_pointrange() + geom_hline(yintercept = 0, linetype=2) + theme_bw() + coord_flip() ``` ] .pull-right[ 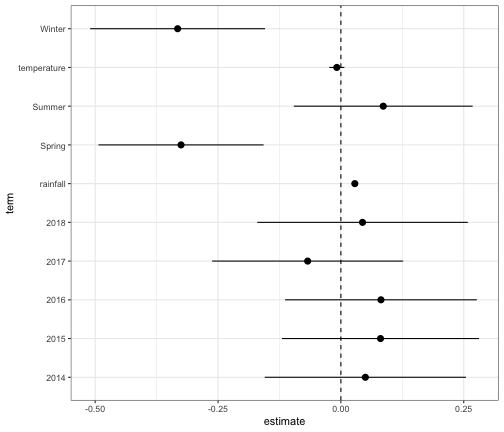<!-- --> ] --- # Displaying model results Let's start with this model: .pull-left[ ```r plt_data <- broom::tidy(myModel) plt_data %>% filter(term != '(Intercept)') %>% mutate(term = str_replace(term, 'season_name','')) %>% mutate(term = str_replace(term, 'factor\\(year\\)','')) %>% # Brackets need to be "escaped" using \\ ggplot(aes(x = term, y = estimate, ymin = estimate - 2 * std.error, ymax = estimate + 2 * std.error))+ geom_pointrange() + geom_hline(yintercept = 0, linetype=2) + theme_bw() + coord_flip() ggsave('results.png') # ggsave knows format from file ``` You can also save the graph from the RStudio Plots pane, but coding it using `ggsave` is more reproducible ] .pull-right[ 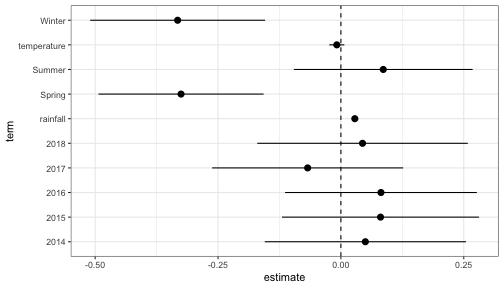<!-- --> If you need to get a high-definition TIFF on a Mac, your best bet is to save your graph as a PDF and then convert it using Acrobat or other scripts (ask me if interested). The TIFF printer in R only creates 72 DPI TIFF files ] --- ## Showing group differences ("Figure 1") The package `ggpubr`, which extends `ggplot2`, makes this very easy. It provides the function `stat_compare_means` --- ## Showing group differences ("Figure 1") .pull-left[ ```r library(ggpubr) *theme_viz <- function(){ * theme_bw() + * theme(axis.title = element_text(size=16), * axis.text = element_text(size=14), * text = element_text(size = 14)) *} ggplot( data=beaches, mapping= aes(x = season_name, y = log10(enterococci), color = season_name)) + geom_boxplot()+geom_jitter()+ labs(x = 'Season', y = expression(paste('log'['10'],'(enterococci)')), color='Season') + theme_viz() ``` ] .pull-right[ 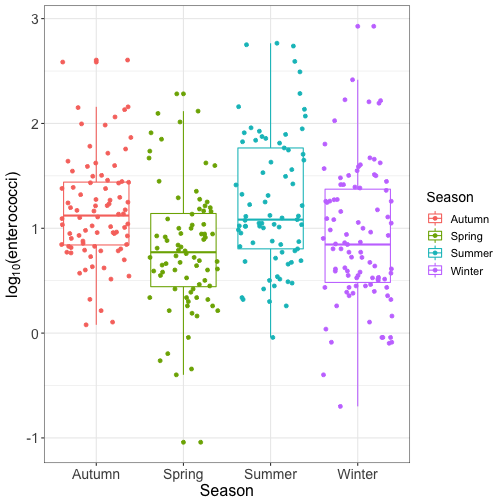<!-- --> ] --- ## Showing group differences ("Figure 1") .pull-left[ ```r library(ggpubr) plt <- ggplot( data=beaches, mapping= aes(x = season_name, y = log10(enterococci), color = season_name)) + geom_boxplot() + geom_jitter(width=0.1) + labs(x = 'Season', y = expression(paste('log'['10'],'(enterococci)')), color='Season')+ theme_viz() my_comparisons <- list(c('Autumn','Spring'), c('Spring','Summer'), c('Summer','Winter'), c('Spring','Winter')) *plt + stat_compare_means() ``` ] .pull-right[ 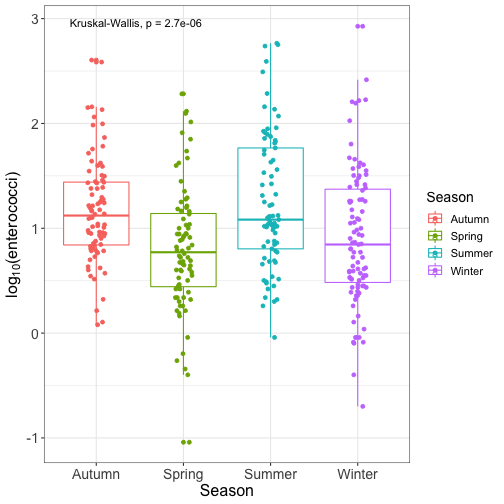<!-- --> ] --- ## Showing group differences ("Figure 1") .pull-left[ ```r library(ggpubr) plt <- ggplot( data=beaches, mapping= aes(x = season_name, y = log10(enterococci), color = season_name)) + geom_boxplot() + geom_jitter(width=0.1)+ labs(x = 'Season', y = expression(paste('log'['10'],'(enterococci)')), color='Season') + theme_viz() my_comparisons <- list(c('Autumn','Spring'), c('Spring','Summer'), c('Summer','Winter'), c('Spring','Winter')) plt + stat_compare_means() + * stat_compare_means(comparisons = my_comparisons) ``` ] .pull-right[ 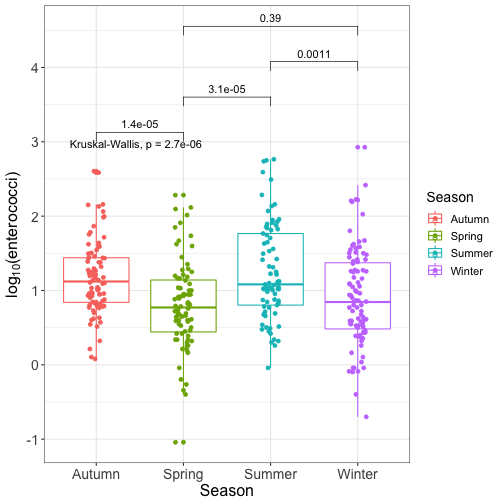<!-- --> ] --- ## Showing group differences ("Figure 1") .pull-left[ ```r library(ggpubr) plt <- ggplot( data=beaches, mapping= aes(x = season_name, y = log10(enterococci), color = season_name)) + geom_boxplot() + geom_jitter(width=0.1)+ labs(x = 'Season', y = expression(paste('log'['10'],'(enterococci)')), color='Season')+ theme_viz() my_comparisons <- list(c('Autumn','Spring'), c('Spring','Summer'), c('Summer','Winter'), c('Spring','Winter')) plt + stat_compare_means(label.y = 6) + stat_compare_means(comparisons = my_comparisons) ``` ] .pull-right[ 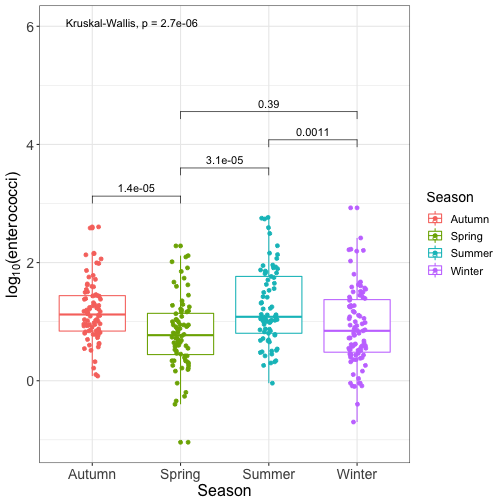<!-- --> ] --- ## Manipulating data for plotting We would like to get density plots of all the variables .pull-left[ ```r dat_spine <- rio::import('data/Dataset_spine.csv', check.names=T) head(dat_spine) ``` ``` #> pelvic_incidence pelvic_tilt lumbar_lordosis_angle sacral_slope pelvic_radius #> 1 63.02782 22.552586 39.60912 40.47523 98.67292 #> 2 39.05695 10.060991 25.01538 28.99596 114.40543 #> 3 68.83202 22.218482 50.09219 46.61354 105.98514 #> 4 69.29701 24.652878 44.31124 44.64413 101.86850 #> 5 49.71286 9.652075 28.31741 40.06078 108.16872 #> 6 40.25020 13.921907 25.12495 26.32829 130.32787 #> degree_spondylolisthesis pelvic_slope direct_tilt thoracic_slope #> 1 -0.254400 0.7445035 12.5661 14.5386 #> 2 4.564259 0.4151857 12.8874 17.5323 #> 3 -3.530317 0.4748892 26.8343 17.4861 #> 4 11.211523 0.3693453 23.5603 12.7074 #> 5 7.918501 0.5433605 35.4940 15.9546 #> 6 2.230652 0.7899929 29.3230 12.0036 #> cervical_tilt sacrum_angle scoliosis_slope class_attribute #> 1 15.30468 -28.658501 43.5123 Abnormal #> 2 16.78486 -25.530607 16.1102 Abnormal #> 3 16.65897 -29.031888 19.2221 Abnormal #> 4 11.42447 -30.470246 18.8329 Abnormal #> 5 8.87237 -16.378376 24.9171 Abnormal #> 6 10.40462 -1.512209 9.6548 Abnormal ``` ] .pull-right[ Facets only work by grouping on a variable. Here we have data in several columns ] --- ## Manipulating data for plotting We would like to get density plots of all the variables. .pull-left[ ```r dat_spine %>% tidyr::gather(variable, value, everything()) ``` ``` #> variable value #> 1 pelvic_incidence 63.0278175 #> 2 pelvic_incidence 39.05695098 #> 3 pelvic_incidence 68.83202098 #> 4 pelvic_incidence 69.29700807 #> 5 pelvic_incidence 49.71285934 #> 6 pelvic_incidence 40.25019968 #> 7 pelvic_incidence 53.43292815 #> 8 pelvic_incidence 45.36675362 #> 9 pelvic_incidence 43.79019026 #> 10 pelvic_incidence 36.68635286 #> 11 pelvic_incidence 49.70660953 #> 12 pelvic_incidence 31.23238734 #> 13 pelvic_incidence 48.91555137 #> 14 pelvic_incidence 53.5721702 #> 15 pelvic_incidence 57.30022656 #> 16 pelvic_incidence 44.31890674 #> 17 pelvic_incidence 63.83498162 #> 18 pelvic_incidence 31.27601184 #> 19 pelvic_incidence 38.69791243 #> 20 pelvic_incidence 41.72996308 #> 21 pelvic_incidence 43.92283983 #> 22 pelvic_incidence 54.91944259 #> 23 pelvic_incidence 63.07361096 #> 24 pelvic_incidence 45.54078988 #> 25 pelvic_incidence 36.12568347 #> 26 pelvic_incidence 54.12492019 #> 27 pelvic_incidence 26.14792141 #> 28 pelvic_incidence 43.58096394 #> 29 pelvic_incidence 44.5510115 #> 30 pelvic_incidence 66.87921138 #> 31 pelvic_incidence 50.81926781 #> 32 pelvic_incidence 46.39026008 #> 33 pelvic_incidence 44.93667457 #> 34 pelvic_incidence 38.66325708 #> 35 pelvic_incidence 59.59554032 #> 36 pelvic_incidence 31.48421834 #> 37 pelvic_incidence 32.09098679 #> 38 pelvic_incidence 35.70345781 #> 39 pelvic_incidence 55.84328595 #> 40 pelvic_incidence 52.41938511 #> 41 pelvic_incidence 35.49244617 #> 42 pelvic_incidence 46.44207842 #> 43 pelvic_incidence 53.85479842 #> 44 pelvic_incidence 66.28539377 #> 45 pelvic_incidence 56.03021778 #> 46 pelvic_incidence 50.91244034 #> 47 pelvic_incidence 48.332638 #> 48 pelvic_incidence 41.35250407 #> 49 pelvic_incidence 40.55735663 #> 50 pelvic_incidence 41.76773173 #> 51 pelvic_incidence 55.28585178 #> 52 pelvic_incidence 74.43359316 #> 53 pelvic_incidence 50.20966979 #> 54 pelvic_incidence 30.14993632 #> 55 pelvic_incidence 41.17167989 #> 56 pelvic_incidence 47.65772963 #> 57 pelvic_incidence 43.34960621 #> 58 pelvic_incidence 46.85578065 #> 59 pelvic_incidence 43.20318499 #> 60 pelvic_incidence 48.10923638 #> 61 pelvic_incidence 74.37767772 #> 62 pelvic_incidence 89.68056731 #> 63 pelvic_incidence 44.529051 #> 64 pelvic_incidence 77.69057712 #> 65 pelvic_incidence 76.1472121 #> 66 pelvic_incidence 83.93300857 #> 67 pelvic_incidence 78.49173027 #> 68 pelvic_incidence 75.64973136 #> 69 pelvic_incidence 72.07627839 #> 70 pelvic_incidence 58.59952852 #> 71 pelvic_incidence 72.56070163 #> 72 pelvic_incidence 86.90079431 #> 73 pelvic_incidence 84.97413208 #> 74 pelvic_incidence 55.512212 #> 75 pelvic_incidence 72.2223343 #> 76 pelvic_incidence 70.22145219 #> 77 pelvic_incidence 86.75360946 #> 78 pelvic_incidence 58.78254775 #> 79 pelvic_incidence 67.41253785 #> 80 pelvic_incidence 47.74467877 #> 81 pelvic_incidence 77.10657122 #> 82 pelvic_incidence 74.00554124 #> 83 pelvic_incidence 88.62390839 #> 84 pelvic_incidence 81.10410039 #> 85 pelvic_incidence 76.32600187 #> 86 pelvic_incidence 45.44374959 #> 87 pelvic_incidence 59.78526526 #> 88 pelvic_incidence 44.91414916 #> 89 pelvic_incidence 56.60577127 #> 90 pelvic_incidence 71.18681115 #> 91 pelvic_incidence 81.65603206 #> 92 pelvic_incidence 70.95272771 #> 93 pelvic_incidence 85.35231529 #> 94 pelvic_incidence 58.10193455 #> 95 pelvic_incidence 94.17482232 #> 96 pelvic_incidence 57.52235608 #> 97 pelvic_incidence 96.65731511 #> 98 pelvic_incidence 74.72074622 #> 99 pelvic_incidence 77.65511874 #> 100 pelvic_incidence 58.52162283 #> 101 pelvic_incidence 84.5856071 #> 102 pelvic_incidence 79.93857026 #> 103 pelvic_incidence 70.39930842 #> 104 pelvic_incidence 49.78212054 #> 105 pelvic_incidence 77.40933294 #> 106 pelvic_incidence 65.00796426 #> 107 pelvic_incidence 65.01377322 #> 108 pelvic_incidence 78.42595126 #> 109 pelvic_incidence 63.17298709 #> 110 pelvic_incidence 68.61300092 #> 111 pelvic_incidence 63.90063261 #> 112 pelvic_incidence 84.99895554 #> 113 pelvic_incidence 42.02138603 #> 114 pelvic_incidence 69.75666532 #> 115 pelvic_incidence 80.98807441 #> 116 pelvic_incidence 129.8340406 #> 117 pelvic_incidence 70.48410444 #> 118 pelvic_incidence 86.04127982 #> 119 pelvic_incidence 65.53600255 #> 120 pelvic_incidence 60.7538935 #> 121 pelvic_incidence 54.74177518 #> 122 pelvic_incidence 83.87994081 #> 123 pelvic_incidence 80.07491418 #> 124 pelvic_incidence 65.66534698 #> 125 pelvic_incidence 74.71722805 #> 126 pelvic_incidence 48.06062649 #> 127 pelvic_incidence 70.67689818 #> 128 pelvic_incidence 80.43342782 #> 129 pelvic_incidence 90.51396072 #> 130 pelvic_incidence 77.23689752 #> 131 pelvic_incidence 50.06678595 #> 132 pelvic_incidence 69.78100617 #> 133 pelvic_incidence 69.62628302 #> 134 pelvic_incidence 81.75441933 #> 135 pelvic_incidence 52.20469309 #> 136 pelvic_incidence 77.12134424 #> 137 pelvic_incidence 88.0244989 #> 138 pelvic_incidence 83.39660609 #> 139 pelvic_incidence 72.05403412 #> 140 pelvic_incidence 85.09550254 #> 141 pelvic_incidence 69.56348614 #> 142 pelvic_incidence 89.5049473 #> 143 pelvic_incidence 85.29017283 #> 144 pelvic_incidence 60.62621697 #> 145 pelvic_incidence 60.04417717 #> 146 pelvic_incidence 85.64378664 #> 147 pelvic_incidence 85.58171024 #> 148 pelvic_incidence 55.08076562 #> 149 pelvic_incidence 65.75567895 #> 150 pelvic_incidence 79.24967118 #> 151 pelvic_incidence 81.11260488 #> 152 pelvic_incidence 48.0306238 #> 153 pelvic_incidence 63.40448058 #> 154 pelvic_incidence 57.28694488 #> 155 pelvic_incidence 41.18776972 #> 156 pelvic_incidence 66.80479632 #> 157 pelvic_incidence 79.4769781 #> 158 pelvic_incidence 44.21646446 #> 159 pelvic_incidence 57.03509717 #> 160 pelvic_incidence 64.27481758 #> 161 pelvic_incidence 92.02630795 #> 162 pelvic_incidence 67.26314926 #> 163 pelvic_incidence 118.1446548 #> 164 pelvic_incidence 115.9232606 #> 165 pelvic_incidence 53.94165809 #> 166 pelvic_incidence 83.7031774 #> 167 pelvic_incidence 56.99140382 #> 168 pelvic_incidence 72.34359434 #> 169 pelvic_incidence 95.38259648 #> 170 pelvic_incidence 44.25347645 #> 171 pelvic_incidence 64.80954139 #> 172 pelvic_incidence 78.40125389 #> 173 pelvic_incidence 56.66829282 #> 174 pelvic_incidence 50.82502875 #> 175 pelvic_incidence 61.41173702 #> 176 pelvic_incidence 56.56382381 #> 177 pelvic_incidence 67.02766447 #> 178 pelvic_incidence 80.81777144 #> 179 pelvic_incidence 80.65431956 #> 180 pelvic_incidence 68.72190982 #> 181 pelvic_incidence 37.90391014 #> 182 pelvic_incidence 64.62400798 #> 183 pelvic_incidence 75.43774787 #> 184 pelvic_incidence 71.00194076 #> 185 pelvic_incidence 81.05661087 #> 186 pelvic_incidence 91.46874146 #> 187 pelvic_incidence 81.08232025 #> 188 pelvic_incidence 60.419932 #> 189 pelvic_incidence 85.68094951 #> 190 pelvic_incidence 82.4065243 #> 191 pelvic_incidence 43.7182623 #> 192 pelvic_incidence 86.472905 #> 193 pelvic_incidence 74.46908181 #> 194 pelvic_incidence 70.25043628 #> 195 pelvic_incidence 72.64385013 #> 196 pelvic_incidence 71.24176388 #> 197 pelvic_incidence 63.7723908 #> 198 pelvic_incidence 58.82837872 #> 199 pelvic_incidence 74.85448008 #> 200 pelvic_incidence 75.29847847 #> 201 pelvic_incidence 63.36433898 #> 202 pelvic_incidence 67.51305267 #> 203 pelvic_incidence 76.31402766 #> 204 pelvic_incidence 73.63596236 #> 205 pelvic_incidence 56.53505139 #> 206 pelvic_incidence 80.11157156 #> 207 pelvic_incidence 95.48022873 #> 208 pelvic_incidence 74.09473084 #> 209 pelvic_incidence 87.67908663 #> 210 pelvic_incidence 48.25991962 #> 211 pelvic_incidence 38.50527283 #> 212 pelvic_incidence 54.92085752 #> 213 pelvic_incidence 44.36249017 #> 214 pelvic_incidence 48.3189305 #> 215 pelvic_incidence 45.70178875 #> 216 pelvic_incidence 30.74193812 #> 217 pelvic_incidence 50.91310144 #> 218 pelvic_incidence 38.12658854 #> 219 pelvic_incidence 51.62467183 #> 220 pelvic_incidence 64.31186727 #> 221 pelvic_incidence 44.48927476 #> 222 pelvic_incidence 54.9509702 #> 223 pelvic_incidence 56.10377352 #> 224 pelvic_incidence 69.3988184 #> 225 pelvic_incidence 89.83467631 #> 226 pelvic_incidence 59.72614016 #> 227 pelvic_incidence 63.95952166 #> 228 pelvic_incidence 61.54059876 #> 229 pelvic_incidence 38.04655072 #> 230 pelvic_incidence 43.43645061 #> 231 pelvic_incidence 65.61180231 #> 232 pelvic_incidence 53.91105429 #> 233 pelvic_incidence 43.11795103 #> 234 pelvic_incidence 40.6832291 #> 235 pelvic_incidence 37.7319919 #> 236 pelvic_incidence 63.92947003 #> 237 pelvic_incidence 61.82162717 #> 238 pelvic_incidence 62.14080535 #> 239 pelvic_incidence 69.00491277 #> 240 pelvic_incidence 56.44702568 #> 241 pelvic_incidence 41.6469159 #> 242 pelvic_incidence 51.52935759 #> 243 pelvic_incidence 39.08726449 #> 244 pelvic_incidence 34.64992241 #> 245 pelvic_incidence 63.02630005 #> 246 pelvic_incidence 47.80555887 #> 247 pelvic_incidence 46.63786363 #> 248 pelvic_incidence 49.82813487 #> 249 pelvic_incidence 47.31964755 #> 250 pelvic_incidence 50.75329025 #> 251 pelvic_incidence 36.15782981 #> 252 pelvic_incidence 40.74699612 #> 253 pelvic_incidence 42.91804052 #> 254 pelvic_incidence 63.79242525 #> 255 pelvic_incidence 72.95564397 #> 256 pelvic_incidence 67.53818154 #> 257 pelvic_incidence 54.75251965 #> 258 pelvic_incidence 50.16007802 #> 259 pelvic_incidence 40.34929637 #> 260 pelvic_incidence 63.61919213 #> 261 pelvic_incidence 54.14240778 #> 262 pelvic_incidence 74.97602148 #> 263 pelvic_incidence 42.51727249 #> 264 pelvic_incidence 33.78884314 #> 265 pelvic_incidence 54.5036853 #> 266 pelvic_incidence 48.17074627 #> 267 pelvic_incidence 46.37408781 #> 268 pelvic_incidence 52.86221391 #> 269 pelvic_incidence 57.1458515 #> 270 pelvic_incidence 37.14014978 #> 271 pelvic_incidence 51.31177106 #> 272 pelvic_incidence 42.51561014 #> 273 pelvic_incidence 39.35870531 #> 274 pelvic_incidence 35.8775708 #> 275 pelvic_incidence 43.1919153 #> 276 pelvic_incidence 67.28971201 #> 277 pelvic_incidence 51.32546366 #> 278 pelvic_incidence 65.7563482 #> 279 pelvic_incidence 40.41336566 #> 280 pelvic_incidence 48.80190855 #> 281 pelvic_incidence 50.08615264 #> 282 pelvic_incidence 64.26150724 #> 283 pelvic_incidence 53.68337998 #> 284 pelvic_incidence 48.99595771 #> 285 pelvic_incidence 59.16761171 #> 286 pelvic_incidence 67.80469442 #> 287 pelvic_incidence 61.73487533 #> 288 pelvic_incidence 33.04168754 #> 289 pelvic_incidence 74.56501543 #> 290 pelvic_incidence 44.43070103 #> 291 pelvic_incidence 36.42248549 #> 292 pelvic_incidence 51.07983294 #> 293 pelvic_incidence 34.75673809 #> 294 pelvic_incidence 48.90290434 #> 295 pelvic_incidence 46.23639915 #> 296 pelvic_incidence 46.42636614 #> 297 pelvic_incidence 39.65690201 #> 298 pelvic_incidence 45.57548229 #> 299 pelvic_incidence 66.50717865 #> 300 pelvic_incidence 82.90535054 #> 301 pelvic_incidence 50.67667667 #> 302 pelvic_incidence 89.01487529 #> 303 pelvic_incidence 54.60031622 #> 304 pelvic_incidence 34.38229939 #> 305 pelvic_incidence 45.07545026 #> 306 pelvic_incidence 47.90356517 #> 307 pelvic_incidence 53.93674778 #> 308 pelvic_incidence 61.44659663 #> 309 pelvic_incidence 45.25279209 #> 310 pelvic_incidence 33.84164075 #> 311 pelvic_tilt 22.55258597 #> 312 pelvic_tilt 10.06099147 #> 313 pelvic_tilt 22.21848205 #> 314 pelvic_tilt 24.65287791 #> 315 pelvic_tilt 9.652074879 #> 316 pelvic_tilt 13.92190658 #> 317 pelvic_tilt 15.86433612 #> 318 pelvic_tilt 10.75561143 #> 319 pelvic_tilt 13.5337531 #> 320 pelvic_tilt 5.010884121 #> 321 pelvic_tilt 13.04097405 #> 322 pelvic_tilt 17.71581923 #> 323 pelvic_tilt 19.96455616 #> 324 pelvic_tilt 20.46082824 #> 325 pelvic_tilt 24.1888846 #> 326 pelvic_tilt 12.53799164 #> 327 pelvic_tilt 20.36250706 #> 328 pelvic_tilt 3.14466948 #> 329 pelvic_tilt 13.44474904 #> 330 pelvic_tilt 12.25407408 #> 331 pelvic_tilt 14.17795853 #> 332 pelvic_tilt 21.06233245 #> 333 pelvic_tilt 24.41380271 #> 334 pelvic_tilt 13.06959759 #> 335 pelvic_tilt 22.75875277 #> 336 pelvic_tilt 26.65048856 #> 337 pelvic_tilt 10.75945357 #> 338 pelvic_tilt 16.5088837 #> 339 pelvic_tilt 21.93114655 #> 340 pelvic_tilt 24.89199889 #> 341 pelvic_tilt 15.40221253 #> 342 pelvic_tilt 11.07904664 #> 343 pelvic_tilt 17.44383762 #> 344 pelvic_tilt 12.98644139 #> 345 pelvic_tilt 31.99824445 #> 346 pelvic_tilt 7.82622134 #> 347 pelvic_tilt 6.989378081 #> 348 pelvic_tilt 19.44325311 #> 349 pelvic_tilt 28.84744756 #> 350 pelvic_tilt 19.01156052 #> 351 pelvic_tilt 11.7016723 #> 352 pelvic_tilt 8.39503589 #> 353 pelvic_tilt 19.23064334 #> 354 pelvic_tilt 26.32784484 #> 355 pelvic_tilt 16.2979149 #> 356 pelvic_tilt 23.01516931 #> 357 pelvic_tilt 22.22778399 #> 358 pelvic_tilt 16.57736351 #> 359 pelvic_tilt 17.97778407 #> 360 pelvic_tilt 17.89940172 #> 361 pelvic_tilt 20.44011836 #> 362 pelvic_tilt 41.55733141 #> 363 pelvic_tilt 29.76012218 #> 364 pelvic_tilt 11.91744524 #> 365 pelvic_tilt 17.32120599 #> 366 pelvic_tilt 13.27738491 #> 367 pelvic_tilt 7.467468964 #> 368 pelvic_tilt 15.35151393 #> 369 pelvic_tilt 19.66314572 #> 370 pelvic_tilt 14.93072472 #> 371 pelvic_tilt 32.05310438 #> 372 pelvic_tilt 32.70443487 #> 373 pelvic_tilt 9.433234213 #> 374 pelvic_tilt 21.38064464 #> 375 pelvic_tilt 21.93618556 #> 376 pelvic_tilt 41.28630543 #> 377 pelvic_tilt 22.1817978 #> 378 pelvic_tilt 19.33979889 #> 379 pelvic_tilt 18.94617604 #> 380 pelvic_tilt -0.261499046 #> 381 pelvic_tilt 17.38519079 #> 382 pelvic_tilt 32.9281677 #> 383 pelvic_tilt 33.02117462 #> 384 pelvic_tilt 20.09515673 #> 385 pelvic_tilt 23.07771056 #> 386 pelvic_tilt 39.82272448 #> 387 pelvic_tilt 36.04301632 #> 388 pelvic_tilt 7.667044186 #> 389 pelvic_tilt 17.44279712 #> 390 pelvic_tilt 12.08935067 #> 391 pelvic_tilt 30.46999418 #> 392 pelvic_tilt 21.12240192 #> 393 pelvic_tilt 29.08945331 #> 394 pelvic_tilt 24.79416792 #> 395 pelvic_tilt 42.39620445 #> 396 pelvic_tilt 9.906071798 #> 397 pelvic_tilt 17.87932332 #> 398 pelvic_tilt 10.21899563 #> 399 pelvic_tilt 16.80020017 #> 400 pelvic_tilt 23.89620111 #> 401 pelvic_tilt 28.74886935 #> 402 pelvic_tilt 20.15993121 #> 403 pelvic_tilt 15.84491006 #> 404 pelvic_tilt 14.83763914 #> 405 pelvic_tilt 15.38076983 #> 406 pelvic_tilt 33.64707522 #> 407 pelvic_tilt 19.46158117 #> 408 pelvic_tilt 19.75694203 #> 409 pelvic_tilt 22.4329501 #> 410 pelvic_tilt 13.92228609 #> 411 pelvic_tilt 30.36168482 #> 412 pelvic_tilt 18.7740711 #> 413 pelvic_tilt 13.46998624 #> 414 pelvic_tilt 6.46680486 #> 415 pelvic_tilt 29.39654543 #> 416 pelvic_tilt 27.60260762 #> 417 pelvic_tilt 9.838262375 #> 418 pelvic_tilt 33.42595126 #> 419 pelvic_tilt 6.330910974 #> 420 pelvic_tilt 15.0822353 #> 421 pelvic_tilt 13.7062037 #> 422 pelvic_tilt 29.61009772 #> 423 pelvic_tilt -6.554948347 #> 424 pelvic_tilt 19.27929659 #> 425 pelvic_tilt 36.84317181 #> 426 pelvic_tilt 8.404475005 #> 427 pelvic_tilt 12.48948765 #> 428 pelvic_tilt 38.75066978 #> 429 pelvic_tilt 24.15748726 #> 430 pelvic_tilt 15.7538935 #> 431 pelvic_tilt 12.09507205 #> 432 pelvic_tilt 23.07742686 #> 433 pelvic_tilt 48.06953097 #> 434 pelvic_tilt 10.54067533 #> 435 pelvic_tilt 14.32167879 #> 436 pelvic_tilt 5.687032126 #> 437 pelvic_tilt 21.70440224 #> 438 pelvic_tilt 16.998479 #> 439 pelvic_tilt 28.27250132 #> 440 pelvic_tilt 16.73762214 #> 441 pelvic_tilt 9.120340183 #> 442 pelvic_tilt 13.77746531 #> 443 pelvic_tilt 21.12275138 #> 444 pelvic_tilt 20.12346562 #> 445 pelvic_tilt 17.21267289 #> 446 pelvic_tilt 30.3498745 #> 447 pelvic_tilt 39.84466878 #> 448 pelvic_tilt 34.31098931 #> 449 pelvic_tilt 24.70073725 #> 450 pelvic_tilt 21.06989651 #> 451 pelvic_tilt 15.4011391 #> 452 pelvic_tilt 48.90365265 #> 453 pelvic_tilt 18.27888963 #> 454 pelvic_tilt 20.5959577 #> 455 pelvic_tilt 14.30965614 #> 456 pelvic_tilt 42.68919513 #> 457 pelvic_tilt 30.45703858 #> 458 pelvic_tilt -3.759929872 #> 459 pelvic_tilt 9.832874231 #> 460 pelvic_tilt 23.94482471 #> 461 pelvic_tilt 20.69044356 #> 462 pelvic_tilt 3.969814743 #> 463 pelvic_tilt 14.11532726 #> 464 pelvic_tilt 15.1493501 #> 465 pelvic_tilt 5.792973871 #> 466 pelvic_tilt 14.55160171 #> 467 pelvic_tilt 26.73226755 #> 468 pelvic_tilt 1.507074501 #> 469 pelvic_tilt 0.34572799 #> 470 pelvic_tilt 12.50864276 #> 471 pelvic_tilt 35.39267395 #> 472 pelvic_tilt 7.194661096 #> 473 pelvic_tilt 38.44950127 #> 474 pelvic_tilt 37.51543601 #> 475 pelvic_tilt 9.306594428 #> 476 pelvic_tilt 20.26822858 #> 477 pelvic_tilt 6.87408897 #> 478 pelvic_tilt 16.42078962 #> 479 pelvic_tilt 24.82263131 #> 480 pelvic_tilt 1.101086714 #> 481 pelvic_tilt 15.17407796 #> 482 pelvic_tilt 14.04225971 #> 483 pelvic_tilt 13.45820343 #> 484 pelvic_tilt 9.064729049 #> 485 pelvic_tilt 25.38436364 #> 486 pelvic_tilt 8.961261611 #> 487 pelvic_tilt 13.28150221 #> 488 pelvic_tilt 19.23898066 #> 489 pelvic_tilt 26.34437939 #> 490 pelvic_tilt 49.4318636 #> 491 pelvic_tilt 4.47909896 #> 492 pelvic_tilt 15.22530262 #> 493 pelvic_tilt 31.53945399 #> 494 pelvic_tilt 37.51577195 #> 495 pelvic_tilt 20.80149217 #> 496 pelvic_tilt 24.50817744 #> 497 pelvic_tilt 21.25584028 #> 498 pelvic_tilt 5.265665422 #> 499 pelvic_tilt 38.65003527 #> 500 pelvic_tilt 29.27642195 #> 501 pelvic_tilt 9.811985315 #> 502 pelvic_tilt 40.30376567 #> 503 pelvic_tilt 33.28315665 #> 504 pelvic_tilt 10.34012252 #> 505 pelvic_tilt 18.92911726 #> 506 pelvic_tilt 5.268270454 #> 507 pelvic_tilt 12.76338484 #> 508 pelvic_tilt 37.57787321 #> 509 pelvic_tilt 13.90908417 #> 510 pelvic_tilt 16.67148361 #> 511 pelvic_tilt 20.02462134 #> 512 pelvic_tilt 33.2755899 #> 513 pelvic_tilt 41.93368293 #> 514 pelvic_tilt 9.711317947 #> 515 pelvic_tilt 14.37718927 #> 516 pelvic_tilt 33.94243223 #> 517 pelvic_tilt 46.55005318 #> 518 pelvic_tilt 18.82372712 #> 519 pelvic_tilt 20.36561331 #> 520 pelvic_tilt 16.41746236 #> 521 pelvic_tilt 16.96429691 #> 522 pelvic_tilt 18.96842952 #> 523 pelvic_tilt 8.945434892 #> 524 pelvic_tilt 17.45212105 #> 525 pelvic_tilt 10.65985935 #> 526 pelvic_tilt 13.35496594 #> 527 pelvic_tilt 6.6769999 #> 528 pelvic_tilt 6.557617408 #> 529 pelvic_tilt 15.96934373 #> 530 pelvic_tilt 26.32836901 #> 531 pelvic_tilt 21.78643263 #> 532 pelvic_tilt 5.865353416 #> 533 pelvic_tilt 13.10630665 #> 534 pelvic_tilt 18.89840693 #> 535 pelvic_tilt 22.63921678 #> 536 pelvic_tilt 7.724872599 #> 537 pelvic_tilt 16.06094486 #> 538 pelvic_tilt 19.67695713 #> 539 pelvic_tilt 8.30166942 #> 540 pelvic_tilt 10.09574326 #> 541 pelvic_tilt 23.13791922 #> 542 pelvic_tilt 12.93931796 #> 543 pelvic_tilt 13.81574355 #> 544 pelvic_tilt 9.148437195 #> 545 pelvic_tilt 9.386298276 #> 546 pelvic_tilt 19.97109671 #> 547 pelvic_tilt 13.59710457 #> 548 pelvic_tilt 13.96097523 #> 549 pelvic_tilt 13.29178975 #> 550 pelvic_tilt 19.44449915 #> 551 pelvic_tilt 8.835549101 #> 552 pelvic_tilt 13.51784732 #> 553 pelvic_tilt 5.536602477 #> 554 pelvic_tilt 7.514782784 #> 555 pelvic_tilt 27.33624023 #> 556 pelvic_tilt 10.68869819 #> 557 pelvic_tilt 15.85371711 #> 558 pelvic_tilt 16.73643493 #> 559 pelvic_tilt 8.573680295 #> 560 pelvic_tilt 20.23505957 #> 561 pelvic_tilt -0.810514093 #> 562 pelvic_tilt 1.835524271 #> 563 pelvic_tilt -5.845994341 #> 564 pelvic_tilt 21.34532339 #> 565 pelvic_tilt 19.57697146 #> 566 pelvic_tilt 14.65504222 #> 567 pelvic_tilt 9.752519649 #> 568 pelvic_tilt -2.970024337 #> 569 pelvic_tilt 10.19474845 #> 570 pelvic_tilt 16.93450781 #> 571 pelvic_tilt 11.93511014 #> 572 pelvic_tilt 14.92170492 #> 573 pelvic_tilt 14.37567126 #> 574 pelvic_tilt 3.675109986 #> 575 pelvic_tilt 6.819910138 #> 576 pelvic_tilt 9.594216702 #> 577 pelvic_tilt 10.21590237 #> 578 pelvic_tilt 9.410371613 #> 579 pelvic_tilt 16.48909145 #> 580 pelvic_tilt 16.48123972 #> 581 pelvic_tilt 8.875541276 #> 582 pelvic_tilt 16.54121618 #> 583 pelvic_tilt 7.011261806 #> 584 pelvic_tilt 1.112373561 #> 585 pelvic_tilt 9.976663803 #> 586 pelvic_tilt 16.7175142 #> 587 pelvic_tilt 13.63122319 #> 588 pelvic_tilt 13.20692644 #> 589 pelvic_tilt -1.329412398 #> 590 pelvic_tilt 18.01776202 #> 591 pelvic_tilt 13.43004422 #> 592 pelvic_tilt 14.49786554 #> 593 pelvic_tilt 13.44702168 #> 594 pelvic_tilt 13.11382047 #> 595 pelvic_tilt 14.56274875 #> 596 pelvic_tilt 16.55066167 #> 597 pelvic_tilt 17.11431203 #> 598 pelvic_tilt -0.324678459 #> 599 pelvic_tilt 15.72431994 #> 600 pelvic_tilt 14.17426387 #> 601 pelvic_tilt 13.87942449 #> 602 pelvic_tilt 14.20993529 #> 603 pelvic_tilt 2.631739646 #> 604 pelvic_tilt 5.587588658 #> 605 pelvic_tilt 10.0627701 #> 606 pelvic_tilt 6.620795049 #> 607 pelvic_tilt 16.20883944 #> 608 pelvic_tilt 18.75913544 #> 609 pelvic_tilt 20.89767207 #> 610 pelvic_tilt 29.89411893 #> 611 pelvic_tilt 6.461501271 #> 612 pelvic_tilt 26.07598143 #> 613 pelvic_tilt 21.48897426 #> 614 pelvic_tilt 2.062682882 #> 615 pelvic_tilt 12.30695118 #> 616 pelvic_tilt 13.61668819 #> 617 pelvic_tilt 20.72149628 #> 618 pelvic_tilt 22.6949683 #> 619 pelvic_tilt 8.693157364 #> 620 pelvic_tilt 5.073991409 #> 621 lumbar_lordosis_angle 39.60911701 #> 622 lumbar_lordosis_angle 25.01537822 #> 623 lumbar_lordosis_angle 50.09219357 #> 624 lumbar_lordosis_angle 44.31123813 #> 625 lumbar_lordosis_angle 28.317406 #> 626 lumbar_lordosis_angle 25.1249496 #> 627 lumbar_lordosis_angle 37.16593387 #> 628 lumbar_lordosis_angle 29.03834896 #> 629 lumbar_lordosis_angle 42.69081398 #> 630 lumbar_lordosis_angle 41.9487509 #> 631 lumbar_lordosis_angle 31.33450009 #> 632 lumbar_lordosis_angle 15.5 #> 633 lumbar_lordosis_angle 40.26379358 #> 634 lumbar_lordosis_angle 33.1 #> 635 lumbar_lordosis_angle 46.99999999 #> 636 lumbar_lordosis_angle 36.098763 #> 637 lumbar_lordosis_angle 54.55243367 #> 638 lumbar_lordosis_angle 32.56299592 #> 639 lumbar_lordosis_angle 31 #> 640 lumbar_lordosis_angle 30.12258646 #> 641 lumbar_lordosis_angle 37.8325467 #> 642 lumbar_lordosis_angle 42.19999999 #> 643 lumbar_lordosis_angle 53.99999999 #> 644 lumbar_lordosis_angle 30.29832059 #> 645 lumbar_lordosis_angle 29 #> 646 lumbar_lordosis_angle 35.32974693 #> 647 lumbar_lordosis_angle 14 #> 648 lumbar_lordosis_angle 46.99999999 #> 649 lumbar_lordosis_angle 26.78591597 #> 650 lumbar_lordosis_angle 49.27859673 #> 651 lumbar_lordosis_angle 42.52893886 #> 652 lumbar_lordosis_angle 32.13655345 #> 653 lumbar_lordosis_angle 27.78057555 #> 654 lumbar_lordosis_angle 39.99999999 #> 655 lumbar_lordosis_angle 46.56025198 #> 656 lumbar_lordosis_angle 24.28481815 #> 657 lumbar_lordosis_angle 35.99819848 #> 658 lumbar_lordosis_angle 20.7 #> 659 lumbar_lordosis_angle 47.69054322 #> 660 lumbar_lordosis_angle 35.87265953 #> 661 lumbar_lordosis_angle 15.59036345 #> 662 lumbar_lordosis_angle 29.0372302 #> 663 lumbar_lordosis_angle 32.77905978 #> 664 lumbar_lordosis_angle 47.49999999 #> 665 lumbar_lordosis_angle 62.27527456 #> 666 lumbar_lordosis_angle 46.99999999 #> 667 lumbar_lordosis_angle 36.18199318 #> 668 lumbar_lordosis_angle 30.70619135 #> 669 lumbar_lordosis_angle 34 #> 670 lumbar_lordosis_angle 20.0308863 #> 671 lumbar_lordosis_angle 34 #> 672 lumbar_lordosis_angle 27.7 #> 673 lumbar_lordosis_angle 36.10400731 #> 674 lumbar_lordosis_angle 34 #> 675 lumbar_lordosis_angle 33.46940277 #> 676 lumbar_lordosis_angle 36.67998541 #> 677 lumbar_lordosis_angle 28.06548279 #> 678 lumbar_lordosis_angle 38 #> 679 lumbar_lordosis_angle 35 #> 680 lumbar_lordosis_angle 35.56468278 #> 681 lumbar_lordosis_angle 78.77201304 #> 682 lumbar_lordosis_angle 83.13073216 #> 683 lumbar_lordosis_angle 51.99999999 #> 684 lumbar_lordosis_angle 64.42944191 #> 685 lumbar_lordosis_angle 82.96150249 #> 686 lumbar_lordosis_angle 61.99999999 #> 687 lumbar_lordosis_angle 59.99999999 #> 688 lumbar_lordosis_angle 64.14868477 #> 689 lumbar_lordosis_angle 50.99999999 #> 690 lumbar_lordosis_angle 51.49999999 #> 691 lumbar_lordosis_angle 51.99999999 #> 692 lumbar_lordosis_angle 47.79434664 #> 693 lumbar_lordosis_angle 60.85987263 #> 694 lumbar_lordosis_angle 43.99999999 #> 695 lumbar_lordosis_angle 90.99999999 #> 696 lumbar_lordosis_angle 68.11840309 #> 697 lumbar_lordosis_angle 69.22104479 #> 698 lumbar_lordosis_angle 53.33894082 #> 699 lumbar_lordosis_angle 60.14464036 #> 700 lumbar_lordosis_angle 38.99999999 #> 701 lumbar_lordosis_angle 69.48062839 #> 702 lumbar_lordosis_angle 57.37950226 #> 703 lumbar_lordosis_angle 47.56426247 #> 704 lumbar_lordosis_angle 77.88702048 #> 705 lumbar_lordosis_angle 57.19999999 #> 706 lumbar_lordosis_angle 44.99999999 #> 707 lumbar_lordosis_angle 59.20646143 #> 708 lumbar_lordosis_angle 44.63091389 #> 709 lumbar_lordosis_angle 41.99999999 #> 710 lumbar_lordosis_angle 43.6966651 #> 711 lumbar_lordosis_angle 58.23282055 #> 712 lumbar_lordosis_angle 62.85910914 #> 713 lumbar_lordosis_angle 71.66865979 #> 714 lumbar_lordosis_angle 79.64983825 #> 715 lumbar_lordosis_angle 67.70572132 #> 716 lumbar_lordosis_angle 50.90985841 #> 717 lumbar_lordosis_angle 90.21149828 #> 718 lumbar_lordosis_angle 82.73535954 #> 719 lumbar_lordosis_angle 93.89277881 #> 720 lumbar_lordosis_angle 41.46785522 #> 721 lumbar_lordosis_angle 65.47948563 #> 722 lumbar_lordosis_angle 63.31183486 #> 723 lumbar_lordosis_angle 61.19999999 #> 724 lumbar_lordosis_angle 52.99999999 #> 725 lumbar_lordosis_angle 63.23230243 #> 726 lumbar_lordosis_angle 50.94751899 #> 727 lumbar_lordosis_angle 57.73583722 #> 728 lumbar_lordosis_angle 76.27743927 #> 729 lumbar_lordosis_angle 62.99999999 #> 730 lumbar_lordosis_angle 63.01469619 #> 731 lumbar_lordosis_angle 62.12433389 #> 732 lumbar_lordosis_angle 83.35219438 #> 733 lumbar_lordosis_angle 67.89999999 #> 734 lumbar_lordosis_angle 48.49999999 #> 735 lumbar_lordosis_angle 86.96060151 #> 736 lumbar_lordosis_angle 48.38405705 #> 737 lumbar_lordosis_angle 62.41714208 #> 738 lumbar_lordosis_angle 47.87140494 #> 739 lumbar_lordosis_angle 45.77516991 #> 740 lumbar_lordosis_angle 43.19915768 #> 741 lumbar_lordosis_angle 40.99999999 #> 742 lumbar_lordosis_angle 87.14151223 #> 743 lumbar_lordosis_angle 52.40343873 #> 744 lumbar_lordosis_angle 56.48913545 #> 745 lumbar_lordosis_angle 32.5 #> 746 lumbar_lordosis_angle 57.05716117 #> 747 lumbar_lordosis_angle 59.18116082 #> 748 lumbar_lordosis_angle 66.53601753 #> 749 lumbar_lordosis_angle 69.8139423 #> 750 lumbar_lordosis_angle 49.77553438 #> 751 lumbar_lordosis_angle 32.16846267 #> 752 lumbar_lordosis_angle 57.99999999 #> 753 lumbar_lordosis_angle 52.76659472 #> 754 lumbar_lordosis_angle 70.56044038 #> 755 lumbar_lordosis_angle 78.09496877 #> 756 lumbar_lordosis_angle 77.48108264 #> 757 lumbar_lordosis_angle 81.77447308 #> 758 lumbar_lordosis_angle 78.42329287 #> 759 lumbar_lordosis_angle 79.87401586 #> 760 lumbar_lordosis_angle 91.73479193 #> 761 lumbar_lordosis_angle 74.43849743 #> 762 lumbar_lordosis_angle 72.0034229 #> 763 lumbar_lordosis_angle 100.7442198 #> 764 lumbar_lordosis_angle 64.53526221 #> 765 lumbar_lordosis_angle 58.03886519 #> 766 lumbar_lordosis_angle 78.7506635 #> 767 lumbar_lordosis_angle 78.23137949 #> 768 lumbar_lordosis_angle 55.99999999 #> 769 lumbar_lordosis_angle 50.82289501 #> 770 lumbar_lordosis_angle 40.79669829 #> 771 lumbar_lordosis_angle 60.68700588 #> 772 lumbar_lordosis_angle 58.34451924 #> 773 lumbar_lordosis_angle 48.13680562 #> 774 lumbar_lordosis_angle 63.99999999 #> 775 lumbar_lordosis_angle 42.86739151 #> 776 lumbar_lordosis_angle 72.08491177 #> 777 lumbar_lordosis_angle 70.65098189 #> 778 lumbar_lordosis_angle 46.11033909 #> 779 lumbar_lordosis_angle 49.19800263 #> 780 lumbar_lordosis_angle 68.70237672 #> 781 lumbar_lordosis_angle 77.41696348 #> 782 lumbar_lordosis_angle 51.69688681 #> 783 lumbar_lordosis_angle 50.83851954 #> 784 lumbar_lordosis_angle 76.79999999 #> 785 lumbar_lordosis_angle 43.10049819 #> 786 lumbar_lordosis_angle 77.1105979 #> 787 lumbar_lordosis_angle 57.00900516 #> 788 lumbar_lordosis_angle 59.86901238 #> 789 lumbar_lordosis_angle 95.15763273 #> 790 lumbar_lordosis_angle 38 #> 791 lumbar_lordosis_angle 58.83999352 #> 792 lumbar_lordosis_angle 79.69426258 #> 793 lumbar_lordosis_angle 43.76970978 #> 794 lumbar_lordosis_angle 56.29999999 #> 795 lumbar_lordosis_angle 39.09686927 #> 796 lumbar_lordosis_angle 52.57784639 #> 797 lumbar_lordosis_angle 66.15040334 #> 798 lumbar_lordosis_angle 61.64245116 #> 799 lumbar_lordosis_angle 60.89811835 #> 800 lumbar_lordosis_angle 68.0560124 #> 801 lumbar_lordosis_angle 24.71027447 #> 802 lumbar_lordosis_angle 67.63216653 #> 803 lumbar_lordosis_angle 89.59999999 #> 804 lumbar_lordosis_angle 84.53709256 #> 805 lumbar_lordosis_angle 91.78449512 #> 806 lumbar_lordosis_angle 84.62027202 #> 807 lumbar_lordosis_angle 78.76675639 #> 808 lumbar_lordosis_angle 59.8142356 #> 809 lumbar_lordosis_angle 82.68097744 #> 810 lumbar_lordosis_angle 77.05456489 #> 811 lumbar_lordosis_angle 51.99999999 #> 812 lumbar_lordosis_angle 61.14101155 #> 813 lumbar_lordosis_angle 66.94210105 #> 814 lumbar_lordosis_angle 76.37007032 #> 815 lumbar_lordosis_angle 67.99999999 #> 816 lumbar_lordosis_angle 85.99958417 #> 817 lumbar_lordosis_angle 65.36052425 #> 818 lumbar_lordosis_angle 125.7423855 #> 819 lumbar_lordosis_angle 62.69325884 #> 820 lumbar_lordosis_angle 61.29620362 #> 821 lumbar_lordosis_angle 67.49870507 #> 822 lumbar_lordosis_angle 96.28306169 #> 823 lumbar_lordosis_angle 93.2848628 #> 824 lumbar_lordosis_angle 62.99999999 #> 825 lumbar_lordosis_angle 44.99154663 #> 826 lumbar_lordosis_angle 85.10160773 #> 827 lumbar_lordosis_angle 58.99999999 #> 828 lumbar_lordosis_angle 76.03215571 #> 829 lumbar_lordosis_angle 93.82241589 #> 830 lumbar_lordosis_angle 36.32913708 #> 831 lumbar_lordosis_angle 35.11281407 #> 832 lumbar_lordosis_angle 51.60145541 #> 833 lumbar_lordosis_angle 46.90209626 #> 834 lumbar_lordosis_angle 47.99999999 #> 835 lumbar_lordosis_angle 42.5778464 #> 836 lumbar_lordosis_angle 35.90352597 #> 837 lumbar_lordosis_angle 30.89652243 #> 838 lumbar_lordosis_angle 50.44507473 #> 839 lumbar_lordosis_angle 35 #> 840 lumbar_lordosis_angle 50.95896417 #> 841 lumbar_lordosis_angle 31.47415392 #> 842 lumbar_lordosis_angle 52.99999999 #> 843 lumbar_lordosis_angle 62.63701952 #> 844 lumbar_lordosis_angle 75.96636144 #> 845 lumbar_lordosis_angle 90.56346144 #> 846 lumbar_lordosis_angle 55.34348527 #> 847 lumbar_lordosis_angle 63.12373633 #> 848 lumbar_lordosis_angle 52.89222856 #> 849 lumbar_lordosis_angle 26.23683004 #> 850 lumbar_lordosis_angle 36.03222439 #> 851 lumbar_lordosis_angle 62.58217893 #> 852 lumbar_lordosis_angle 38.99999999 #> 853 lumbar_lordosis_angle 40.34738779 #> 854 lumbar_lordosis_angle 31.02159252 #> 855 lumbar_lordosis_angle 41.99999999 #> 856 lumbar_lordosis_angle 40.17704963 #> 857 lumbar_lordosis_angle 63.99999999 #> 858 lumbar_lordosis_angle 57.99999999 #> 859 lumbar_lordosis_angle 55.5701429 #> 860 lumbar_lordosis_angle 43.5778464 #> 861 lumbar_lordosis_angle 36.03197484 #> 862 lumbar_lordosis_angle 35 #> 863 lumbar_lordosis_angle 26.93203835 #> 864 lumbar_lordosis_angle 42.99999999 #> 865 lumbar_lordosis_angle 51.60501665 #> 866 lumbar_lordosis_angle 53.99999999 #> 867 lumbar_lordosis_angle 39.99999999 #> 868 lumbar_lordosis_angle 28 #> 869 lumbar_lordosis_angle 35.56025198 #> 870 lumbar_lordosis_angle 37 #> 871 lumbar_lordosis_angle 33.62731353 #> 872 lumbar_lordosis_angle 49.99999999 #> 873 lumbar_lordosis_angle 57.99999999 #> 874 lumbar_lordosis_angle 65.99999999 #> 875 lumbar_lordosis_angle 61.00707117 #> 876 lumbar_lordosis_angle 58.00142908 #> 877 lumbar_lordosis_angle 47.99999999 #> 878 lumbar_lordosis_angle 41.99999999 #> 879 lumbar_lordosis_angle 37.96774659 #> 880 lumbar_lordosis_angle 49.34926218 #> 881 lumbar_lordosis_angle 42.99999999 #> 882 lumbar_lordosis_angle 53.73007172 #> 883 lumbar_lordosis_angle 25.32356538 #> 884 lumbar_lordosis_angle 25.5 #> 885 lumbar_lordosis_angle 46.99999999 #> 886 lumbar_lordosis_angle 39.71092029 #> 887 lumbar_lordosis_angle 42.69999999 #> 888 lumbar_lordosis_angle 46.98805181 #> 889 lumbar_lordosis_angle 42.84214764 #> 890 lumbar_lordosis_angle 24 #> 891 lumbar_lordosis_angle 56.99999999 #> 892 lumbar_lordosis_angle 41.99999999 #> 893 lumbar_lordosis_angle 37 #> 894 lumbar_lordosis_angle 43.45725694 #> 895 lumbar_lordosis_angle 28.93814927 #> 896 lumbar_lordosis_angle 50.99999999 #> 897 lumbar_lordosis_angle 33.25857782 #> 898 lumbar_lordosis_angle 43.99999999 #> 899 lumbar_lordosis_angle 30.98276809 #> 900 lumbar_lordosis_angle 51.99999999 #> 901 lumbar_lordosis_angle 34.45754051 #> 902 lumbar_lordosis_angle 43.90250363 #> 903 lumbar_lordosis_angle 41.58429713 #> 904 lumbar_lordosis_angle 51.87351997 #> 905 lumbar_lordosis_angle 43.19915768 #> 906 lumbar_lordosis_angle 43.25680184 #> 907 lumbar_lordosis_angle 46.89999999 #> 908 lumbar_lordosis_angle 19.0710746 #> 909 lumbar_lordosis_angle 58.61858244 #> 910 lumbar_lordosis_angle 32.2434952 #> 911 lumbar_lordosis_angle 20.24256187 #> 912 lumbar_lordosis_angle 35.95122893 #> 913 lumbar_lordosis_angle 29.50438112 #> 914 lumbar_lordosis_angle 55.49999999 #> 915 lumbar_lordosis_angle 37 #> 916 lumbar_lordosis_angle 48.09999999 #> 917 lumbar_lordosis_angle 36.67485694 #> 918 lumbar_lordosis_angle 33.77414297 #> 919 lumbar_lordosis_angle 31.72747138 #> 920 lumbar_lordosis_angle 58.25054221 #> 921 lumbar_lordosis_angle 35 #> 922 lumbar_lordosis_angle 69.02125897 #> 923 lumbar_lordosis_angle 29.36021618 #> 924 lumbar_lordosis_angle 32.39081996 #> 925 lumbar_lordosis_angle 44.58317718 #> 926 lumbar_lordosis_angle 36 #> 927 lumbar_lordosis_angle 29.22053381 #> 928 lumbar_lordosis_angle 46.17034732 #> 929 lumbar_lordosis_angle 41.5831264 #> 930 lumbar_lordosis_angle 36.64123294 #> 931 sacral_slope 40.47523153 #> 932 sacral_slope 28.99595951 #> 933 sacral_slope 46.61353893 #> 934 sacral_slope 44.64413017 #> 935 sacral_slope 40.06078446 #> 936 sacral_slope 26.32829311 #> 937 sacral_slope 37.56859203 #> 938 sacral_slope 34.61114218 #> 939 sacral_slope 30.25643716 #> 940 sacral_slope 31.67546874 #> 941 sacral_slope 36.66563548 #> 942 sacral_slope 13.51656811 #> 943 sacral_slope 28.95099521 #> 944 sacral_slope 33.11134196 #> 945 sacral_slope 33.11134196 #> 946 sacral_slope 31.78091509 #> 947 sacral_slope 43.47247456 #> 948 sacral_slope 28.13134236 #> 949 sacral_slope 25.25316339 #> 950 sacral_slope 29.475889 #> 951 sacral_slope 29.7448813 #> 952 sacral_slope 33.85711014 #> 953 sacral_slope 38.65980825 #> 954 sacral_slope 32.47119229 #> 955 sacral_slope 13.3669307 #> 956 sacral_slope 27.47443163 #> 957 sacral_slope 15.38846783 #> 958 sacral_slope 27.07208024 #> 959 sacral_slope 22.61986495 #> 960 sacral_slope 41.9872125 #> 961 sacral_slope 35.41705528 #> 962 sacral_slope 35.31121344 #> 963 sacral_slope 27.49283695 #> 964 sacral_slope 25.67681568 #> 965 sacral_slope 27.59729587 #> 966 sacral_slope 23.657997 #> 967 sacral_slope 25.10160871 #> 968 sacral_slope 16.26020471 #> 969 sacral_slope 26.99583839 #> 970 sacral_slope 33.40782459 #> 971 sacral_slope 23.79077387 #> 972 sacral_slope 38.04704253 #> 973 sacral_slope 34.62415508 #> 974 sacral_slope 39.95754893 #> 975 sacral_slope 39.73230287 #> 976 sacral_slope 27.89727103 #> 977 sacral_slope 26.10485401 #> 978 sacral_slope 24.77514057 #> 979 sacral_slope 22.57957256 #> 980 sacral_slope 23.86833001 #> 981 sacral_slope 34.84573342 #> 982 sacral_slope 32.87626175 #> 983 sacral_slope 20.44954761 #> 984 sacral_slope 18.23249108 #> 985 sacral_slope 23.85047391 #> 986 sacral_slope 34.38034472 #> 987 sacral_slope 35.88213725 #> 988 sacral_slope 31.50426672 #> 989 sacral_slope 23.54003927 #> 990 sacral_slope 33.17851166 #> 991 sacral_slope 42.32457334 #> 992 sacral_slope 56.97613244 #> 993 sacral_slope 35.09581679 #> 994 sacral_slope 56.30993248 #> 995 sacral_slope 54.21102654 #> 996 sacral_slope 42.64670314 #> 997 sacral_slope 56.30993248 #> 998 sacral_slope 56.30993248 #> 999 sacral_slope 53.13010236 #> 1000 sacral_slope 58.86102756 #> 1001 sacral_slope 55.17551084 #> 1002 sacral_slope 53.97262661 #> 1003 sacral_slope 51.95295747 #> 1004 sacral_slope 35.41705528 #> 1005 sacral_slope 49.14462374 #> 1006 sacral_slope 30.39872771 #> 1007 sacral_slope 50.71059314 #> 1008 sacral_slope 51.11550357 #> 1009 sacral_slope 49.96974073 #> 1010 sacral_slope 35.6553281 #> 1011 sacral_slope 46.63657704 #> 1012 sacral_slope 52.88313932 #> 1013 sacral_slope 59.53445508 #> 1014 sacral_slope 56.30993247 #> 1015 sacral_slope 33.92979742 #> 1016 sacral_slope 35.53767779 #> 1017 sacral_slope 41.90594194 #> 1018 sacral_slope 34.69515353 #> 1019 sacral_slope 39.80557109 #> 1020 sacral_slope 47.29061004 #> 1021 sacral_slope 52.9071627 #> 1022 sacral_slope 50.7927965 #> 1023 sacral_slope 69.50740523 #> 1024 sacral_slope 43.26429541 #> 1025 sacral_slope 78.79405249 #> 1026 sacral_slope 23.87528085 #> 1027 sacral_slope 77.19573393 #> 1028 sacral_slope 54.96380419 #> 1029 sacral_slope 55.22216863 #> 1030 sacral_slope 44.59933674 #> 1031 sacral_slope 54.22392228 #> 1032 sacral_slope 61.16449915 #> 1033 sacral_slope 56.92932218 #> 1034 sacral_slope 43.31531568 #> 1035 sacral_slope 48.0127875 #> 1036 sacral_slope 37.40535663 #> 1037 sacral_slope 55.17551084 #> 1038 sacral_slope 45 #> 1039 sacral_slope 56.84207612 #> 1040 sacral_slope 53.53076561 #> 1041 sacral_slope 50.19442891 #> 1042 sacral_slope 55.38885782 #> 1043 sacral_slope 48.57633437 #> 1044 sacral_slope 50.47736873 #> 1045 sacral_slope 44.1449026 #> 1046 sacral_slope 121.4295656 #> 1047 sacral_slope 57.99461679 #> 1048 sacral_slope 47.29061004 #> 1049 sacral_slope 41.3785153 #> 1050 sacral_slope 45 #> 1051 sacral_slope 42.64670314 #> 1052 sacral_slope 60.80251395 #> 1053 sacral_slope 32.00538321 #> 1054 sacral_slope 55.12467166 #> 1055 sacral_slope 60.39554926 #> 1056 sacral_slope 42.37359436 #> 1057 sacral_slope 48.97249594 #> 1058 sacral_slope 63.43494882 #> 1059 sacral_slope 62.2414594 #> 1060 sacral_slope 60.49927538 #> 1061 sacral_slope 40.94644577 #> 1062 sacral_slope 56.00354085 #> 1063 sacral_slope 48.50353164 #> 1064 sacral_slope 61.63095371 #> 1065 sacral_slope 34.9920202 #> 1066 sacral_slope 46.77146974 #> 1067 sacral_slope 48.17983012 #> 1068 sacral_slope 49.08561678 #> 1069 sacral_slope 47.35329687 #> 1070 sacral_slope 64.02560604 #> 1071 sacral_slope 54.16234705 #> 1072 sacral_slope 40.60129465 #> 1073 sacral_slope 67.0112832 #> 1074 sacral_slope 40.03025927 #> 1075 sacral_slope 45.73452103 #> 1076 sacral_slope 42.95459151 #> 1077 sacral_slope 55.12467166 #> 1078 sacral_slope 58.84069549 #> 1079 sacral_slope 55.92280472 #> 1080 sacral_slope 55.30484647 #> 1081 sacral_slope 60.42216132 #> 1082 sacral_slope 44.06080905 #> 1083 sacral_slope 49.28915333 #> 1084 sacral_slope 42.13759477 #> 1085 sacral_slope 35.39479584 #> 1086 sacral_slope 52.25319461 #> 1087 sacral_slope 52.74471055 #> 1088 sacral_slope 42.70938996 #> 1089 sacral_slope 56.68936918 #> 1090 sacral_slope 51.76617482 #> 1091 sacral_slope 56.633634 #> 1092 sacral_slope 60.06848816 #> 1093 sacral_slope 79.69515353 #> 1094 sacral_slope 78.40782459 #> 1095 sacral_slope 44.63506366 #> 1096 sacral_slope 63.43494882 #> 1097 sacral_slope 50.11731485 #> 1098 sacral_slope 55.92280472 #> 1099 sacral_slope 70.55996517 #> 1100 sacral_slope 43.15238973 #> 1101 sacral_slope 49.63546343 #> 1102 sacral_slope 64.35899418 #> 1103 sacral_slope 43.21008939 #> 1104 sacral_slope 41.7602997 #> 1105 sacral_slope 36.02737339 #> 1106 sacral_slope 47.6025622 #> 1107 sacral_slope 53.74616226 #> 1108 sacral_slope 61.57879078 #> 1109 sacral_slope 54.30994017 #> 1110 sacral_slope 19.29004622 #> 1111 sacral_slope 33.42481118 #> 1112 sacral_slope 49.39870535 #> 1113 sacral_slope 43.89829388 #> 1114 sacral_slope 33.48616882 #> 1115 sacral_slope 60.2551187 #> 1116 sacral_slope 66.96056402 #> 1117 sacral_slope 59.82647997 #> 1118 sacral_slope 55.15426658 #> 1119 sacral_slope 47.03091424 #> 1120 sacral_slope 53.13010235 #> 1121 sacral_slope 33.90627699 #> 1122 sacral_slope 46.16913933 #> 1123 sacral_slope 41.18592517 #> 1124 sacral_slope 59.91031376 #> 1125 sacral_slope 53.71473287 #> 1126 sacral_slope 65.97349342 #> 1127 sacral_slope 51.00900596 #> 1128 sacral_slope 21.25050551 #> 1129 sacral_slope 60.9453959 #> 1130 sacral_slope 58.62699486 #> 1131 sacral_slope 43.33971763 #> 1132 sacral_slope 34.23746278 #> 1133 sacral_slope 34.38034472 #> 1134 sacral_slope 63.92464442 #> 1135 sacral_slope 42.15786212 #> 1136 sacral_slope 46.16913933 #> 1137 sacral_slope 48.93017555 #> 1138 sacral_slope 55.27100372 #> 1139 sacral_slope 67.31347333 #> 1140 sacral_slope 31.84245726 #> 1141 sacral_slope 21.54097592 #> 1142 sacral_slope 35.952428 #> 1143 sacral_slope 35.41705528 #> 1144 sacral_slope 30.86680945 #> 1145 sacral_slope 35.0419294 #> 1146 sacral_slope 17.38697218 #> 1147 sacral_slope 44.23610154 #> 1148 sacral_slope 31.56897113 #> 1149 sacral_slope 35.6553281 #> 1150 sacral_slope 37.98349826 #> 1151 sacral_slope 22.70284212 #> 1152 sacral_slope 49.08561678 #> 1153 sacral_slope 42.99746687 #> 1154 sacral_slope 50.50041147 #> 1155 sacral_slope 67.19545953 #> 1156 sacral_slope 52.00126756 #> 1157 sacral_slope 47.8985768 #> 1158 sacral_slope 41.86364163 #> 1159 sacral_slope 29.7448813 #> 1160 sacral_slope 33.34070735 #> 1161 sacral_slope 42.47388309 #> 1162 sacral_slope 40.97173633 #> 1163 sacral_slope 29.30220748 #> 1164 sacral_slope 31.53479191 #> 1165 sacral_slope 28.34569362 #> 1166 sacral_slope 43.95837332 #> 1167 sacral_slope 48.22452261 #> 1168 sacral_slope 48.17983012 #> 1169 sacral_slope 55.71312302 #> 1170 sacral_slope 37.00252653 #> 1171 sacral_slope 32.8113668 #> 1172 sacral_slope 38.01151027 #> 1173 sacral_slope 33.55066201 #> 1174 sacral_slope 27.13513962 #> 1175 sacral_slope 35.69005983 #> 1176 sacral_slope 37.11686068 #> 1177 sacral_slope 30.78414653 #> 1178 sacral_slope 33.09169994 #> 1179 sacral_slope 38.74596726 #> 1180 sacral_slope 30.51823068 #> 1181 sacral_slope 36.96834391 #> 1182 sacral_slope 38.91147185 #> 1183 sacral_slope 48.76403486 #> 1184 sacral_slope 42.44710185 #> 1185 sacral_slope 53.37867251 #> 1186 sacral_slope 52.88313932 #> 1187 sacral_slope 45 #> 1188 sacral_slope 53.13010235 #> 1189 sacral_slope 30.15454792 #> 1190 sacral_slope 46.68468432 #> 1191 sacral_slope 42.20729763 #> 1192 sacral_slope 60.05431656 #> 1193 sacral_slope 28.14160123 #> 1194 sacral_slope 30.11373315 #> 1195 sacral_slope 47.68377516 #> 1196 sacral_slope 38.57652956 #> 1197 sacral_slope 36.15818544 #> 1198 sacral_slope 43.4518423 #> 1199 sacral_slope 40.65676005 #> 1200 sacral_slope 20.65891006 #> 1201 sacral_slope 42.43622979 #> 1202 sacral_slope 25.97439396 #> 1203 sacral_slope 32.3474435 #> 1204 sacral_slope 34.76519724 #> 1205 sacral_slope 33.21525149 #> 1206 sacral_slope 50.5721978 #> 1207 sacral_slope 37.69424047 #> 1208 sacral_slope 52.54942177 #> 1209 sacral_slope 41.74277806 #> 1210 sacral_slope 30.78414653 #> 1211 sacral_slope 36.65610842 #> 1212 sacral_slope 49.76364169 #> 1213 sacral_slope 40.23635831 #> 1214 sacral_slope 35.88213725 #> 1215 sacral_slope 44.60486296 #> 1216 sacral_slope 51.25403274 #> 1217 sacral_slope 44.6205633 #> 1218 sacral_slope 33.366366 #> 1219 sacral_slope 58.84069549 #> 1220 sacral_slope 30.25643716 #> 1221 sacral_slope 22.543061 #> 1222 sacral_slope 36.86989765 #> 1223 sacral_slope 32.12499844 #> 1224 sacral_slope 43.31531568 #> 1225 sacral_slope 36.17362905 #> 1226 sacral_slope 39.80557109 #> 1227 sacral_slope 23.44806258 #> 1228 sacral_slope 26.81634684 #> 1229 sacral_slope 45.60950658 #> 1230 sacral_slope 53.01123161 #> 1231 sacral_slope 44.2151754 #> 1232 sacral_slope 62.93889386 #> 1233 sacral_slope 33.11134196 #> 1234 sacral_slope 32.31961651 #> 1235 sacral_slope 32.76849908 #> 1236 sacral_slope 34.28687698 #> 1237 sacral_slope 33.21525149 #> 1238 sacral_slope 38.75162833 #> 1239 sacral_slope 36.55963472 #> 1240 sacral_slope 28.76764934 #> 1241 pelvic_radius 98.67291675 #> 1242 pelvic_radius 114.4054254 #> 1243 pelvic_radius 105.9851355 #> 1244 pelvic_radius 101.8684951 #> 1245 pelvic_radius 108.1687249 #> 1246 pelvic_radius 130.3278713 #> 1247 pelvic_radius 120.5675233 #> 1248 pelvic_radius 117.2700675 #> 1249 pelvic_radius 125.0028927 #> 1250 pelvic_radius 84.24141517 #> 1251 pelvic_radius 108.6482654 #> 1252 pelvic_radius 120.0553988 #> 1253 pelvic_radius 119.321358 #> 1254 pelvic_radius 110.9666978 #> 1255 pelvic_radius 116.8065868 #> 1256 pelvic_radius 124.1158358 #> 1257 pelvic_radius 112.3094915 #> 1258 pelvic_radius 129.0114183 #> 1259 pelvic_radius 123.1592507 #> 1260 pelvic_radius 116.5857056 #> 1261 pelvic_radius 134.4610156 #> 1262 pelvic_radius 125.2127163 #> 1263 pelvic_radius 106.4243295 #> 1264 pelvic_radius 117.9808303 #> 1265 pelvic_radius 115.5771163 #> 1266 pelvic_radius 121.447011 #> 1267 pelvic_radius 125.2032956 #> 1268 pelvic_radius 109.271634 #> 1269 pelvic_radius 111.0729197 #> 1270 pelvic_radius 113.4770183 #> 1271 pelvic_radius 112.192804 #> 1272 pelvic_radius 98.77454633 #> 1273 pelvic_radius 117.9803245 #> 1274 pelvic_radius 124.914118 #> 1275 pelvic_radius 119.3303537 #> 1276 pelvic_radius 113.8331446 #> 1277 pelvic_radius 132.264735 #> 1278 pelvic_radius 137.5406125 #> 1279 pelvic_radius 123.3118449 #> 1280 pelvic_radius 116.5597709 #> 1281 pelvic_radius 106.9388517 #> 1282 pelvic_radius 115.4814047 #> 1283 pelvic_radius 121.6709148 #> 1284 pelvic_radius 121.2196839 #> 1285 pelvic_radius 114.0231172 #> 1286 pelvic_radius 117.4222591 #> 1287 pelvic_radius 117.3846251 #> 1288 pelvic_radius 113.2666746 #> 1289 pelvic_radius 121.0462458 #> 1290 pelvic_radius 118.3633889 #> 1291 pelvic_radius 115.8770174 #> 1292 pelvic_radius 107.9493045 #> 1293 pelvic_radius 128.2925148 #> 1294 pelvic_radius 112.6841408 #> 1295 pelvic_radius 116.3778894 #> 1296 pelvic_radius 98.24978071 #> 1297 pelvic_radius 112.7761866 #> 1298 pelvic_radius 116.2509174 #> 1299 pelvic_radius 124.8461088 #> 1300 pelvic_radius 124.0564518 #> 1301 pelvic_radius 143.5606905 #> 1302 pelvic_radius 129.9554764 #> 1303 pelvic_radius 134.7117723 #> 1304 pelvic_radius 114.818751 #> 1305 pelvic_radius 123.9320096 #> 1306 pelvic_radius 115.012334 #> 1307 pelvic_radius 118.5303266 #> 1308 pelvic_radius 95.9036288 #> 1309 pelvic_radius 114.2130126 #> 1310 pelvic_radius 102.0428116 #> 1311 pelvic_radius 119.1937238 #> 1312 pelvic_radius 135.0753635 #> 1313 pelvic_radius 125.6595336 #> 1314 pelvic_radius 122.648753 #> 1315 pelvic_radius 137.7366546 #> 1316 pelvic_radius 148.5255624 #> 1317 pelvic_radius 139.414504 #> 1318 pelvic_radius 98.50115697 #> 1319 pelvic_radius 111.12397 #> 1320 pelvic_radius 117.5120039 #> 1321 pelvic_radius 112.1516 #> 1322 pelvic_radius 120.2059626 #> 1323 pelvic_radius 121.7647796 #> 1324 pelvic_radius 151.8398566 #> 1325 pelvic_radius 124.267007 #> 1326 pelvic_radius 163.0710405 #> 1327 pelvic_radius 119.3191109 #> 1328 pelvic_radius 130.0756599 #> 1329 pelvic_radius 127.2945222 #> 1330 pelvic_radius 119.8649383 #> 1331 pelvic_radius 114.7698556 #> 1332 pelvic_radius 116.1779325 #> 1333 pelvic_radius 124.4197875 #> 1334 pelvic_radius 113.5876551 #> 1335 pelvic_radius 114.8901128 #> 1336 pelvic_radius 140.9817119 #> 1337 pelvic_radius 120.6730408 #> 1338 pelvic_radius 109.3565941 #> 1339 pelvic_radius 123.0557067 #> 1340 pelvic_radius 115.514798 #> 1341 pelvic_radius 108.0102185 #> 1342 pelvic_radius 114.787107 #> 1343 pelvic_radius 102.3375244 #> 1344 pelvic_radius 110.8647831 #> 1345 pelvic_radius 118.4507311 #> 1346 pelvic_radius 116.5811088 #> 1347 pelvic_radius 94.73852542 #> 1348 pelvic_radius 138.5541111 #> 1349 pelvic_radius 110.6440206 #> 1350 pelvic_radius 123.4311742 #> 1351 pelvic_radius 114.1292425 #> 1352 pelvic_radius 126.9129899 #> 1353 pelvic_radius 111.5857819 #> 1354 pelvic_radius 96.49136982 #> 1355 pelvic_radius 141.0881494 #> 1356 pelvic_radius 107.690466 #> 1357 pelvic_radius 114.1900488 #> 1358 pelvic_radius 122.0929536 #> 1359 pelvic_radius 136.4403015 #> 1360 pelvic_radius 113.0533309 #> 1361 pelvic_radius 117.6432188 #> 1362 pelvic_radius 124.6460723 #> 1363 pelvic_radius 110.7099121 #> 1364 pelvic_radius 109.1627768 #> 1365 pelvic_radius 107.1822176 #> 1366 pelvic_radius 95.44375749 #> 1367 pelvic_radius 103.0083545 #> 1368 pelvic_radius 116.4389807 #> 1369 pelvic_radius 100.8921596 #> 1370 pelvic_radius 110.6903772 #> 1371 pelvic_radius 99.71245318 #> 1372 pelvic_radius 118.9306656 #> 1373 pelvic_radius 116.8030913 #> 1374 pelvic_radius 119.4250857 #> 1375 pelvic_radius 136.9725168 #> 1376 pelvic_radius 110.6111484 #> 1377 pelvic_radius 116.6015376 #> 1378 pelvic_radius 110.4665164 #> 1379 pelvic_radius 107.1723576 #> 1380 pelvic_radius 109.062312 #> 1381 pelvic_radius 105.0673556 #> 1382 pelvic_radius 134.6342912 #> 1383 pelvic_radius 110.6607005 #> 1384 pelvic_radius 117.2255542 #> 1385 pelvic_radius 105.1316639 #> 1386 pelvic_radius 105.1440758 #> 1387 pelvic_radius 114.8660487 #> 1388 pelvic_radius 109.9153669 #> 1389 pelvic_radius 104.3949585 #> 1390 pelvic_radius 98.62251165 #> 1391 pelvic_radius 94.01878339 #> 1392 pelvic_radius 125.3509625 #> 1393 pelvic_radius 111.9160075 #> 1394 pelvic_radius 116.7353868 #> 1395 pelvic_radius 103.3488802 #> 1396 pelvic_radius 82.45603817 #> 1397 pelvic_radius 118.5886691 #> 1398 pelvic_radius 108.6295666 #> 1399 pelvic_radius 103.0486975 #> 1400 pelvic_radius 95.25245421 #> 1401 pelvic_radius 115.72353 #> 1402 pelvic_radius 97.8010854 #> 1403 pelvic_radius 81.0245406 #> 1404 pelvic_radius 104.6986033 #> 1405 pelvic_radius 124.3978211 #> 1406 pelvic_radius 125.4801739 #> 1407 pelvic_radius 109.978045 #> 1408 pelvic_radius 70.08257486 #> 1409 pelvic_radius 89.3075466 #> 1410 pelvic_radius 98.27410705 #> 1411 pelvic_radius 111.679961 #> 1412 pelvic_radius 104.7312342 #> 1413 pelvic_radius 93.69220863 #> 1414 pelvic_radius 78.99945411 #> 1415 pelvic_radius 103.4045971 #> 1416 pelvic_radius 98.77711506 #> 1417 pelvic_radius 100.7154129 #> 1418 pelvic_radius 89.47183446 #> 1419 pelvic_radius 120.1034928 #> 1420 pelvic_radius 125.0185168 #> 1421 pelvic_radius 157.848799 #> 1422 pelvic_radius 90.298468 #> 1423 pelvic_radius 106.8295898 #> 1424 pelvic_radius 125.1642324 #> 1425 pelvic_radius 125.430176 #> 1426 pelvic_radius 117.3078968 #> 1427 pelvic_radius 90.07187999 #> 1428 pelvic_radius 109.0330745 #> 1429 pelvic_radius 120.8407069 #> 1430 pelvic_radius 117.0422439 #> 1431 pelvic_radius 88.43424213 #> 1432 pelvic_radius 97.4041888 #> 1433 pelvic_radius 146.4660009 #> 1434 pelvic_radius 119.2370072 #> 1435 pelvic_radius 116.9634162 #> 1436 pelvic_radius 110.703107 #> 1437 pelvic_radius 89.82274067 #> 1438 pelvic_radius 135.6294176 #> 1439 pelvic_radius 115.2087008 #> 1440 pelvic_radius 118.8833881 #> 1441 pelvic_radius 130.9992576 #> 1442 pelvic_radius 145.6010328 #> 1443 pelvic_radius 132.2672855 #> 1444 pelvic_radius 98.72792982 #> 1445 pelvic_radius 101.7233343 #> 1446 pelvic_radius 125.5936237 #> 1447 pelvic_radius 96.68390337 #> 1448 pelvic_radius 128.4057314 #> 1449 pelvic_radius 120.9448288 #> 1450 pelvic_radius 94.88233607 #> 1451 pelvic_radius 127.6328747 #> 1452 pelvic_radius 125.8466462 #> 1453 pelvic_radius 129.220682 #> 1454 pelvic_radius 128.9803079 #> 1455 pelvic_radius 130.1783144 #> 1456 pelvic_radius 142.4101072 #> 1457 pelvic_radius 118.151531 #> 1458 pelvic_radius 132.114805 #> 1459 pelvic_radius 129.385308 #> 1460 pelvic_radius 106.1777511 #> 1461 pelvic_radius 113.7784936 #> 1462 pelvic_radius 126.9703283 #> 1463 pelvic_radius 116.2285032 #> 1464 pelvic_radius 103.5825398 #> 1465 pelvic_radius 100.5011917 #> 1466 pelvic_radius 125.1742214 #> 1467 pelvic_radius 142.3601245 #> 1468 pelvic_radius 118.6862678 #> 1469 pelvic_radius 123.8034132 #> 1470 pelvic_radius 137.4396942 #> 1471 pelvic_radius 124.1280012 #> 1472 pelvic_radius 118.1930354 #> 1473 pelvic_radius 128.5177217 #> 1474 pelvic_radius 139.1184721 #> 1475 pelvic_radius 135.740926 #> 1476 pelvic_radius 113.0659387 #> 1477 pelvic_radius 121.779803 #> 1478 pelvic_radius 133.2818339 #> 1479 pelvic_radius 126.6116215 #> 1480 pelvic_radius 139.1896903 #> 1481 pelvic_radius 116.5551679 #> 1482 pelvic_radius 126.7185156 #> 1483 pelvic_radius 131.5844199 #> 1484 pelvic_radius 123.9877408 #> 1485 pelvic_radius 114.5066078 #> 1486 pelvic_radius 125.3911378 #> 1487 pelvic_radius 119.3776026 #> 1488 pelvic_radius 121.4355585 #> 1489 pelvic_radius 120.5769719 #> 1490 pelvic_radius 122.343516 #> 1491 pelvic_radius 135.9369096 #> 1492 pelvic_radius 139.2471502 #> 1493 pelvic_radius 121.6068586 #> 1494 pelvic_radius 119.5503909 #> 1495 pelvic_radius 111.2340468 #> 1496 pelvic_radius 123.6322597 #> 1497 pelvic_radius 123.0379985 #> 1498 pelvic_radius 131.8024914 #> 1499 pelvic_radius 128.0099272 #> 1500 pelvic_radius 117.0897469 #> 1501 pelvic_radius 122.2090834 #> 1502 pelvic_radius 105.6453997 #> 1503 pelvic_radius 128.9056892 #> 1504 pelvic_radius 128.3253556 #> 1505 pelvic_radius 111.7911722 #> 1506 pelvic_radius 135.6233101 #> 1507 pelvic_radius 121.2476572 #> 1508 pelvic_radius 123.0912395 #> 1509 pelvic_radius 113.8061775 #> 1510 pelvic_radius 125.0143609 #> 1511 pelvic_radius 126.4722584 #> 1512 pelvic_radius 120.631941 #> 1513 pelvic_radius 117.8187599 #> 1514 pelvic_radius 126.9239062 #> 1515 pelvic_radius 123.4674001 #> 1516 pelvic_radius 137.5917777 #> 1517 pelvic_radius 131.3061224 #> 1518 pelvic_radius 129.3935728 #> 1519 pelvic_radius 119.3356546 #> 1520 pelvic_radius 139.1504066 #> 1521 pelvic_radius 119.1346221 #> 1522 pelvic_radius 115.3882683 #> 1523 pelvic_radius 113.9137026 #> 1524 pelvic_radius 126.3981876 #> 1525 pelvic_radius 121.0356423 #> 1526 pelvic_radius 119.6856451 #> 1527 pelvic_radius 120.9201997 #> 1528 pelvic_radius 120.3886112 #> 1529 pelvic_radius 105.417304 #> 1530 pelvic_radius 131.7176127 #> 1531 pelvic_radius 126.0768612 #> 1532 pelvic_radius 115.8037111 #> 1533 pelvic_radius 127.1398495 #> 1534 pelvic_radius 137.1082886 #> 1535 pelvic_radius 128.0636203 #> 1536 pelvic_radius 130.3500956 #> 1537 pelvic_radius 131.922009 #> 1538 pelvic_radius 116.7970069 #> 1539 pelvic_radius 128.9029049 #> 1540 pelvic_radius 110.7089577 #> 1541 pelvic_radius 116.5879699 #> 1542 pelvic_radius 111.4810746 #> 1543 pelvic_radius 118.3433212 #> 1544 pelvic_radius 128.3001991 #> 1545 pelvic_radius 147.8946372 #> 1546 pelvic_radius 117.4490622 #> 1547 pelvic_radius 114.365845 #> 1548 pelvic_radius 125.6707246 #> 1549 pelvic_radius 118.5458418 #> 1550 pelvic_radius 123.9452436 #> 1551 degree_spondylolisthesis -0.254399986 #> 1552 degree_spondylolisthesis 4.564258645 #> 1553 degree_spondylolisthesis -3.530317314 #> 1554 degree_spondylolisthesis 11.21152344 #> 1555 degree_spondylolisthesis 7.918500615 #> 1556 degree_spondylolisthesis 2.230651729 #> 1557 degree_spondylolisthesis 5.988550702 #> 1558 degree_spondylolisthesis -10.67587083 #> 1559 degree_spondylolisthesis 13.28901817 #> 1560 degree_spondylolisthesis 0.664437117 #> 1561 degree_spondylolisthesis -7.825985755 #> 1562 degree_spondylolisthesis 0.499751446 #> 1563 degree_spondylolisthesis 8.028894629 #> 1564 degree_spondylolisthesis 7.044802938 #> 1565 degree_spondylolisthesis 5.766946943 #> 1566 degree_spondylolisthesis 5.415825143 #> 1567 degree_spondylolisthesis -0.622526643 #> 1568 degree_spondylolisthesis 3.623020073 #> 1569 degree_spondylolisthesis 1.429185758 #> 1570 degree_spondylolisthesis -1.244402488 #> 1571 degree_spondylolisthesis 6.451647637 #> 1572 degree_spondylolisthesis 2.432561437 #> 1573 degree_spondylolisthesis 15.77969683 #> 1574 degree_spondylolisthesis -4.987129618 #> 1575 degree_spondylolisthesis -3.237562489 #> 1576 degree_spondylolisthesis 1.571204816 #> 1577 degree_spondylolisthesis -10.09310817 #> 1578 degree_spondylolisthesis 8.992815727 #> 1579 degree_spondylolisthesis 2.652320636 #> 1580 degree_spondylolisthesis -2.005891748 #> 1581 degree_spondylolisthesis 10.86956554 #> 1582 degree_spondylolisthesis 6.386831648 #> 1583 degree_spondylolisthesis 5.569619587 #> 1584 degree_spondylolisthesis 2.703008052 #> 1585 degree_spondylolisthesis 1.474285836 #> 1586 degree_spondylolisthesis 4.393080498 #> 1587 degree_spondylolisthesis 6.413427708 #> 1588 degree_spondylolisthesis -0.263489651 #> 1589 degree_spondylolisthesis 2.812426855 #> 1590 degree_spondylolisthesis 1.694705102 #> 1591 degree_spondylolisthesis -3.460357991 #> 1592 degree_spondylolisthesis 2.045475795 #> 1593 degree_spondylolisthesis 5.329843204 #> 1594 degree_spondylolisthesis -0.799624469 #> 1595 degree_spondylolisthesis -2.325683841 #> 1596 degree_spondylolisthesis -2.526701511 #> 1597 degree_spondylolisthesis 6.481709096 #> 1598 degree_spondylolisthesis -4.497957556 #> 1599 degree_spondylolisthesis -1.537383074 #> 1600 degree_spondylolisthesis 2.062962549 #> 1601 degree_spondylolisthesis 3.558372358 #> 1602 degree_spondylolisthesis 5.000088788 #> 1603 degree_spondylolisthesis 5.740614083 #> 1604 degree_spondylolisthesis 11.46322327 #> 1605 degree_spondylolisthesis -9.569249858 #> 1606 degree_spondylolisthesis 6.273012173 #> 1607 degree_spondylolisthesis 5.753277458 #> 1608 degree_spondylolisthesis 1.662705589 #> 1609 degree_spondylolisthesis -2.919075955 #> 1610 degree_spondylolisthesis 7.947904861 #> 1611 degree_spondylolisthesis 56.12590603 #> 1612 degree_spondylolisthesis 92.02727682 #> 1613 degree_spondylolisthesis 29.10657504 #> 1614 degree_spondylolisthesis 26.93184095 #> 1615 degree_spondylolisthesis 10.43197194 #> 1616 degree_spondylolisthesis 26.58810016 #> 1617 degree_spondylolisthesis 27.38321314 #> 1618 degree_spondylolisthesis 69.55130292 #> 1619 degree_spondylolisthesis 1.01004051 #> 1620 degree_spondylolisthesis 28.05969711 #> 1621 degree_spondylolisthesis 32.10853735 #> 1622 degree_spondylolisthesis 101.7190919 #> 1623 degree_spondylolisthesis 74.33340864 #> 1624 degree_spondylolisthesis 34.55294641 #> 1625 degree_spondylolisthesis 56.80409277 #> 1626 degree_spondylolisthesis 145.3781432 #> 1627 degree_spondylolisthesis 110.8607824 #> 1628 degree_spondylolisthesis 51.58412476 #> 1629 degree_spondylolisthesis 33.15764573 #> 1630 degree_spondylolisthesis 21.68240136 #> 1631 degree_spondylolisthesis 70.75908308 #> 1632 degree_spondylolisthesis 74.55516588 #> 1633 degree_spondylolisthesis 51.80589921 #> 1634 degree_spondylolisthesis 65.21461611 #> 1635 degree_spondylolisthesis 50.12745689 #> 1636 degree_spondylolisthesis 20.31531532 #> 1637 degree_spondylolisthesis 22.12386874 #> 1638 degree_spondylolisthesis 37.36453993 #> 1639 degree_spondylolisthesis 24.0185747 #> 1640 degree_spondylolisthesis 27.28398451 #> 1641 degree_spondylolisthesis 30.60914842 #> 1642 degree_spondylolisthesis 32.522331 #> 1643 degree_spondylolisthesis 76.0206034 #> 1644 degree_spondylolisthesis 50.23787808 #> 1645 degree_spondylolisthesis 53.25522004 #> 1646 degree_spondylolisthesis 148.7537109 #> 1647 degree_spondylolisthesis 64.08099841 #> 1648 degree_spondylolisthesis 33.30606685 #> 1649 degree_spondylolisthesis 61.2111866 #> 1650 degree_spondylolisthesis 30.3879839 #> 1651 degree_spondylolisthesis 25.11847846 #> 1652 degree_spondylolisthesis 38.53874133 #> 1653 degree_spondylolisthesis 25.53842852 #> 1654 degree_spondylolisthesis 25.33564729 #> 1655 degree_spondylolisthesis 93.56373734 #> 1656 degree_spondylolisthesis 7.015977884 #> 1657 degree_spondylolisthesis 49.69695462 #> 1658 degree_spondylolisthesis 77.15517241 #> 1659 degree_spondylolisthesis 42.60807567 #> 1660 degree_spondylolisthesis 39.49798659 #> 1661 degree_spondylolisthesis 41.42282844 #> 1662 degree_spondylolisthesis 71.32117542 #> 1663 degree_spondylolisthesis 27.33867086 #> 1664 degree_spondylolisthesis 51.1696403 #> 1665 degree_spondylolisthesis 85.87215224 #> 1666 degree_spondylolisthesis 418.5430821 #> 1667 degree_spondylolisthesis 56.90244779 #> 1668 degree_spondylolisthesis 61.98827709 #> 1669 degree_spondylolisthesis 16.37808564 #> 1670 degree_spondylolisthesis 31.69354839 #> 1671 degree_spondylolisthesis 40.3823266 #> 1672 degree_spondylolisthesis 80.55560527 #> 1673 degree_spondylolisthesis 67.72731595 #> 1674 degree_spondylolisthesis 53.93202006 #> 1675 degree_spondylolisthesis 37.01708012 #> 1676 degree_spondylolisthesis 32.83587702 #> 1677 degree_spondylolisthesis 27.8101478 #> 1678 degree_spondylolisthesis 57.78125 #> 1679 degree_spondylolisthesis 58.82364821 #> 1680 degree_spondylolisthesis 39.7871542 #> 1681 degree_spondylolisthesis 26.76669655 #> 1682 degree_spondylolisthesis 17.91456046 #> 1683 degree_spondylolisthesis 54.81686729 #> 1684 degree_spondylolisthesis 55.50688907 #> 1685 degree_spondylolisthesis 54.93913416 #> 1686 degree_spondylolisthesis 82.09360704 #> 1687 degree_spondylolisthesis 56.76608323 #> 1688 degree_spondylolisthesis 49.67209559 #> 1689 degree_spondylolisthesis 56.42615873 #> 1690 degree_spondylolisthesis 38.03283108 #> 1691 degree_spondylolisthesis 29.70121083 #> 1692 degree_spondylolisthesis 118.3533701 #> 1693 degree_spondylolisthesis 58.88494802 #> 1694 degree_spondylolisthesis 104.8592474 #> 1695 degree_spondylolisthesis 30.40913315 #> 1696 degree_spondylolisthesis 42.88742577 #> 1697 degree_spondylolisthesis 68.37612182 #> 1698 degree_spondylolisthesis 31.77358318 #> 1699 degree_spondylolisthesis 39.30721246 #> 1700 degree_spondylolisthesis 36.7063954 #> 1701 degree_spondylolisthesis 40.51098228 #> 1702 degree_spondylolisthesis 35.00007784 #> 1703 degree_spondylolisthesis 31.78449499 #> 1704 degree_spondylolisthesis 30.34120327 #> 1705 degree_spondylolisthesis 27.66027669 #> 1706 degree_spondylolisthesis 41.6854736 #> 1707 degree_spondylolisthesis 61.70059824 #> 1708 degree_spondylolisthesis 42.81048066 #> 1709 degree_spondylolisthesis 52.16514503 #> 1710 degree_spondylolisthesis 39.40982612 #> 1711 degree_spondylolisthesis 58.05754155 #> 1712 degree_spondylolisthesis 42.13694325 #> 1713 degree_spondylolisthesis 74.04376736 #> 1714 degree_spondylolisthesis 81.19892712 #> 1715 degree_spondylolisthesis 25.0821266 #> 1716 degree_spondylolisthesis 69.279571 #> 1717 degree_spondylolisthesis 36.81011057 #> 1718 degree_spondylolisthesis 12.07264427 #> 1719 degree_spondylolisthesis 57.66084135 #> 1720 degree_spondylolisthesis 23.9106354 #> 1721 degree_spondylolisthesis 21.40719845 #> 1722 degree_spondylolisthesis 12.39285327 #> 1723 degree_spondylolisthesis 21.10812135 #> 1724 degree_spondylolisthesis 23.04152435 #> 1725 degree_spondylolisthesis 21.84340688 #> 1726 degree_spondylolisthesis 50.70187326 #> 1727 degree_spondylolisthesis 33.98913551 #> 1728 degree_spondylolisthesis 44.167602 #> 1729 degree_spondylolisthesis 52.46755185 #> 1730 degree_spondylolisthesis 54.69128928 #> 1731 degree_spondylolisthesis 33.60702661 #> 1732 degree_spondylolisthesis 31.32641123 #> 1733 degree_spondylolisthesis 54.96578902 #> 1734 degree_spondylolisthesis 67.77118983 #> 1735 degree_spondylolisthesis 38.18178176 #> 1736 degree_spondylolisthesis 52.62304673 #> 1737 degree_spondylolisthesis 49.159426 #> 1738 degree_spondylolisthesis 30.26578534 #> 1739 degree_spondylolisthesis 61.95903428 #> 1740 degree_spondylolisthesis 62.76534831 #> 1741 degree_spondylolisthesis 40.88092253 #> 1742 degree_spondylolisthesis 55.75222146 #> 1743 degree_spondylolisthesis 124.9844057 #> 1744 degree_spondylolisthesis 32.66650243 #> 1745 degree_spondylolisthesis 25.38424676 #> 1746 degree_spondylolisthesis 38.2598637 #> 1747 degree_spondylolisthesis 55.99545386 #> 1748 degree_spondylolisthesis 117.3146829 #> 1749 degree_spondylolisthesis 33.17225512 #> 1750 degree_spondylolisthesis 31.57582292 #> 1751 degree_spondylolisthesis 37.55670552 #> 1752 degree_spondylolisthesis 88.30148594 #> 1753 degree_spondylolisthesis 101.2187828 #> 1754 degree_spondylolisthesis 26.97578722 #> 1755 degree_spondylolisthesis 25.77317356 #> 1756 degree_spondylolisthesis 100.2921068 #> 1757 degree_spondylolisthesis 77.28307195 #> 1758 degree_spondylolisthesis 73.38821617 #> 1759 degree_spondylolisthesis 76.73062904 #> 1760 degree_spondylolisthesis 28.34379914 #> 1761 degree_spondylolisthesis 7.986683227 #> 1762 degree_spondylolisthesis 2.001642472 #> 1763 degree_spondylolisthesis 4.994195288 #> 1764 degree_spondylolisthesis -0.910940567 #> 1765 degree_spondylolisthesis -3.38890999 #> 1766 degree_spondylolisthesis -2.005372903 #> 1767 degree_spondylolisthesis -1.057985526 #> 1768 degree_spondylolisthesis 6.338199339 #> 1769 degree_spondylolisthesis 1.00922834 #> 1770 degree_spondylolisthesis 3.118221289 #> 1771 degree_spondylolisthesis -0.284129366 #> 1772 degree_spondylolisthesis -0.631602951 #> 1773 degree_spondylolisthesis 31.17276727 #> 1774 degree_spondylolisthesis -0.44366081 #> 1775 degree_spondylolisthesis 3.040973261 #> 1776 degree_spondylolisthesis 3.235159224 #> 1777 degree_spondylolisthesis 6.298970934 #> 1778 degree_spondylolisthesis 4.815031084 #> 1779 degree_spondylolisthesis 3.885773488 #> 1780 degree_spondylolisthesis -3.114450861 #> 1781 degree_spondylolisthesis -4.083298414 #> 1782 degree_spondylolisthesis 5.074353176 #> 1783 degree_spondylolisthesis 0.970926407 #> 1784 degree_spondylolisthesis -2.511618596 #> 1785 degree_spondylolisthesis 13.68304672 #> 1786 degree_spondylolisthesis -11.05817866 #> 1787 degree_spondylolisthesis 1.296191194 #> 1788 degree_spondylolisthesis 4.955105669 #> 1789 degree_spondylolisthesis 10.83201105 #> 1790 degree_spondylolisthesis -1.859688529 #> 1791 degree_spondylolisthesis -6.054537956 #> 1792 degree_spondylolisthesis 13.92833085 #> 1793 degree_spondylolisthesis -0.75946135 #> 1794 degree_spondylolisthesis -4.082937601 #> 1795 degree_spondylolisthesis 7.439869802 #> 1796 degree_spondylolisthesis -0.402523218 #> 1797 degree_spondylolisthesis 9.06458168 #> 1798 degree_spondylolisthesis 1.91330704 #> 1799 degree_spondylolisthesis 1.630663508 #> 1800 degree_spondylolisthesis 2.288487746 #> 1801 degree_spondylolisthesis -2.092506504 #> 1802 degree_spondylolisthesis 0.668556793 #> 1803 degree_spondylolisthesis -3.362044654 #> 1804 degree_spondylolisthesis 12.38260373 #> 1805 degree_spondylolisthesis 0.813491154 #> 1806 degree_spondylolisthesis 25.9702063 #> 1807 degree_spondylolisthesis 8.235294118 #> 1808 degree_spondylolisthesis -8.290203373 #> 1809 degree_spondylolisthesis 0.458901373 #> 1810 degree_spondylolisthesis -0.357811974 #> 1811 degree_spondylolisthesis 0.153549242 #> 1812 degree_spondylolisthesis 1.594747729 #> 1813 degree_spondylolisthesis 0.75702014 #> 1814 degree_spondylolisthesis -1.776111234 #> 1815 degree_spondylolisthesis -4.406769011 #> 1816 degree_spondylolisthesis 5.360050572 #> 1817 degree_spondylolisthesis -0.54202201 #> 1818 degree_spondylolisthesis 1.856659161 #> 1819 degree_spondylolisthesis 5.0151857 #> 1820 degree_spondylolisthesis 7.366425398 #> 1821 degree_spondylolisthesis -2.144043911 #> 1822 degree_spondylolisthesis 7.876730692 #> 1823 degree_spondylolisthesis 1.904048199 #> 1824 degree_spondylolisthesis -1.632238263 #> 1825 degree_spondylolisthesis 1.741017579 #> 1826 degree_spondylolisthesis 4.960343813 #> 1827 degree_spondylolisthesis 1.78886965 #> 1828 degree_spondylolisthesis -1.982120038 #> 1829 degree_spondylolisthesis -6.173674823 #> 1830 degree_spondylolisthesis 10.44286169 #> 1831 degree_spondylolisthesis 3.089484465 #> 1832 degree_spondylolisthesis 5.951454368 #> 1833 degree_spondylolisthesis 2.737035292 #> 1834 degree_spondylolisthesis 0.535471617 #> 1835 degree_spondylolisthesis 2.830504124 #> 1836 degree_spondylolisthesis 4.867539941 #> 1837 degree_spondylolisthesis 3.087725997 #> 1838 degree_spondylolisthesis 9.354364925 #> 1839 degree_spondylolisthesis 0.599247113 #> 1840 degree_spondylolisthesis -3.604255336 #> 1841 degree_spondylolisthesis 0.179717077 #> 1842 degree_spondylolisthesis 6.905089963 #> 1843 degree_spondylolisthesis -0.460894198 #> 1844 degree_spondylolisthesis 19.85475919 #> 1845 degree_spondylolisthesis -5.100053328 #> 1846 degree_spondylolisthesis 2.449382401 #> 1847 degree_spondylolisthesis -4.968979881 #> 1848 degree_spondylolisthesis 3.131909921 #> 1849 degree_spondylolisthesis 1.517203356 #> 1850 degree_spondylolisthesis 6.079337831 #> 1851 degree_spondylolisthesis -0.214710615 #> 1852 degree_spondylolisthesis 6.061508401 #> 1853 degree_spondylolisthesis -1.471067262 #> 1854 degree_spondylolisthesis -3.365515555 #> 1855 degree_spondylolisthesis -8.941709421 #> 1856 degree_spondylolisthesis -4.245395422 #> 1857 degree_spondylolisthesis -0.421010392 #> 1858 degree_spondylolisthesis -2.707879517 #> 1859 degree_spondylolisthesis 0.214750167 #> 1860 degree_spondylolisthesis -0.199249089 #> 1861 pelvic_slope 0.744503464 #> 1862 pelvic_slope 0.415185678 #> 1863 pelvic_slope 0.474889164 #> 1864 pelvic_slope 0.369345264 #> 1865 pelvic_slope 0.543360472 #> 1866 pelvic_slope 0.789992856 #> 1867 pelvic_slope 0.198919573 #> 1868 pelvic_slope 0.131972555 #> 1869 pelvic_slope 0.190407626 #> 1870 pelvic_slope 0.367700139 #> 1871 pelvic_slope 0.6880095 #> 1872 pelvic_slope 0.608342758 #> 1873 pelvic_slope 0.139478165 #> 1874 pelvic_slope 0.081930993 #> 1875 pelvic_slope 0.416721511 #> 1876 pelvic_slope 0.664040876 #> 1877 pelvic_slope 0.560675371 #> 1878 pelvic_slope 0.534481238 #> 1879 pelvic_slope 0.30658054 #> 1880 pelvic_slope 0.468525928 #> 1881 pelvic_slope 0.280446206 #> 1882 pelvic_slope 0.175244572 #> 1883 pelvic_slope 0.666388008 #> 1884 pelvic_slope 0.567450078 #> 1885 pelvic_slope 0.126473707 #> 1886 pelvic_slope 0.928687869 #> 1887 pelvic_slope 0.391971136 #> 1888 pelvic_slope 0.594175694 #> 1889 pelvic_slope 0.527891438 #> 1890 pelvic_slope 0.677267795 #> 1891 pelvic_slope 0.678987086 #> 1892 pelvic_slope 0.064872511 #> 1893 pelvic_slope 0.81674803 #> 1894 pelvic_slope 0.815941481 #> 1895 pelvic_slope 0.477087978 #> 1896 pelvic_slope 0.713153407 #> 1897 pelvic_slope 0.872719252 #> 1898 pelvic_slope 0.882807309 #> 1899 pelvic_slope 0.142325313 #> 1900 pelvic_slope 0.059106366 #> 1901 pelvic_slope 0.743812023 #> 1902 pelvic_slope 0.80691143 #> 1903 pelvic_slope 0.417637339 #> 1904 pelvic_slope 0.647625866 #> 1905 pelvic_slope 0.072901223 #> 1906 pelvic_slope 0.319204878 #> 1907 pelvic_slope 0.062276845 #> 1908 pelvic_slope 0.982250408 #> 1909 pelvic_slope 0.011971076 #> 1910 pelvic_slope 0.371350889 #> 1911 pelvic_slope 0.680654711 #> 1912 pelvic_slope 0.606767772 #> 1913 pelvic_slope 0.031139167 #> 1914 pelvic_slope 0.659772403 #> 1915 pelvic_slope 0.302179133 #> 1916 pelvic_slope 0.972555636 #> 1917 pelvic_slope 0.592034449 #> 1918 pelvic_slope 0.121465407 #> 1919 pelvic_slope 0.016747113 #> 1920 pelvic_slope 0.623275382 #> 1921 pelvic_slope 0.159377926 #> 1922 pelvic_slope 0.527584239 #> 1923 pelvic_slope 0.11662693 #> 1924 pelvic_slope 0.046225827 #> 1925 pelvic_slope 0.252796128 #> 1926 pelvic_slope 0.61476653 #> 1927 pelvic_slope 0.008485564 #> 1928 pelvic_slope 0.687091535 #> 1929 pelvic_slope 0.084621266 #> 1930 pelvic_slope 0.356359455 #> 1931 pelvic_slope 0.267291991 #> 1932 pelvic_slope 0.459674217 #> 1933 pelvic_slope 0.600115713 #> 1934 pelvic_slope 0.672041297 #> 1935 pelvic_slope 0.826532439 #> 1936 pelvic_slope 0.946610646 #> 1937 pelvic_slope 0.640487619 #> 1938 pelvic_slope 0.06039077 #> 1939 pelvic_slope 0.855643425 #> 1940 pelvic_slope 0.652596503 #> 1941 pelvic_slope 0.156502675 #> 1942 pelvic_slope 0.406965314 #> 1943 pelvic_slope 0.770613922 #> 1944 pelvic_slope 0.972005589 #> 1945 pelvic_slope 0.583098014 #> 1946 pelvic_slope 0.345234838 #> 1947 pelvic_slope 0.374705546 #> 1948 pelvic_slope 0.690088008 #> 1949 pelvic_slope 0.793168976 #> 1950 pelvic_slope 0.402241133 #> 1951 pelvic_slope 0.832811085 #> 1952 pelvic_slope 0.054842915 #> 1953 pelvic_slope 0.046938967 #> 1954 pelvic_slope 0.951361122 #> 1955 pelvic_slope 0.199874982 #> 1956 pelvic_slope 0.597457003 #> 1957 pelvic_slope 0.623388207 #> 1958 pelvic_slope 0.558833102 #> 1959 pelvic_slope 0.924902858 #> 1960 pelvic_slope 0.401084706 #> 1961 pelvic_slope 0.341664609 #> 1962 pelvic_slope 0.708886697 #> 1963 pelvic_slope 0.974400946 #> 1964 pelvic_slope 0.334657138 #> 1965 pelvic_slope 0.37528738 #> 1966 pelvic_slope 0.867324121 #> 1967 pelvic_slope 0.151993641 #> 1968 pelvic_slope 0.580604336 #> 1969 pelvic_slope 0.23433535 #> 1970 pelvic_slope 0.249350536 #> 1971 pelvic_slope 0.715779422 #> 1972 pelvic_slope 0.998826684 #> 1973 pelvic_slope 0.986271547 #> 1974 pelvic_slope 0.797662594 #> 1975 pelvic_slope 0.496180717 #> 1976 pelvic_slope 0.860222868 #> 1977 pelvic_slope 0.823630076 #> 1978 pelvic_slope 0.035210491 #> 1979 pelvic_slope 0.377683884 #> 1980 pelvic_slope 0.822344641 #> 1981 pelvic_slope 0.440722184 #> 1982 pelvic_slope 0.436932625 #> 1983 pelvic_slope 0.099940503 #> 1984 pelvic_slope 0.184438017 #> 1985 pelvic_slope 0.979253427 #> 1986 pelvic_slope 0.403799874 #> 1987 pelvic_slope 0.039655293 #> 1988 pelvic_slope 0.09580622 #> 1989 pelvic_slope 0.881441257 #> 1990 pelvic_slope 0.707245245 #> 1991 pelvic_slope 0.710450644 #> 1992 pelvic_slope 0.668562821 #> 1993 pelvic_slope 0.286894092 #> 1994 pelvic_slope 0.265889494 #> 1995 pelvic_slope 0.006128311 #> 1996 pelvic_slope 0.278327565 #> 1997 pelvic_slope 0.238984543 #> 1998 pelvic_slope 0.771860278 #> 1999 pelvic_slope 0.296152366 #> 2000 pelvic_slope 0.481861846 #> 2001 pelvic_slope 0.003220264 #> 2002 pelvic_slope 0.039380359 #> 2003 pelvic_slope 0.487939752 #> 2004 pelvic_slope 0.386903046 #> 2005 pelvic_slope 0.41469298 #> 2006 pelvic_slope 0.844294085 #> 2007 pelvic_slope 0.624974835 #> 2008 pelvic_slope 0.651920192 #> 2009 pelvic_slope 0.081033285 #> 2010 pelvic_slope 0.672569597 #> 2011 pelvic_slope 0.530804498 #> 2012 pelvic_slope 0.820727828 #> 2013 pelvic_slope 0.113089671 #> 2014 pelvic_slope 0.431125462 #> 2015 pelvic_slope 0.044130317 #> 2016 pelvic_slope 0.738028916 #> 2017 pelvic_slope 0.642084751 #> 2018 pelvic_slope 0.480821979 #> 2019 pelvic_slope 0.561323329 #> 2020 pelvic_slope 0.352038125 #> 2021 pelvic_slope 0.302441921 #> 2022 pelvic_slope 0.936622832 #> 2023 pelvic_slope 0.599392845 #> 2024 pelvic_slope 0.542816002 #> 2025 pelvic_slope 0.871551213 #> 2026 pelvic_slope 0.73595883 #> 2027 pelvic_slope 0.555518055 #> 2028 pelvic_slope 0.056944145 #> 2029 pelvic_slope 0.268276345 #> 2030 pelvic_slope 0.180863626 #> 2031 pelvic_slope 0.303206157 #> 2032 pelvic_slope 0.842278621 #> 2033 pelvic_slope 0.524781111 #> 2034 pelvic_slope 0.051929588 #> 2035 pelvic_slope 0.697750246 #> 2036 pelvic_slope 0.588947646 #> 2037 pelvic_slope 0.053300996 #> 2038 pelvic_slope 0.080967648 #> 2039 pelvic_slope 0.93160362 #> 2040 pelvic_slope 0.3798954 #> 2041 pelvic_slope 0.473130085 #> 2042 pelvic_slope 0.781685896 #> 2043 pelvic_slope 0.030341261 #> 2044 pelvic_slope 0.60269836 #> 2045 pelvic_slope 0.849081982 #> 2046 pelvic_slope 0.560009753 #> 2047 pelvic_slope 0.817563954 #> 2048 pelvic_slope 0.916504638 #> 2049 pelvic_slope 0.807918832 #> 2050 pelvic_slope 0.418463519 #> 2051 pelvic_slope 0.81109806 #> 2052 pelvic_slope 0.273687068 #> 2053 pelvic_slope 0.059381409 #> 2054 pelvic_slope 0.163594906 #> 2055 pelvic_slope 0.438382061 #> 2056 pelvic_slope 0.506379066 #> 2057 pelvic_slope 0.276928366 #> 2058 pelvic_slope 0.751482204 #> 2059 pelvic_slope 0.82191484 #> 2060 pelvic_slope 0.677506366 #> 2061 pelvic_slope 0.659016993 #> 2062 pelvic_slope 0.575212433 #> 2063 pelvic_slope 0.218938743 #> 2064 pelvic_slope 0.198909407 #> 2065 pelvic_slope 0.052601671 #> 2066 pelvic_slope 0.088561249 #> 2067 pelvic_slope 0.778847512 #> 2068 pelvic_slope 0.910885747 #> 2069 pelvic_slope 0.57477539 #> 2070 pelvic_slope 0.388444626 #> 2071 pelvic_slope 0.396363819 #> 2072 pelvic_slope 0.106174649 #> 2073 pelvic_slope 0.537574087 #> 2074 pelvic_slope 0.744321935 #> 2075 pelvic_slope 0.990034481 #> 2076 pelvic_slope 0.326835429 #> 2077 pelvic_slope 0.038359424 #> 2078 pelvic_slope 0.023094566 #> 2079 pelvic_slope 0.00504538 #> 2080 pelvic_slope 0.379709586 #> 2081 pelvic_slope 0.547080375 #> 2082 pelvic_slope 0.777717236 #> 2083 pelvic_slope 0.713860571 #> 2084 pelvic_slope 0.647608649 #> 2085 pelvic_slope 0.379932692 #> 2086 pelvic_slope 0.08021879 #> 2087 pelvic_slope 0.134954068 #> 2088 pelvic_slope 0.212129265 #> 2089 pelvic_slope 0.933377696 #> 2090 pelvic_slope 0.283588696 #> 2091 pelvic_slope 0.997247491 #> 2092 pelvic_slope 0.146745173 #> 2093 pelvic_slope 0.110795865 #> 2094 pelvic_slope 0.775688024 #> 2095 pelvic_slope 0.465169721 #> 2096 pelvic_slope 0.412296214 #> 2097 pelvic_slope 0.629660667 #> 2098 pelvic_slope 0.122419736 #> 2099 pelvic_slope 0.385073208 #> 2100 pelvic_slope 0.076818991 #> 2101 pelvic_slope 0.098119047 #> 2102 pelvic_slope 0.863545131 #> 2103 pelvic_slope 0.252511739 #> 2104 pelvic_slope 0.419743489 #> 2105 pelvic_slope 0.577847158 #> 2106 pelvic_slope 0.750423593 #> 2107 pelvic_slope 0.478970567 #> 2108 pelvic_slope 0.487833677 #> 2109 pelvic_slope 0.634019655 #> 2110 pelvic_slope 0.147745134 #> 2111 pelvic_slope 0.332027235 #> 2112 pelvic_slope 0.277170683 #> 2113 pelvic_slope 0.542970928 #> 2114 pelvic_slope 0.607879237 #> 2115 pelvic_slope 0.409585847 #> 2116 pelvic_slope 0.330438834 #> 2117 pelvic_slope 0.385732443 #> 2118 pelvic_slope 0.308798826 #> 2119 pelvic_slope 0.170983426 #> 2120 pelvic_slope 0.9539319 #> 2121 pelvic_slope 0.354894839 #> 2122 pelvic_slope 0.318966687 #> 2123 pelvic_slope 0.625180876 #> 2124 pelvic_slope 0.389858261 #> 2125 pelvic_slope 0.509022104 #> 2126 pelvic_slope 0.859959636 #> 2127 pelvic_slope 0.95599665 #> 2128 pelvic_slope 0.226819134 #> 2129 pelvic_slope 0.223549676 #> 2130 pelvic_slope 0.851140631 #> 2131 pelvic_slope 0.46741327 #> 2132 pelvic_slope 0.040763821 #> 2133 pelvic_slope 0.58775384 #> 2134 pelvic_slope 0.793121083 #> 2135 pelvic_slope 0.300086307 #> 2136 pelvic_slope 0.233195482 #> 2137 pelvic_slope 0.417720656 #> 2138 pelvic_slope 0.72753665 #> 2139 pelvic_slope 0.456246663 #> 2140 pelvic_slope 0.10501352 #> 2141 pelvic_slope 0.744332895 #> 2142 pelvic_slope 0.033702747 #> 2143 pelvic_slope 0.702208022 #> 2144 pelvic_slope 0.732730192 #> 2145 pelvic_slope 0.693905676 #> 2146 pelvic_slope 0.03615827 #> 2147 pelvic_slope 0.455056082 #> 2148 pelvic_slope 0.167308823 #> 2149 pelvic_slope 0.117780016 #> 2150 pelvic_slope 0.126792054 #> 2151 pelvic_slope 0.686409465 #> 2152 pelvic_slope 0.705725568 #> 2153 pelvic_slope 0.281611979 #> 2154 pelvic_slope 0.215175297 #> 2155 pelvic_slope 0.860783838 #> 2156 pelvic_slope 0.515439224 #> 2157 pelvic_slope 0.794716755 #> 2158 pelvic_slope 0.514212483 #> 2159 pelvic_slope 0.787252431 #> 2160 pelvic_slope 0.827146263 #> 2161 pelvic_slope 0.021178285 #> 2162 pelvic_slope 0.544504889 #> 2163 pelvic_slope 0.962907494 #> 2164 pelvic_slope 0.581168695 #> 2165 pelvic_slope 0.932921843 #> 2166 pelvic_slope 0.129744031 #> 2167 pelvic_slope 0.047913469 #> 2168 pelvic_slope 0.081070436 #> 2169 pelvic_slope 0.159250601 #> 2170 pelvic_slope 0.674504089 #> 2171 direct_tilt 12.5661 #> 2172 direct_tilt 12.8874 #> 2173 direct_tilt 26.8343 #> 2174 direct_tilt 23.5603 #> 2175 direct_tilt 35.494 #> 2176 direct_tilt 29.323 #> 2177 direct_tilt 13.8514 #> 2178 direct_tilt 28.8165 #> 2179 direct_tilt 22.7085 #> 2180 direct_tilt 26.2011 #> 2181 direct_tilt 31.3502 #> 2182 direct_tilt 21.4356 #> 2183 direct_tilt 32.7916 #> 2184 direct_tilt 15.058 #> 2185 direct_tilt 16.5158 #> 2186 direct_tilt 9.5021 #> 2187 direct_tilt 10.769 #> 2188 direct_tilt 31.1641 #> 2189 direct_tilt 28.3015 #> 2190 direct_tilt 28.5598 #> 2191 direct_tilt 12.4719 #> 2192 direct_tilt 23.0791 #> 2193 direct_tilt 11.9696 #> 2194 direct_tilt 23.8889 #> 2195 direct_tilt 25.6206 #> 2196 direct_tilt 14.6686 #> 2197 direct_tilt 9.871 #> 2198 direct_tilt 30.4577 #> 2199 direct_tilt 32.4275 #> 2200 direct_tilt 12.4271 #> 2201 direct_tilt 7.1103 #> 2202 direct_tilt 14.2826 #> 2203 direct_tilt 27.5218 #> 2204 direct_tilt 30.2045 #> 2205 direct_tilt 8.6051 #> 2206 direct_tilt 9.7107 #> 2207 direct_tilt 24.4626 #> 2208 direct_tilt 32.5864 #> 2209 direct_tilt 12.6634 #> 2210 direct_tilt 30.0191 #> 2211 direct_tilt 15.3987 #> 2212 direct_tilt 27.8754 #> 2213 direct_tilt 9.3514 #> 2214 direct_tilt 9.0466 #> 2215 direct_tilt 24.861 #> 2216 direct_tilt 30.6389 #> 2217 direct_tilt 23.5538 #> 2218 direct_tilt 29.2557 #> 2219 direct_tilt 31.6694 #> 2220 direct_tilt 21.1136 #> 2221 direct_tilt 16.711 #> 2222 direct_tilt 32.6283 #> 2223 direct_tilt 36.1431 #> 2224 direct_tilt 12.0969 #> 2225 direct_tilt 9.2518 #> 2226 direct_tilt 18.2046 #> 2227 direct_tilt 14.3377 #> 2228 direct_tilt 30.283 #> 2229 direct_tilt 26.8235 #> 2230 direct_tilt 33.5947 #> 2231 direct_tilt 35.9529 #> 2232 direct_tilt 26.3756 #> 2233 direct_tilt 22.0548 #> 2234 direct_tilt 24.5548 #> 2235 direct_tilt 21.5934 #> 2236 direct_tilt 8.8345 #> 2237 direct_tilt 7.5647 #> 2238 direct_tilt 24.1004 #> 2239 direct_tilt 12.6031 #> 2240 direct_tilt 11.4299 #> 2241 direct_tilt 26.9716 #> 2242 direct_tilt 25.0986 #> 2243 direct_tilt 25.6364 #> 2244 direct_tilt 35.0909 #> 2245 direct_tilt 32.3379 #> 2246 direct_tilt 10.384 #> 2247 direct_tilt 16.5571 #> 2248 direct_tilt 26.0645 #> 2249 direct_tilt 18.9972 #> 2250 direct_tilt 23.2067 #> 2251 direct_tilt 10.849 #> 2252 direct_tilt 10.5895 #> 2253 direct_tilt 13.1962 #> 2254 direct_tilt 10.5715 #> 2255 direct_tilt 33.1635 #> 2256 direct_tilt 31.8795 #> 2257 direct_tilt 23.3173 #> 2258 direct_tilt 18.0873 #> 2259 direct_tilt 19.5456 #> 2260 direct_tilt 9.1296 #> 2261 direct_tilt 23.1811 #> 2262 direct_tilt 7.3173 #> 2263 direct_tilt 26.0497 #> 2264 direct_tilt 33.904 #> 2265 direct_tilt 12.1048 #> 2266 direct_tilt 21.5943 #> 2267 direct_tilt 21.5013 #> 2268 direct_tilt 7.027 #> 2269 direct_tilt 14.9502 #> 2270 direct_tilt 34.6931 #> 2271 direct_tilt 30.4108 #> 2272 direct_tilt 11.2935 #> 2273 direct_tilt 30.9297 #> 2274 direct_tilt 17.6515 #> 2275 direct_tilt 11.2385 #> 2276 direct_tilt 12.1292 #> 2277 direct_tilt 14.3986 #> 2278 direct_tilt 36.6285 #> 2279 direct_tilt 24.5629 #> 2280 direct_tilt 27.0646 #> 2281 direct_tilt 24.8714 #> 2282 direct_tilt 7.0551 #> 2283 direct_tilt 15.6365 #> 2284 direct_tilt 9.6196 #> 2285 direct_tilt 27.5223 #> 2286 direct_tilt 18.5943 #> 2287 direct_tilt 13.5572 #> 2288 direct_tilt 27.5499 #> 2289 direct_tilt 25.067 #> 2290 direct_tilt 26.2469 #> 2291 direct_tilt 30.0719 #> 2292 direct_tilt 7.2994 #> 2293 direct_tilt 20.2822 #> 2294 direct_tilt 20.2044 #> 2295 direct_tilt 25.3481 #> 2296 direct_tilt 31.1599 #> 2297 direct_tilt 15.7748 #> 2298 direct_tilt 26.778 #> 2299 direct_tilt 13.5739 #> 2300 direct_tilt 22.5858 #> 2301 direct_tilt 21.9074 #> 2302 direct_tilt 24.2342 #> 2303 direct_tilt 18.5916 #> 2304 direct_tilt 15.379 #> 2305 direct_tilt 20.3617 #> 2306 direct_tilt 21.9069 #> 2307 direct_tilt 14.6182 #> 2308 direct_tilt 24.9264 #> 2309 direct_tilt 7.7545 #> 2310 direct_tilt 17.1681 #> 2311 direct_tilt 13.3594 #> 2312 direct_tilt 19.8712 #> 2313 direct_tilt 9.4271 #> 2314 direct_tilt 17.0217 #> 2315 direct_tilt 17.6829 #> 2316 direct_tilt 16.9272 #> 2317 direct_tilt 26.5001 #> 2318 direct_tilt 36.7439 #> 2319 direct_tilt 35.6242 #> 2320 direct_tilt 29.0324 #> 2321 direct_tilt 11.4265 #> 2322 direct_tilt 22.6313 #> 2323 direct_tilt 20.9044 #> 2324 direct_tilt 22.253 #> 2325 direct_tilt 21.0757 #> 2326 direct_tilt 24.6372 #> 2327 direct_tilt 8.2975 #> 2328 direct_tilt 20.0603 #> 2329 direct_tilt 35.4921 #> 2330 direct_tilt 36.3579 #> 2331 direct_tilt 30.0162 #> 2332 direct_tilt 17.7459 #> 2333 direct_tilt 35.8563 #> 2334 direct_tilt 22.3317 #> 2335 direct_tilt 17.5525 #> 2336 direct_tilt 33.8124 #> 2337 direct_tilt 33.3025 #> 2338 direct_tilt 31.823 #> 2339 direct_tilt 28.6901 #> 2340 direct_tilt 9.971 #> 2341 direct_tilt 25.2382 #> 2342 direct_tilt 29.9807 #> 2343 direct_tilt 8.0015 #> 2344 direct_tilt 29.3962 #> 2345 direct_tilt 8.4084 #> 2346 direct_tilt 18.1451 #> 2347 direct_tilt 8.5072 #> 2348 direct_tilt 23.0765 #> 2349 direct_tilt 20.0845 #> 2350 direct_tilt 17.4281 #> 2351 direct_tilt 24.8271 #> 2352 direct_tilt 24.7626 #> 2353 direct_tilt 8.763 #> 2354 direct_tilt 10.1025 #> 2355 direct_tilt 11.0201 #> 2356 direct_tilt 13.9263 #> 2357 direct_tilt 16.7111 #> 2358 direct_tilt 35.031 #> 2359 direct_tilt 24.8883 #> 2360 direct_tilt 29.7716 #> 2361 direct_tilt 10.6583 #> 2362 direct_tilt 10.1834 #> 2363 direct_tilt 33.708 #> 2364 direct_tilt 9.0344 #> 2365 direct_tilt 13.0121 #> 2366 direct_tilt 23.0527 #> 2367 direct_tilt 24.5529 #> 2368 direct_tilt 12.6576 #> 2369 direct_tilt 20.4667 #> 2370 direct_tilt 10.1345 #> 2371 direct_tilt 34.2436 #> 2372 direct_tilt 9.1217 #> 2373 direct_tilt 31.5204 #> 2374 direct_tilt 30.8752 #> 2375 direct_tilt 31.0079 #> 2376 direct_tilt 11.6503 #> 2377 direct_tilt 29.838 #> 2378 direct_tilt 13.1813 #> 2379 direct_tilt 23.8665 #> 2380 direct_tilt 16.1775 #> 2381 direct_tilt 34.8106 #> 2382 direct_tilt 7.3907 #> 2383 direct_tilt 33.0601 #> 2384 direct_tilt 36.6194 #> 2385 direct_tilt 26.6333 #> 2386 direct_tilt 16.5218 #> 2387 direct_tilt 35.6096 #> 2388 direct_tilt 19.7932 #> 2389 direct_tilt 32.42 #> 2390 direct_tilt 7.9787 #> 2391 direct_tilt 23.9247 #> 2392 direct_tilt 16.7774 #> 2393 direct_tilt 9.1686 #> 2394 direct_tilt 29.0187 #> 2395 direct_tilt 9.4868 #> 2396 direct_tilt 26.2021 #> 2397 direct_tilt 31.7659 #> 2398 direct_tilt 14.4911 #> 2399 direct_tilt 14.7528 #> 2400 direct_tilt 36.0097 #> 2401 direct_tilt 30.0422 #> 2402 direct_tilt 17.7116 #> 2403 direct_tilt 8.9802 #> 2404 direct_tilt 31.2682 #> 2405 direct_tilt 28.9703 #> 2406 direct_tilt 19.7733 #> 2407 direct_tilt 17.9906 #> 2408 direct_tilt 33.8766 #> 2409 direct_tilt 35.4534 #> 2410 direct_tilt 11.2853 #> 2411 direct_tilt 10.0549 #> 2412 direct_tilt 33.2628 #> 2413 direct_tilt 26.2684 #> 2414 direct_tilt 29.72 #> 2415 direct_tilt 11.2203 #> 2416 direct_tilt 32.8112 #> 2417 direct_tilt 23.5284 #> 2418 direct_tilt 30.4187 #> 2419 direct_tilt 27.8268 #> 2420 direct_tilt 28.9054 #> 2421 direct_tilt 10.4752 #> 2422 direct_tilt 11.196 #> 2423 direct_tilt 12.4697 #> 2424 direct_tilt 21.5605 #> 2425 direct_tilt 25.4781 #> 2426 direct_tilt 8.351 #> 2427 direct_tilt 28.1386 #> 2428 direct_tilt 17.9008 #> 2429 direct_tilt 12.8223 #> 2430 direct_tilt 15.5713 #> 2431 direct_tilt 22.1191 #> 2432 direct_tilt 13.2564 #> 2433 direct_tilt 35.6342 #> 2434 direct_tilt 9.673 #> 2435 direct_tilt 28.2247 #> 2436 direct_tilt 21.7568 #> 2437 direct_tilt 34.9209 #> 2438 direct_tilt 21.8843 #> 2439 direct_tilt 25.7485 #> 2440 direct_tilt 10.7294 #> 2441 direct_tilt 22.7101 #> 2442 direct_tilt 16.7193 #> 2443 direct_tilt 29.1291 #> 2444 direct_tilt 10.7594 #> 2445 direct_tilt 12.799 #> 2446 direct_tilt 23.3207 #> 2447 direct_tilt 29.8852 #> 2448 direct_tilt 33.264 #> 2449 direct_tilt 27.1984 #> 2450 direct_tilt 26.1245 #> 2451 direct_tilt 31.9991 #> 2452 direct_tilt 32.2615 #> 2453 direct_tilt 24.7795 #> 2454 direct_tilt 33.7477 #> 2455 direct_tilt 24.8746 #> 2456 direct_tilt 18.4894 #> 2457 direct_tilt 8.866 #> 2458 direct_tilt 17.196 #> 2459 direct_tilt 18.0547 #> 2460 direct_tilt 15.1269 #> 2461 direct_tilt 8.3909 #> 2462 direct_tilt 24.1378 #> 2463 direct_tilt 24.1257 #> 2464 direct_tilt 29.8825 #> 2465 direct_tilt 9.5912 #> 2466 direct_tilt 9.1955 #> 2467 direct_tilt 31.3737 #> 2468 direct_tilt 24.2526 #> 2469 direct_tilt 12.8877 #> 2470 direct_tilt 12.5622 #> 2471 direct_tilt 18.7846 #> 2472 direct_tilt 27.0219 #> 2473 direct_tilt 30.8554 #> 2474 direct_tilt 12.0774 #> 2475 direct_tilt 32.1169 #> 2476 direct_tilt 7.8433 #> 2477 direct_tilt 19.1986 #> 2478 direct_tilt 16.2059 #> 2479 direct_tilt 14.7334 #> 2480 direct_tilt 19.3825 #> 2481 thoracic_slope 14.5386 #> 2482 thoracic_slope 17.5323 #> 2483 thoracic_slope 17.4861 #> 2484 thoracic_slope 12.7074 #> 2485 thoracic_slope 15.9546 #> 2486 thoracic_slope 12.0036 #> 2487 thoracic_slope 10.7146 #> 2488 thoracic_slope 7.7676 #> 2489 thoracic_slope 11.4234 #> 2490 thoracic_slope 8.738 #> 2491 thoracic_slope 16.5097 #> 2492 thoracic_slope 9.2589 #> 2493 thoracic_slope 7.2049 #> 2494 thoracic_slope 12.8127 #> 2495 thoracic_slope 18.6222 #> 2496 thoracic_slope 19.1756 #> 2497 thoracic_slope 16.8116 #> 2498 thoracic_slope 18.6089 #> 2499 thoracic_slope 17.9575 #> 2500 thoracic_slope 12.4637 #> 2501 thoracic_slope 16.8965 #> 2502 thoracic_slope 14.2195 #> 2503 thoracic_slope 17.6891 #> 2504 thoracic_slope 9.1019 #> 2505 thoracic_slope 15.7438 #> 2506 thoracic_slope 13.57 #> 2507 thoracic_slope 8.6406 #> 2508 thoracic_slope 17.97 #> 2509 thoracic_slope 10.2244 #> 2510 thoracic_slope 8.2495 #> 2511 thoracic_slope 7.2481 #> 2512 thoracic_slope 7.4515 #> 2513 thoracic_slope 13.8357 #> 2514 thoracic_slope 7.5284 #> 2515 thoracic_slope 8.3058 #> 2516 thoracic_slope 8.1003 #> 2517 thoracic_slope 11.6395 #> 2518 thoracic_slope 12.7274 #> 2519 thoracic_slope 8.855 #> 2520 thoracic_slope 18.9113 #> 2521 thoracic_slope 9.3239 #> 2522 thoracic_slope 12.2285 #> 2523 thoracic_slope 11.5243 #> 2524 thoracic_slope 10.2636 #> 2525 thoracic_slope 12.2345 #> 2526 thoracic_slope 18.6181 #> 2527 thoracic_slope 11.0942 #> 2528 thoracic_slope 16.7065 #> 2529 thoracic_slope 8.2526 #> 2530 thoracic_slope 7.1646 #> 2531 thoracic_slope 15.9714 #> 2532 thoracic_slope 9.8062 #> 2533 thoracic_slope 13.9907 #> 2534 thoracic_slope 10.7071 #> 2535 thoracic_slope 12.8432 #> 2536 thoracic_slope 16.9817 #> 2537 thoracic_slope 14.2176 #> 2538 thoracic_slope 14.6233 #> 2539 thoracic_slope 16.2905 #> 2540 thoracic_slope 14.1761 #> 2541 thoracic_slope 15.3975 #> 2542 thoracic_slope 18.6012 #> 2543 thoracic_slope 13.7595 #> 2544 thoracic_slope 15.3209 #> 2545 thoracic_slope 7.8098 #> 2546 thoracic_slope 7.2405 #> 2547 thoracic_slope 12.6737 #> 2548 thoracic_slope 13.3444 #> 2549 thoracic_slope 9.6504 #> 2550 thoracic_slope 15.3124 #> 2551 thoracic_slope 18.3111 #> 2552 thoracic_slope 8.7655 #> 2553 thoracic_slope 17.7501 #> 2554 thoracic_slope 8.6569 #> 2555 thoracic_slope 7.4437 #> 2556 thoracic_slope 9.5742 #> 2557 thoracic_slope 17.3635 #> 2558 thoracic_slope 11.7696 #> 2559 thoracic_slope 12.8779 #> 2560 thoracic_slope 14.7577 #> 2561 thoracic_slope 13.2216 #> 2562 thoracic_slope 12.5946 #> 2563 thoracic_slope 13.659 #> 2564 thoracic_slope 11.2339 #> 2565 thoracic_slope 8.383 #> 2566 thoracic_slope 15.0011 #> 2567 thoracic_slope 15.0648 #> 2568 thoracic_slope 18.1846 #> 2569 thoracic_slope 8.7779 #> 2570 thoracic_slope 16.7172 #> 2571 thoracic_slope 11.2491 #> 2572 thoracic_slope 16.3676 #> 2573 thoracic_slope 11.7878 #> 2574 thoracic_slope 11.6354 #> 2575 thoracic_slope 19.1837 #> 2576 thoracic_slope 7.5666 #> 2577 thoracic_slope 13.0551 #> 2578 thoracic_slope 7.8697 #> 2579 thoracic_slope 15.0493 #> 2580 thoracic_slope 10.3564 #> 2581 thoracic_slope 15.7092 #> 2582 thoracic_slope 10.922 #> 2583 thoracic_slope 9.5431 #> 2584 thoracic_slope 11.704 #> 2585 thoracic_slope 12.9197 #> 2586 thoracic_slope 13.8536 #> 2587 thoracic_slope 8.9024 #> 2588 thoracic_slope 16.6264 #> 2589 thoracic_slope 11.073 #> 2590 thoracic_slope 17.7171 #> 2591 thoracic_slope 16.645 #> 2592 thoracic_slope 9.0119 #> 2593 thoracic_slope 10.8504 #> 2594 thoracic_slope 17.4517 #> 2595 thoracic_slope 13.5136 #> 2596 thoracic_slope 11.1514 #> 2597 thoracic_slope 10.4727 #> 2598 thoracic_slope 12.008 #> 2599 thoracic_slope 13.7801 #> 2600 thoracic_slope 17.3268 #> 2601 thoracic_slope 19.2053 #> 2602 thoracic_slope 11.1917 #> 2603 thoracic_slope 10.3082 #> 2604 thoracic_slope 11.528 #> 2605 thoracic_slope 8.2316 #> 2606 thoracic_slope 11.5825 #> 2607 thoracic_slope 14.8568 #> 2608 thoracic_slope 18.2886 #> 2609 thoracic_slope 16.3289 #> 2610 thoracic_slope 12.5804 #> 2611 thoracic_slope 18.8412 #> 2612 thoracic_slope 8.7164 #> 2613 thoracic_slope 15.4963 #> 2614 thoracic_slope 18.1885 #> 2615 thoracic_slope 8.5783 #> 2616 thoracic_slope 17.0071 #> 2617 thoracic_slope 12.1692 #> 2618 thoracic_slope 7.6245 #> 2619 thoracic_slope 10.8824 #> 2620 thoracic_slope 8.4727 #> 2621 thoracic_slope 18.2659 #> 2622 thoracic_slope 8.9861 #> 2623 thoracic_slope 12.5462 #> 2624 thoracic_slope 8.8097 #> 2625 thoracic_slope 16.5256 #> 2626 thoracic_slope 8.0109 #> 2627 thoracic_slope 11.2204 #> 2628 thoracic_slope 9.0278 #> 2629 thoracic_slope 7.4483 #> 2630 thoracic_slope 14.5804 #> 2631 thoracic_slope 8.3428 #> 2632 thoracic_slope 14.8226 #> 2633 thoracic_slope 13.1978 #> 2634 thoracic_slope 17.6755 #> 2635 thoracic_slope 10.5374 #> 2636 thoracic_slope 12.5023 #> 2637 thoracic_slope 8.384 #> 2638 thoracic_slope 14.7325 #> 2639 thoracic_slope 14.1033 #> 2640 thoracic_slope 7.0378 #> 2641 thoracic_slope 9.8318 #> 2642 thoracic_slope 9.6738 #> 2643 thoracic_slope 10.9266 #> 2644 thoracic_slope 8.8519 #> 2645 thoracic_slope 17.5404 #> 2646 thoracic_slope 15.1125 #> 2647 thoracic_slope 18.5683 #> 2648 thoracic_slope 15.7134 #> 2649 thoracic_slope 7.2124 #> 2650 thoracic_slope 12.4275 #> 2651 thoracic_slope 7.175 #> 2652 thoracic_slope 7.1135 #> 2653 thoracic_slope 18.1719 #> 2654 thoracic_slope 16.3725 #> 2655 thoracic_slope 15.698 #> 2656 thoracic_slope 13.9634 #> 2657 thoracic_slope 13.34 #> 2658 thoracic_slope 12.6822 #> 2659 thoracic_slope 18.4914 #> 2660 thoracic_slope 16.0009 #> 2661 thoracic_slope 17.5433 #> 2662 thoracic_slope 18.5975 #> 2663 thoracic_slope 11.0399 #> 2664 thoracic_slope 14.5544 #> 2665 thoracic_slope 10.3301 #> 2666 thoracic_slope 12.1023 #> 2667 thoracic_slope 13.4438 #> 2668 thoracic_slope 18.2437 #> 2669 thoracic_slope 13.238 #> 2670 thoracic_slope 16.189 #> 2671 thoracic_slope 16.9317 #> 2672 thoracic_slope 10.416 #> 2673 thoracic_slope 12.3988 #> 2674 thoracic_slope 11.9015 #> 2675 thoracic_slope 14.5781 #> 2676 thoracic_slope 15.243 #> 2677 thoracic_slope 10.4232 #> 2678 thoracic_slope 15.9381 #> 2679 thoracic_slope 9.351 #> 2680 thoracic_slope 12.2607 #> 2681 thoracic_slope 10.1943 #> 2682 thoracic_slope 19.324 #> 2683 thoracic_slope 13.6555 #> 2684 thoracic_slope 9.4553 #> 2685 thoracic_slope 14.4887 #> 2686 thoracic_slope 16.1451 #> 2687 thoracic_slope 14.5939 #> 2688 thoracic_slope 10.1368 #> 2689 thoracic_slope 13.0473 #> 2690 thoracic_slope 15.0636 #> 2691 thoracic_slope 12.7802 #> 2692 thoracic_slope 11.3014 #> 2693 thoracic_slope 7.808 #> 2694 thoracic_slope 14.635 #> 2695 thoracic_slope 18.3694 #> 2696 thoracic_slope 18.0205 #> 2697 thoracic_slope 13.0974 #> 2698 thoracic_slope 11.3293 #> 2699 thoracic_slope 13.8149 #> 2700 thoracic_slope 11.4337 #> 2701 thoracic_slope 13.4464 #> 2702 thoracic_slope 10.5263 #> 2703 thoracic_slope 16.5844 #> 2704 thoracic_slope 13.1408 #> 2705 thoracic_slope 17.7556 #> 2706 thoracic_slope 8.7872 #> 2707 thoracic_slope 10.957 #> 2708 thoracic_slope 7.5117 #> 2709 thoracic_slope 11.1235 #> 2710 thoracic_slope 11.0132 #> 2711 thoracic_slope 17.6222 #> 2712 thoracic_slope 17.7521 #> 2713 thoracic_slope 15.1873 #> 2714 thoracic_slope 13.6632 #> 2715 thoracic_slope 10.2016 #> 2716 thoracic_slope 11.1443 #> 2717 thoracic_slope 13.6082 #> 2718 thoracic_slope 16.3819 #> 2719 thoracic_slope 7.4752 #> 2720 thoracic_slope 16.1623 #> 2721 thoracic_slope 8.7771 #> 2722 thoracic_slope 11.087 #> 2723 thoracic_slope 12.6508 #> 2724 thoracic_slope 15.279 #> 2725 thoracic_slope 14.0752 #> 2726 thoracic_slope 12.0502 #> 2727 thoracic_slope 11.0671 #> 2728 thoracic_slope 15.5507 #> 2729 thoracic_slope 15.313 #> 2730 thoracic_slope 14.5673 #> 2731 thoracic_slope 17.1414 #> 2732 thoracic_slope 16.527 #> 2733 thoracic_slope 8.5981 #> 2734 thoracic_slope 8.0233 #> 2735 thoracic_slope 12.4063 #> 2736 thoracic_slope 9.9145 #> 2737 thoracic_slope 14.9326 #> 2738 thoracic_slope 16.4723 #> 2739 thoracic_slope 13.9897 #> 2740 thoracic_slope 9.9518 #> 2741 thoracic_slope 9.3492 #> 2742 thoracic_slope 11.3042 #> 2743 thoracic_slope 18.7123 #> 2744 thoracic_slope 11.3129 #> 2745 thoracic_slope 18.7158 #> 2746 thoracic_slope 14.418 #> 2747 thoracic_slope 8.8496 #> 2748 thoracic_slope 15.3118 #> 2749 thoracic_slope 9.5092 #> 2750 thoracic_slope 18.2159 #> 2751 thoracic_slope 10.5134 #> 2752 thoracic_slope 15.9657 #> 2753 thoracic_slope 9.8168 #> 2754 thoracic_slope 11.0116 #> 2755 thoracic_slope 17.7684 #> 2756 thoracic_slope 13.7148 #> 2757 thoracic_slope 12.3509 #> 2758 thoracic_slope 17.5228 #> 2759 thoracic_slope 16.4107 #> 2760 thoracic_slope 19.2659 #> 2761 thoracic_slope 15.1042 #> 2762 thoracic_slope 15.3692 #> 2763 thoracic_slope 11.4937 #> 2764 thoracic_slope 7.5426 #> 2765 thoracic_slope 14.7433 #> 2766 thoracic_slope 15.4016 #> 2767 thoracic_slope 14.9831 #> 2768 thoracic_slope 11.2466 #> 2769 thoracic_slope 15.6236 #> 2770 thoracic_slope 7.9912 #> 2771 thoracic_slope 10.87 #> 2772 thoracic_slope 12.6652 #> 2773 thoracic_slope 11.2762 #> 2774 thoracic_slope 9.9608 #> 2775 thoracic_slope 15.1769 #> 2776 thoracic_slope 10.6369 #> 2777 thoracic_slope 18.3533 #> 2778 thoracic_slope 12.9572 #> 2779 thoracic_slope 11.8978 #> 2780 thoracic_slope 12.3646 #> 2781 thoracic_slope 8.007 #> 2782 thoracic_slope 13.3731 #> 2783 thoracic_slope 11.4198 #> 2784 thoracic_slope 16.6255 #> 2785 thoracic_slope 14.3037 #> 2786 thoracic_slope 14.7484 #> 2787 thoracic_slope 18.1972 #> 2788 thoracic_slope 13.5565 #> 2789 thoracic_slope 16.0928 #> 2790 thoracic_slope 17.6963 #> 2791 cervical_tilt 15.30468 #> 2792 cervical_tilt 16.78486 #> 2793 cervical_tilt 16.65897 #> 2794 cervical_tilt 11.42447 #> 2795 cervical_tilt 8.87237 #> 2796 cervical_tilt 10.40462 #> 2797 cervical_tilt 11.37832 #> 2798 cervical_tilt 7.60961 #> 2799 cervical_tilt 10.59188 #> 2800 cervical_tilt 14.91416 #> 2801 cervical_tilt 15.17645 #> 2802 cervical_tilt 14.76412 #> 2803 cervical_tilt 8.61882 #> 2804 cervical_tilt 12.00109 #> 2805 cervical_tilt 8.51898 #> 2806 cervical_tilt 7.25707 #> 2807 cervical_tilt 11.41344 #> 2808 cervical_tilt 8.4402 #> 2809 cervical_tilt 14.75417 #> 2810 cervical_tilt 14.1961 #> 2811 cervical_tilt 10.32658 #> 2812 cervical_tilt 14.14196 #> 2813 cervical_tilt 7.63771 #> 2814 cervical_tilt 7.70987 #> 2815 cervical_tilt 11.5561 #> 2816 cervical_tilt 16.12951 #> 2817 cervical_tilt 15.78046 #> 2818 cervical_tilt 10.79356 #> 2819 cervical_tilt 11.71324 #> 2820 cervical_tilt 7.58784 #> 2821 cervical_tilt 9.94785 #> 2822 cervical_tilt 7.30184 #> 2823 cervical_tilt 13.54721 #> 2824 cervical_tilt 9.28229 #> 2825 cervical_tilt 8.537 #> 2826 cervical_tilt 11.85555 #> 2827 cervical_tilt 11.00446 #> 2828 cervical_tilt 9.53575 #> 2829 cervical_tilt 10.55193 #> 2830 cervical_tilt 16.64731 #> 2831 cervical_tilt 16.71638 #> 2832 cervical_tilt 9.55731 #> 2833 cervical_tilt 12.37699 #> 2834 cervical_tilt 13.50349 #> 2835 cervical_tilt 14.16436 #> 2836 cervical_tilt 15.55901 #> 2837 cervical_tilt 13.15072 #> 2838 cervical_tilt 16.38754 #> 2839 cervical_tilt 7.88212 #> 2840 cervical_tilt 9.82029 #> 2841 cervical_tilt 14.37627 #> 2842 cervical_tilt 11.62142 #> 2843 cervical_tilt 12.90967 #> 2844 cervical_tilt 16.18484 #> 2845 cervical_tilt 8.09991 #> 2846 cervical_tilt 12.32724 #> 2847 cervical_tilt 9.64034 #> 2848 cervical_tilt 8.75046 #> 2849 cervical_tilt 14.76132 #> 2850 cervical_tilt 15.86896 #> 2851 cervical_tilt 11.71169 #> 2852 cervical_tilt 16.09596 #> 2853 cervical_tilt 16.77905 #> 2854 cervical_tilt 11.10896 #> 2855 cervical_tilt 11.07095 #> 2856 cervical_tilt 9.79573 #> 2857 cervical_tilt 8.03422 #> 2858 cervical_tilt 15.75602 #> 2859 cervical_tilt 10.87581 #> 2860 cervical_tilt 15.06436 #> 2861 cervical_tilt 13.67428 #> 2862 cervical_tilt 8.9351 #> 2863 cervical_tilt 7.886 #> 2864 cervical_tilt 14.35843 #> 2865 cervical_tilt 16.44128 #> 2866 cervical_tilt 11.22353 #> 2867 cervical_tilt 12.70134 #> 2868 cervical_tilt 9.99732 #> 2869 cervical_tilt 11.82259 #> 2870 cervical_tilt 8.43155 #> 2871 cervical_tilt 11.55132 #> 2872 cervical_tilt 15.87462 #> 2873 cervical_tilt 15.27164 #> 2874 cervical_tilt 13.29506 #> 2875 cervical_tilt 13.75752 #> 2876 cervical_tilt 15.89311 #> 2877 cervical_tilt 8.89121 #> 2878 cervical_tilt 15.62397 #> 2879 cervical_tilt 16.40308 #> 2880 cervical_tilt 7.0306 #> 2881 cervical_tilt 11.69024 #> 2882 cervical_tilt 12.21365 #> 2883 cervical_tilt 14.39722 #> 2884 cervical_tilt 12.20428 #> 2885 cervical_tilt 8.97829 #> 2886 cervical_tilt 7.81812 #> 2887 cervical_tilt 11.35399 #> 2888 cervical_tilt 13.97269 #> 2889 cervical_tilt 7.57722 #> 2890 cervical_tilt 10.64403 #> 2891 cervical_tilt 11.58279 #> 2892 cervical_tilt 7.17197 #> 2893 cervical_tilt 12.34978 #> 2894 cervical_tilt 16.26239 #> 2895 cervical_tilt 13.82148 #> 2896 cervical_tilt 11.95397 #> 2897 cervical_tilt 10.84295 #> 2898 cervical_tilt 7.96524 #> 2899 cervical_tilt 16.30352 #> 2900 cervical_tilt 7.1822 #> 2901 cervical_tilt 16.46184 #> 2902 cervical_tilt 9.85541 #> 2903 cervical_tilt 16.20134 #> 2904 cervical_tilt 7.24562 #> 2905 cervical_tilt 11.60893 #> 2906 cervical_tilt 11.36543 #> 2907 cervical_tilt 11.19482 #> 2908 cervical_tilt 9.6275 #> 2909 cervical_tilt 14.63875 #> 2910 cervical_tilt 12.46333 #> 2911 cervical_tilt 14.52239 #> 2912 cervical_tilt 16.2815 #> 2913 cervical_tilt 15.89258 #> 2914 cervical_tilt 16.41116 #> 2915 cervical_tilt 12.17025 #> 2916 cervical_tilt 11.35088 #> 2917 cervical_tilt 11.45991 #> 2918 cervical_tilt 11.96597 #> 2919 cervical_tilt 16.52676 #> 2920 cervical_tilt 7.61063 #> 2921 cervical_tilt 9.1785 #> 2922 cervical_tilt 7.96714 #> 2923 cervical_tilt 15.92252 #> 2924 cervical_tilt 14.66728 #> 2925 cervical_tilt 12.06379 #> 2926 cervical_tilt 16.22809 #> 2927 cervical_tilt 7.39996 #> 2928 cervical_tilt 13.236 #> 2929 cervical_tilt 7.23178 #> 2930 cervical_tilt 11.9815 #> 2931 cervical_tilt 15.38281 #> 2932 cervical_tilt 14.77008 #> 2933 cervical_tilt 9.74172 #> 2934 cervical_tilt 16.82108 #> 2935 cervical_tilt 10.35218 #> 2936 cervical_tilt 15.0803 #> 2937 cervical_tilt 12.33663 #> 2938 cervical_tilt 14.69484 #> 2939 cervical_tilt 12.13128 #> 2940 cervical_tilt 16.56784 #> 2941 cervical_tilt 7.26048 #> 2942 cervical_tilt 8.35383 #> 2943 cervical_tilt 13.33661 #> 2944 cervical_tilt 12.20726 #> 2945 cervical_tilt 9.66748 #> 2946 cervical_tilt 11.90802 #> 2947 cervical_tilt 11.96108 #> 2948 cervical_tilt 11.98973 #> 2949 cervical_tilt 7.99506 #> 2950 cervical_tilt 13.83023 #> 2951 cervical_tilt 11.21248 #> 2952 cervical_tilt 7.23554 #> 2953 cervical_tilt 13.11354 #> 2954 cervical_tilt 11.4896 #> 2955 cervical_tilt 16.73581 #> 2956 cervical_tilt 14.38085 #> 2957 cervical_tilt 16.03569 #> 2958 cervical_tilt 9.36778 #> 2959 cervical_tilt 13.13055 #> 2960 cervical_tilt 9.73836 #> 2961 cervical_tilt 10.20296 #> 2962 cervical_tilt 13.21647 #> 2963 cervical_tilt 15.03453 #> 2964 cervical_tilt 11.35215 #> 2965 cervical_tilt 12.90325 #> 2966 cervical_tilt 12.46567 #> 2967 cervical_tilt 12.63134 #> 2968 cervical_tilt 11.71401 #> 2969 cervical_tilt 8.98429 #> 2970 cervical_tilt 14.48126 #> 2971 cervical_tilt 10.87176 #> 2972 cervical_tilt 13.0176 #> 2973 cervical_tilt 16.74065 #> 2974 cervical_tilt 10.61123 #> 2975 cervical_tilt 12.1325 #> 2976 cervical_tilt 15.45998 #> 2977 cervical_tilt 13.7822 #> 2978 cervical_tilt 11.9537 #> 2979 cervical_tilt 10.73285 #> 2980 cervical_tilt 8.58139 #> 2981 cervical_tilt 9.51271 #> 2982 cervical_tilt 16.38788 #> 2983 cervical_tilt 10.01481 #> 2984 cervical_tilt 7.54207 #> 2985 cervical_tilt 13.75328 #> 2986 cervical_tilt 14.14875 #> 2987 cervical_tilt 14.9747 #> 2988 cervical_tilt 15.2392 #> 2989 cervical_tilt 14.37763 #> 2990 cervical_tilt 11.22559 #> 2991 cervical_tilt 15.12032 #> 2992 cervical_tilt 11.30614 #> 2993 cervical_tilt 12.22936 #> 2994 cervical_tilt 11.84325 #> 2995 cervical_tilt 14.17105 #> 2996 cervical_tilt 9.6995 #> 2997 cervical_tilt 12.2245 #> 2998 cervical_tilt 8.49572 #> 2999 cervical_tilt 9.41012 #> 3000 cervical_tilt 13.79474 #> 3001 cervical_tilt 15.24996 #> 3002 cervical_tilt 8.37076 #> 3003 cervical_tilt 11.3766 #> 3004 cervical_tilt 11.6271 #> 3005 cervical_tilt 16.44832 #> 3006 cervical_tilt 7.55792 #> 3007 cervical_tilt 12.04558 #> 3008 cervical_tilt 14.17628 #> 3009 cervical_tilt 11.2849 #> 3010 cervical_tilt 13.81463 #> 3011 cervical_tilt 9.27506 #> 3012 cervical_tilt 16.4625 #> 3013 cervical_tilt 16.49071 #> 3014 cervical_tilt 9.58711 #> 3015 cervical_tilt 10.98189 #> 3016 cervical_tilt 7.46821 #> 3017 cervical_tilt 13.32169 #> 3018 cervical_tilt 12.11463 #> 3019 cervical_tilt 11.05746 #> 3020 cervical_tilt 8.51675 #> 3021 cervical_tilt 13.39076 #> 3022 cervical_tilt 16.43174 #> 3023 cervical_tilt 10.59114 #> 3024 cervical_tilt 13.015 #> 3025 cervical_tilt 11.24951 #> 3026 cervical_tilt 7.97351 #> 3027 cervical_tilt 8.34518 #> 3028 cervical_tilt 9.66244 #> 3029 cervical_tilt 7.76405 #> 3030 cervical_tilt 14.93052 #> 3031 cervical_tilt 8.64451 #> 3032 cervical_tilt 12.42093 #> 3033 cervical_tilt 12.36056 #> 3034 cervical_tilt 16.49241 #> 3035 cervical_tilt 7.38995 #> 3036 cervical_tilt 15.65862 #> 3037 cervical_tilt 7.58202 #> 3038 cervical_tilt 15.89565 #> 3039 cervical_tilt 13.47998 #> 3040 cervical_tilt 11.98326 #> 3041 cervical_tilt 13.60366 #> 3042 cervical_tilt 15.75651 #> 3043 cervical_tilt 9.07771 #> 3044 cervical_tilt 8.72676 #> 3045 cervical_tilt 7.05411 #> 3046 cervical_tilt 16.25839 #> 3047 cervical_tilt 13.57371 #> 3048 cervical_tilt 8.02315 #> 3049 cervical_tilt 10.31438 #> 3050 cervical_tilt 15.09867 #> 3051 cervical_tilt 14.34981 #> 3052 cervical_tilt 13.47166 #> 3053 cervical_tilt 8.97822 #> 3054 cervical_tilt 13.23731 #> 3055 cervical_tilt 12.21306 #> 3056 cervical_tilt 12.55075 #> 3057 cervical_tilt 12.75813 #> 3058 cervical_tilt 13.91499 #> 3059 cervical_tilt 7.06295 #> 3060 cervical_tilt 9.4178 #> 3061 cervical_tilt 10.13608 #> 3062 cervical_tilt 8.1948 #> 3063 cervical_tilt 15.93402 #> 3064 cervical_tilt 7.92836 #> 3065 cervical_tilt 7.77773 #> 3066 cervical_tilt 10.46265 #> 3067 cervical_tilt 11.99547 #> 3068 cervical_tilt 8.31382 #> 3069 cervical_tilt 7.95631 #> 3070 cervical_tilt 8.31303 #> 3071 cervical_tilt 14.46625 #> 3072 cervical_tilt 16.31794 #> 3073 cervical_tilt 10.80051 #> 3074 cervical_tilt 15.7409 #> 3075 cervical_tilt 8.02792 #> 3076 cervical_tilt 13.86568 #> 3077 cervical_tilt 8.27541 #> 3078 cervical_tilt 9.14463 #> 3079 cervical_tilt 10.54562 #> 3080 cervical_tilt 12.23055 #> 3081 cervical_tilt 7.27404 #> 3082 cervical_tilt 13.92353 #> 3083 cervical_tilt 11.54866 #> 3084 cervical_tilt 10.86798 #> 3085 cervical_tilt 16.49989 #> 3086 cervical_tilt 15.11344 #> 3087 cervical_tilt 13.16102 #> 3088 cervical_tilt 12.40401 #> 3089 cervical_tilt 9.2322 #> 3090 cervical_tilt 16.61754 #> 3091 cervical_tilt 9.74352 #> 3092 cervical_tilt 11.04819 #> 3093 cervical_tilt 13.82322 #> 3094 cervical_tilt 7.20496 #> 3095 cervical_tilt 10.64326 #> 3096 cervical_tilt 8.51707 #> 3097 cervical_tilt 7.08745 #> 3098 cervical_tilt 8.89572 #> 3099 cervical_tilt 9.75922 #> 3100 cervical_tilt 13.72929 #> 3101 sacrum_angle -28.658501 #> 3102 sacrum_angle -25.530607 #> 3103 sacrum_angle -29.031888 #> 3104 sacrum_angle -30.470246 #> 3105 sacrum_angle -16.378376 #> 3106 sacrum_angle -1.512209 #> 3107 sacrum_angle -20.510434 #> 3108 sacrum_angle -25.111459 #> 3109 sacrum_angle -20.020075 #> 3110 sacrum_angle -1.702097 #> 3111 sacrum_angle -0.502127 #> 3112 sacrum_angle -21.724559 #> 3113 sacrum_angle -1.215542 #> 3114 sacrum_angle -1.734117 #> 3115 sacrum_angle -33.441303 #> 3116 sacrum_angle -32.893911 #> 3117 sacrum_angle 2.676002 #> 3118 sacrum_angle 4.482424 #> 3119 sacrum_angle -14.252676 #> 3120 sacrum_angle -20.392538 #> 3121 sacrum_angle -4.986668 #> 3122 sacrum_angle 3.780394 #> 3123 sacrum_angle -14.183602 #> 3124 sacrum_angle -19.37903 #> 3125 sacrum_angle -18.108941 #> 3126 sacrum_angle -17.630363 #> 3127 sacrum_angle -19.650163 #> 3128 sacrum_angle -25.180777 #> 3129 sacrum_angle -28.506125 #> 3130 sacrum_angle -3.963385 #> 3131 sacrum_angle -17.379206 #> 3132 sacrum_angle -24.360827 #> 3133 sacrum_angle -2.925586 #> 3134 sacrum_angle -2.817753 #> 3135 sacrum_angle -0.029028 #> 3136 sacrum_angle -26.650369 #> 3137 sacrum_angle -16.407275 #> 3138 sacrum_angle -14.695641 #> 3139 sacrum_angle -16.404668 #> 3140 sacrum_angle -26.32863 #> 3141 sacrum_angle -2.488862 #> 3142 sacrum_angle -22.35809 #> 3143 sacrum_angle -24.199605 #> 3144 sacrum_angle 1.138079 #> 3145 sacrum_angle -30.035767 #> 3146 sacrum_angle 2.537043 #> 3147 sacrum_angle -4.200276 #> 3148 sacrum_angle -3.494359 #> 3149 sacrum_angle -9.567871 #> 3150 sacrum_angle -6.841914 #> 3151 sacrum_angle 4.779509 #> 3152 sacrum_angle -10.028289 #> 3153 sacrum_angle -30.430498 #> 3154 sacrum_angle -4.763914 #> 3155 sacrum_angle -17.401155 #> 3156 sacrum_angle -26.375211 #> 3157 sacrum_angle -12.480751 #> 3158 sacrum_angle 4.334375 #> 3159 sacrum_angle -24.294191 #> 3160 sacrum_angle 2.50245 #> 3161 sacrum_angle -18.628293 #> 3162 sacrum_angle -18.70133 #> 3163 sacrum_angle -7.146471 #> 3164 sacrum_angle -23.279118 #> 3165 sacrum_angle -34.89766 #> 3166 sacrum_angle -20.130727 #> 3167 sacrum_angle -22.037558 #> 3168 sacrum_angle -0.550516 #> 3169 sacrum_angle -16.918585 #> 3170 sacrum_angle -8.393927 #> 3171 sacrum_angle -19.605044 #> 3172 sacrum_angle -21.31896 #> 3173 sacrum_angle 4.442569 #> 3174 sacrum_angle -33.990906 #> 3175 sacrum_angle -9.487619 #> 3176 sacrum_angle 4.641629 #> 3177 sacrum_angle -15.088499 #> 3178 sacrum_angle -0.622813 #> 3179 sacrum_angle -14.55007 #> 3180 sacrum_angle -33.338734 #> 3181 sacrum_angle -1.860646 #> 3182 sacrum_angle -10.624659 #> 3183 sacrum_angle -4.208953 #> 3184 sacrum_angle -12.139219 #> 3185 sacrum_angle -32.106343 #> 3186 sacrum_angle -10.750511 #> 3187 sacrum_angle -32.248563 #> 3188 sacrum_angle -22.670743 #> 3189 sacrum_angle -35.287375 #> 3190 sacrum_angle -4.667443 #> 3191 sacrum_angle -25.011107 #> 3192 sacrum_angle -5.091336 #> 3193 sacrum_angle -12.551344 #> 3194 sacrum_angle -22.326717 #> 3195 sacrum_angle -3.549557 #> 3196 sacrum_angle -27.570464 #> 3197 sacrum_angle -14.093301 #> 3198 sacrum_angle -5.115515 #> 3199 sacrum_angle 0.307904 #> 3200 sacrum_angle -26.05199 #> 3201 sacrum_angle -1.273566 #> 3202 sacrum_angle -34.653679 #> 3203 sacrum_angle 6.089565 #> 3204 sacrum_angle -0.89559 #> 3205 sacrum_angle 6.079425 #> 3206 sacrum_angle -20.735613 #> 3207 sacrum_angle 6.573829 #> 3208 sacrum_angle -19.123087 #> 3209 sacrum_angle -4.447261 #> 3210 sacrum_angle -20.883262 #> 3211 sacrum_angle -1.682575 #> 3212 sacrum_angle -19.314135 #> 3213 sacrum_angle 0.043299 #> 3214 sacrum_angle -7.96908 #> 3215 sacrum_angle -13.600284 #> 3216 sacrum_angle -34.202073 #> 3217 sacrum_angle -1.999994 #> 3218 sacrum_angle 5.603229 #> 3219 sacrum_angle -15.898046 #> 3220 sacrum_angle -17.516477 #> 3221 sacrum_angle -18.805512 #> 3222 sacrum_angle -8.553212 #> 3223 sacrum_angle -14.15607 #> 3224 sacrum_angle -24.064246 #> 3225 sacrum_angle 1.594477 #> 3226 sacrum_angle -25.723134 #> 3227 sacrum_angle -18.475476 #> 3228 sacrum_angle -25.207568 #> 3229 sacrum_angle -10.917156 #> 3230 sacrum_angle -32.87061 #> 3231 sacrum_angle 5.120515 #> 3232 sacrum_angle -15.682309 #> 3233 sacrum_angle 1.320769 #> 3234 sacrum_angle -3.994716 #> 3235 sacrum_angle -2.974064 #> 3236 sacrum_angle -0.05579 #> 3237 sacrum_angle -11.029157 #> 3238 sacrum_angle -21.449617 #> 3239 sacrum_angle -2.990023 #> 3240 sacrum_angle -25.387556 #> 3241 sacrum_angle -2.504431 #> 3242 sacrum_angle 6.868423 #> 3243 sacrum_angle -33.848051 #> 3244 sacrum_angle -30.591567 #> 3245 sacrum_angle 1.270053 #> 3246 sacrum_angle -1.056403 #> 3247 sacrum_angle -15.774389 #> 3248 sacrum_angle -4.850453 #> 3249 sacrum_angle -5.163605 #> 3250 sacrum_angle -0.26959 #> 3251 sacrum_angle -15.985527 #> 3252 sacrum_angle -32.826775 #> 3253 sacrum_angle -24.275516 #> 3254 sacrum_angle -21.986137 #> 3255 sacrum_angle -9.571667 #> 3256 sacrum_angle -7.585607 #> 3257 sacrum_angle 5.237386 #> 3258 sacrum_angle 5.365967 #> 3259 sacrum_angle -26.223143 #> 3260 sacrum_angle -27.923626 #> 3261 sacrum_angle -19.264777 #> 3262 sacrum_angle -32.43783 #> 3263 sacrum_angle -17.52081 #> 3264 sacrum_angle -6.754004 #> 3265 sacrum_angle -17.874616 #> 3266 sacrum_angle -20.214168 #> 3267 sacrum_angle -0.718491 #> 3268 sacrum_angle -33.489059 #> 3269 sacrum_angle -6.412477 #> 3270 sacrum_angle -6.195062 #> 3271 sacrum_angle -33.025711 #> 3272 sacrum_angle -29.71579 #> 3273 sacrum_angle -16.408583 #> 3274 sacrum_angle -26.496342 #> 3275 sacrum_angle -27.124475 #> 3276 sacrum_angle 0.74424 #> 3277 sacrum_angle -21.818611 #> 3278 sacrum_angle -32.340192 #> 3279 sacrum_angle -19.329173 #> 3280 sacrum_angle -31.852569 #> 3281 sacrum_angle -11.190833 #> 3282 sacrum_angle -20.526277 #> 3283 sacrum_angle -22.735586 #> 3284 sacrum_angle -23.883075 #> 3285 sacrum_angle -4.893491 #> 3286 sacrum_angle -11.179414 #> 3287 sacrum_angle -23.922201 #> 3288 sacrum_angle -1.30467 #> 3289 sacrum_angle 0.627326 #> 3290 sacrum_angle -31.511149 #> 3291 sacrum_angle -8.828259 #> 3292 sacrum_angle -11.786587 #> 3293 sacrum_angle -27.349859 #> 3294 sacrum_angle -5.95662 #> 3295 sacrum_angle -21.282686 #> 3296 sacrum_angle -19.895641 #> 3297 sacrum_angle -22.28622 #> 3298 sacrum_angle -15.444826 #> 3299 sacrum_angle 4.230442 #> 3300 sacrum_angle -12.196755 #> 3301 sacrum_angle 1.278801 #> 3302 sacrum_angle -3.519343 #> 3303 sacrum_angle 4.894807 #> 3304 sacrum_angle -34.729173 #> 3305 sacrum_angle -31.121553 #> 3306 sacrum_angle 4.44648 #> 3307 sacrum_angle -21.695815 #> 3308 sacrum_angle -0.33713 #> 3309 sacrum_angle 5.212541 #> 3310 sacrum_angle -8.044644 #> 3311 sacrum_angle -28.833891 #> 3312 sacrum_angle -17.723457 #> 3313 sacrum_angle -5.202362 #> 3314 sacrum_angle -28.598802 #> 3315 sacrum_angle -13.438814 #> 3316 sacrum_angle 2.00213 #> 3317 sacrum_angle -9.237245 #> 3318 sacrum_angle -30.312742 #> 3319 sacrum_angle -25.770956 #> 3320 sacrum_angle -30.220853 #> 3321 sacrum_angle -8.230224 #> 3322 sacrum_angle -31.937038 #> 3323 sacrum_angle -8.594772 #> 3324 sacrum_angle -22.403652 #> 3325 sacrum_angle -16.891891 #> 3326 sacrum_angle 3.916838 #> 3327 sacrum_angle -9.939014 #> 3328 sacrum_angle -19.934103 #> 3329 sacrum_angle 5.412408 #> 3330 sacrum_angle -23.90419 #> 3331 sacrum_angle -16.36997 #> 3332 sacrum_angle 0.138844 #> 3333 sacrum_angle -17.943314 #> 3334 sacrum_angle -4.591917 #> 3335 sacrum_angle -19.160909 #> 3336 sacrum_angle -7.809627 #> 3337 sacrum_angle -10.939434 #> 3338 sacrum_angle -16.783645 #> 3339 sacrum_angle -11.716465 #> 3340 sacrum_angle -12.656406 #> 3341 sacrum_angle -5.079724 #> 3342 sacrum_angle -15.259539 #> 3343 sacrum_angle -34.071611 #> 3344 sacrum_angle -3.437709 #> 3345 sacrum_angle 6.635051 #> 3346 sacrum_angle -4.137017 #> 3347 sacrum_angle 5.157255 #> 3348 sacrum_angle -28.277945 #> 3349 sacrum_angle -1.889282 #> 3350 sacrum_angle -26.881964 #> 3351 sacrum_angle -12.162522 #> 3352 sacrum_angle -28.284145 #> 3353 sacrum_angle -27.62739 #> 3354 sacrum_angle -8.842283 #> 3355 sacrum_angle -9.04664 #> 3356 sacrum_angle -27.302888 #> 3357 sacrum_angle -18.445718 #> 3358 sacrum_angle -34.615429 #> 3359 sacrum_angle -30.255112 #> 3360 sacrum_angle -26.758834 #> 3361 sacrum_angle -19.444964 #> 3362 sacrum_angle -20.789258 #> 3363 sacrum_angle -14.510574 #> 3364 sacrum_angle -2.244119 #> 3365 sacrum_angle 5.672553 #> 3366 sacrum_angle -24.517621 #> 3367 sacrum_angle 3.332207 #> 3368 sacrum_angle -32.013803 #> 3369 sacrum_angle -31.862036 #> 3370 sacrum_angle -31.427534 #> 3371 sacrum_angle 5.738583 #> 3372 sacrum_angle -33.380627 #> 3373 sacrum_angle -8.871793 #> 3374 sacrum_angle -5.852864 #> 3375 sacrum_angle -35.077537 #> 3376 sacrum_angle -9.187554 #> 3377 sacrum_angle -34.927709 #> 3378 sacrum_angle 6.972071 #> 3379 sacrum_angle -11.376063 #> 3380 sacrum_angle -22.690688 #> 3381 sacrum_angle -7.052293 #> 3382 sacrum_angle -4.179409 #> 3383 sacrum_angle -30.625194 #> 3384 sacrum_angle -6.421289 #> 3385 sacrum_angle -33.650471 #> 3386 sacrum_angle -17.473008 #> 3387 sacrum_angle -0.48876 #> 3388 sacrum_angle -29.11456 #> 3389 sacrum_angle -32.494544 #> 3390 sacrum_angle -26.340144 #> 3391 sacrum_angle -26.195988 #> 3392 sacrum_angle -3.478546 #> 3393 sacrum_angle -12.02522 #> 3394 sacrum_angle 1.956131 #> 3395 sacrum_angle -22.420021 #> 3396 sacrum_angle 2.963625 #> 3397 sacrum_angle -6.652617 #> 3398 sacrum_angle -12.363109 #> 3399 sacrum_angle -14.824364 #> 3400 sacrum_angle -15.758791 #> 3401 sacrum_angle -1.228604 #> 3402 sacrum_angle -3.5053 #> 3403 sacrum_angle -5.606449 #> 3404 sacrum_angle -31.374823 #> 3405 sacrum_angle -31.198847 #> 3406 sacrum_angle -15.728927 #> 3407 sacrum_angle 6.013843 #> 3408 sacrum_angle 3.564463 #> 3409 sacrum_angle 5.767308 #> 3410 sacrum_angle 1.783007 #> 3411 scoliosis_slope 43.5123 #> 3412 scoliosis_slope 16.1102 #> 3413 scoliosis_slope 19.2221 #> 3414 scoliosis_slope 18.8329 #> 3415 scoliosis_slope 24.9171 #> 3416 scoliosis_slope 9.6548 #> 3417 scoliosis_slope 25.9477 #> 3418 scoliosis_slope 26.3543 #> 3419 scoliosis_slope 40.0276 #> 3420 scoliosis_slope 21.432 #> 3421 scoliosis_slope 18.3437 #> 3422 scoliosis_slope 36.4449 #> 3423 scoliosis_slope 27.3713 #> 3424 scoliosis_slope 15.6205 #> 3425 scoliosis_slope 13.2498 #> 3426 scoliosis_slope 19.5695 #> 3427 scoliosis_slope 17.3859 #> 3428 scoliosis_slope 24.6513 #> 3429 scoliosis_slope 24.9361 #> 3430 scoliosis_slope 33.0265 #> 3431 scoliosis_slope 22.4667 #> 3432 scoliosis_slope 24.9278 #> 3433 scoliosis_slope 44.2338 #> 3434 scoliosis_slope 20.3649 #> 3435 scoliosis_slope 24.1151 #> 3436 scoliosis_slope 28.1902 #> 3437 scoliosis_slope 43.955 #> 3438 scoliosis_slope 18.3196 #> 3439 scoliosis_slope 28.047 #> 3440 scoliosis_slope 27.3587 #> 3441 scoliosis_slope 14.7187 #> 3442 scoliosis_slope 28.2366 #> 3443 scoliosis_slope 36.0452 #> 3444 scoliosis_slope 31.5193 #> 3445 scoliosis_slope 40.5823 #> 3446 scoliosis_slope 12.6599 #> 3447 scoliosis_slope 38.8912 #> 3448 scoliosis_slope 38.7458 #> 3449 scoliosis_slope 15.2954 #> 3450 scoliosis_slope 39.2753 #> 3451 scoliosis_slope 20.0076 #> 3452 scoliosis_slope 14.3317 #> 3453 scoliosis_slope 11.3375 #> 3454 scoliosis_slope 34.3683 #> 3455 scoliosis_slope 22.4654 #> 3456 scoliosis_slope 9.431 #> 3457 scoliosis_slope 20.0348 #> 3458 scoliosis_slope 14.5269 #> 3459 scoliosis_slope 11.9238 #> 3460 scoliosis_slope 11.6156 #> 3461 scoliosis_slope 43.261 #> 3462 scoliosis_slope 9.3141 #> 3463 scoliosis_slope 31.6999 #> 3464 scoliosis_slope 26.8917 #> 3465 scoliosis_slope 22.8036 #> 3466 scoliosis_slope 14.1334 #> 3467 scoliosis_slope 13.0244 #> 3468 scoliosis_slope 16.8302 #> 3469 scoliosis_slope 23.8843 #> 3470 scoliosis_slope 23.0762 #> 3471 scoliosis_slope 22.5623 #> 3472 scoliosis_slope 35.8729 #> 3473 scoliosis_slope 13.5143 #> 3474 scoliosis_slope 16.1132 #> 3475 scoliosis_slope 43.1487 #> 3476 scoliosis_slope 22.4032 #> 3477 scoliosis_slope 32.0972 #> 3478 scoliosis_slope 18.2915 #> 3479 scoliosis_slope 14.0322 #> 3480 scoliosis_slope 30.7765 #> 3481 scoliosis_slope 22.759 #> 3482 scoliosis_slope 12.8518 #> 3483 scoliosis_slope 13.4605 #> 3484 scoliosis_slope 25.5218 #> 3485 scoliosis_slope 9.6867 #> 3486 scoliosis_slope 9.8472 #> 3487 scoliosis_slope 7.0079 #> 3488 scoliosis_slope 24.3675 #> 3489 scoliosis_slope 42.2698 #> 3490 scoliosis_slope 22.7226 #> 3491 scoliosis_slope 18.6033 #> 3492 scoliosis_slope 31.9699 #> 3493 scoliosis_slope 32.934 #> 3494 scoliosis_slope 11.8487 #> 3495 scoliosis_slope 18.6868 #> 3496 scoliosis_slope 22.7075 #> 3497 scoliosis_slope 40.3949 #> 3498 scoliosis_slope 37.0558 #> 3499 scoliosis_slope 31.6243 #> 3500 scoliosis_slope 14.5344 #> 3501 scoliosis_slope 21.918 #> 3502 scoliosis_slope 17.2601 #> 3503 scoliosis_slope 39.8494 #> 3504 scoliosis_slope 19.5797 #> 3505 scoliosis_slope 7.3222 #> 3506 scoliosis_slope 17.8768 #> 3507 scoliosis_slope 20.818 #> 3508 scoliosis_slope 20.6402 #> 3509 scoliosis_slope 33.7201 #> 3510 scoliosis_slope 10.4338 #> 3511 scoliosis_slope 29.6399 #> 3512 scoliosis_slope 28.1835 #> 3513 scoliosis_slope 34.9908 #> 3514 scoliosis_slope 38.4719 #> 3515 scoliosis_slope 11.8698 #> 3516 scoliosis_slope 9.7675 #> 3517 scoliosis_slope 35.1025 #> 3518 scoliosis_slope 16.1431 #> 3519 scoliosis_slope 41.326 #> 3520 scoliosis_slope 17.113 #> 3521 scoliosis_slope 13.9094 #> 3522 scoliosis_slope 43.0086 #> 3523 scoliosis_slope 20.6529 #> 3524 scoliosis_slope 33.678 #> 3525 scoliosis_slope 34.3656 #> 3526 scoliosis_slope 27.5144 #> 3527 scoliosis_slope 26.1908 #> 3528 scoliosis_slope 36.2899 #> 3529 scoliosis_slope 19.5298 #> 3530 scoliosis_slope 22.9375 #> 3531 scoliosis_slope 33.1902 #> 3532 scoliosis_slope 24.8562 #> 3533 scoliosis_slope 39.973 #> 3534 scoliosis_slope 15.9787 #> 3535 scoliosis_slope 31.4138 #> 3536 scoliosis_slope 40.82 #> 3537 scoliosis_slope 19.8407 #> 3538 scoliosis_slope 21.545 #> 3539 scoliosis_slope 35.6543 #> 3540 scoliosis_slope 19.6294 #> 3541 scoliosis_slope 18.8581 #> 3542 scoliosis_slope 34.906 #> 3543 scoliosis_slope 34.8665 #> 3544 scoliosis_slope 33.2091 #> 3545 scoliosis_slope 24.2895 #> 3546 scoliosis_slope 27.6595 #> 3547 scoliosis_slope 40.7572 #> 3548 scoliosis_slope 16.2153 #> 3549 scoliosis_slope 29.9404 #> 3550 scoliosis_slope 8.3163 #> 3551 scoliosis_slope 14.2555 #> 3552 scoliosis_slope 29.1844 #> 3553 scoliosis_slope 9.4194 #> 3554 scoliosis_slope 35.4529 #> 3555 scoliosis_slope 10.4207 #> 3556 scoliosis_slope 11.4148 #> 3557 scoliosis_slope 40.4629 #> 3558 scoliosis_slope 18.1958 #> 3559 scoliosis_slope 43.2649 #> 3560 scoliosis_slope 31.7726 #> 3561 scoliosis_slope 29.5574 #> 3562 scoliosis_slope 38.8071 #> 3563 scoliosis_slope 9.173 #> 3564 scoliosis_slope 23.1484 #> 3565 scoliosis_slope 30.5253 #> 3566 scoliosis_slope 39.7443 #> 3567 scoliosis_slope 10.8006 #> 3568 scoliosis_slope 13.7113 #> 3569 scoliosis_slope 40.1564 #> 3570 scoliosis_slope 7.0698 #> 3571 scoliosis_slope 19.9972 #> 3572 scoliosis_slope 33.0292 #> 3573 scoliosis_slope 21.2408 #> 3574 scoliosis_slope 32.5082 #> 3575 scoliosis_slope 19.5841 #> 3576 scoliosis_slope 11.7348 #> 3577 scoliosis_slope 34.6601 #> 3578 scoliosis_slope 28.9479 #> 3579 scoliosis_slope 19.9792 #> 3580 scoliosis_slope 25.57 #> 3581 scoliosis_slope 17.1654 #> 3582 scoliosis_slope 19.1168 #> 3583 scoliosis_slope 33.2835 #> 3584 scoliosis_slope 33.9151 #> 3585 scoliosis_slope 22.9564 #> 3586 scoliosis_slope 32.4471 #> 3587 scoliosis_slope 40.6229 #> 3588 scoliosis_slope 27.1091 #> 3589 scoliosis_slope 31.0862 #> 3590 scoliosis_slope 30.533 #> 3591 scoliosis_slope 29.909 #> 3592 scoliosis_slope 17.3548 #> 3593 scoliosis_slope 43.1209 #> 3594 scoliosis_slope 38.7949 #> 3595 scoliosis_slope 29.244 #> 3596 scoliosis_slope 30.316 #> 3597 scoliosis_slope 29.5953 #> 3598 scoliosis_slope 34.2437 #> 3599 scoliosis_slope 11.2469 #> 3600 scoliosis_slope 32.6221 #> 3601 scoliosis_slope 12.1023 #> 3602 scoliosis_slope 10.0888 #> 3603 scoliosis_slope 14.9309 #> 3604 scoliosis_slope 43.8608 #> 3605 scoliosis_slope 41.2131 #> 3606 scoliosis_slope 8.6239 #> 3607 scoliosis_slope 21.7822 #> 3608 scoliosis_slope 7.2157 #> 3609 scoliosis_slope 39.2266 #> 3610 scoliosis_slope 31.7385 #> 3611 scoliosis_slope 39.9331 #> 3612 scoliosis_slope 28.2238 #> 3613 scoliosis_slope 16.5217 #> 3614 scoliosis_slope 26.674 #> 3615 scoliosis_slope 32.2469 #> 3616 scoliosis_slope 36.0749 #> 3617 scoliosis_slope 38.7849 #> 3618 scoliosis_slope 11.6844 #> 3619 scoliosis_slope 28.6308 #> 3620 scoliosis_slope 21.6135 #> 3621 scoliosis_slope 18.0442 #> 3622 scoliosis_slope 9.8711 #> 3623 scoliosis_slope 33.2503 #> 3624 scoliosis_slope 10.3379 #> 3625 scoliosis_slope 34.2846 #> 3626 scoliosis_slope 30.1215 #> 3627 scoliosis_slope 31.722 #> 3628 scoliosis_slope 38.1538 #> 3629 scoliosis_slope 35.6191 #> 3630 scoliosis_slope 17.9129 #> 3631 scoliosis_slope 15.5635 #> 3632 scoliosis_slope 22.1518 #> 3633 scoliosis_slope 7.7532 #> 3634 scoliosis_slope 12.5917 #> 3635 scoliosis_slope 28.09 #> 3636 scoliosis_slope 43.4384 #> 3637 scoliosis_slope 31.3141 #> 3638 scoliosis_slope 41.7304 #> 3639 scoliosis_slope 38.2581 #> 3640 scoliosis_slope 35.0749 #> 3641 scoliosis_slope 21.4495 #> 3642 scoliosis_slope 30.7846 #> 3643 scoliosis_slope 33.0483 #> 3644 scoliosis_slope 19.9869 #> 3645 scoliosis_slope 34.0011 #> 3646 scoliosis_slope 29.5091 #> 3647 scoliosis_slope 20.7594 #> 3648 scoliosis_slope 43.8402 #> 3649 scoliosis_slope 13.0886 #> 3650 scoliosis_slope 40.5705 #> 3651 scoliosis_slope 29.4263 #> 3652 scoliosis_slope 18.2936 #> 3653 scoliosis_slope 43.7183 #> 3654 scoliosis_slope 21.8868 #> 3655 scoliosis_slope 13.4565 #> 3656 scoliosis_slope 39.6199 #> 3657 scoliosis_slope 17.469 #> 3658 scoliosis_slope 22.042 #> 3659 scoliosis_slope 37.7836 #> 3660 scoliosis_slope 10.3342 #> 3661 scoliosis_slope 28.3449 #> 3662 scoliosis_slope 7.4324 #> 3663 scoliosis_slope 12.7281 #> 3664 scoliosis_slope 34.5721 #> 3665 scoliosis_slope 16.8122 #> 3666 scoliosis_slope 25.0493 #> 3667 scoliosis_slope 13.8497 #> 3668 scoliosis_slope 9.9615 #> 3669 scoliosis_slope 18.7992 #> 3670 scoliosis_slope 40.4129 #> 3671 scoliosis_slope 40.8512 #> 3672 scoliosis_slope 41.2149 #> 3673 scoliosis_slope 22.6375 #> 3674 scoliosis_slope 10.2925 #> 3675 scoliosis_slope 43.6689 #> 3676 scoliosis_slope 18.4675 #> 3677 scoliosis_slope 9.3333 #> 3678 scoliosis_slope 17.6135 #> 3679 scoliosis_slope 24.3522 #> 3680 scoliosis_slope 35.7839 #> 3681 scoliosis_slope 36.7874 #> 3682 scoliosis_slope 30.2644 #> 3683 scoliosis_slope 12.5514 #> 3684 scoliosis_slope 22.7358 #> 3685 scoliosis_slope 27.5972 #> 3686 scoliosis_slope 27.8587 #> 3687 scoliosis_slope 19.3766 #> 3688 scoliosis_slope 31.7892 #> 3689 scoliosis_slope 23.331 #> 3690 scoliosis_slope 39.6096 #> 3691 scoliosis_slope 15.9536 #> 3692 scoliosis_slope 32.9659 #> 3693 scoliosis_slope 41.901 #> 3694 scoliosis_slope 11.9857 #> 3695 scoliosis_slope 16.7094 #> 3696 scoliosis_slope 39.3526 #> 3697 scoliosis_slope 24.9564 #> 3698 scoliosis_slope 40.9249 #> 3699 scoliosis_slope 16.6229 #> 3700 scoliosis_slope 32.3929 #> 3701 scoliosis_slope 21.8465 #> 3702 scoliosis_slope 28.7425 #> 3703 scoliosis_slope 44.3412 #> 3704 scoliosis_slope 23.7274 #> 3705 scoliosis_slope 40.2061 #> 3706 scoliosis_slope 23.0719 #> 3707 scoliosis_slope 26.3297 #> 3708 scoliosis_slope 31.9668 #> 3709 scoliosis_slope 43.8409 #> 3710 scoliosis_slope 35.9458 #> 3711 scoliosis_slope 14.2547 #> 3712 scoliosis_slope 33.4196 #> 3713 scoliosis_slope 18.5514 #> 3714 scoliosis_slope 29.5748 #> 3715 scoliosis_slope 11.2307 #> 3716 scoliosis_slope 11.5472 #> 3717 scoliosis_slope 43.8693 #> 3718 scoliosis_slope 18.4151 #> 3719 scoliosis_slope 33.7192 #> 3720 scoliosis_slope 40.6049 #> 3721 class_attribute Abnormal #> 3722 class_attribute Abnormal #> 3723 class_attribute Abnormal #> 3724 class_attribute Abnormal #> 3725 class_attribute Abnormal #> 3726 class_attribute Abnormal #> 3727 class_attribute Abnormal #> 3728 class_attribute Abnormal #> 3729 class_attribute Abnormal #> 3730 class_attribute Abnormal #> 3731 class_attribute Abnormal #> 3732 class_attribute Abnormal #> 3733 class_attribute Abnormal #> 3734 class_attribute Abnormal #> 3735 class_attribute Abnormal #> 3736 class_attribute Abnormal #> 3737 class_attribute Abnormal #> 3738 class_attribute Abnormal #> 3739 class_attribute Abnormal #> 3740 class_attribute Abnormal #> 3741 class_attribute Abnormal #> 3742 class_attribute Abnormal #> 3743 class_attribute Abnormal #> 3744 class_attribute Abnormal #> 3745 class_attribute Abnormal #> 3746 class_attribute Abnormal #> 3747 class_attribute Abnormal #> 3748 class_attribute Abnormal #> 3749 class_attribute Abnormal #> 3750 class_attribute Abnormal #> 3751 class_attribute Abnormal #> 3752 class_attribute Abnormal #> 3753 class_attribute Abnormal #> 3754 class_attribute Abnormal #> 3755 class_attribute Abnormal #> 3756 class_attribute Abnormal #> 3757 class_attribute Abnormal #> 3758 class_attribute Abnormal #> 3759 class_attribute Abnormal #> 3760 class_attribute Abnormal #> 3761 class_attribute Abnormal #> 3762 class_attribute Abnormal #> 3763 class_attribute Abnormal #> 3764 class_attribute Abnormal #> 3765 class_attribute Abnormal #> 3766 class_attribute Abnormal #> 3767 class_attribute Abnormal #> 3768 class_attribute Abnormal #> 3769 class_attribute Abnormal #> 3770 class_attribute Abnormal #> 3771 class_attribute Abnormal #> 3772 class_attribute Abnormal #> 3773 class_attribute Abnormal #> 3774 class_attribute Abnormal #> 3775 class_attribute Abnormal #> 3776 class_attribute Abnormal #> 3777 class_attribute Abnormal #> 3778 class_attribute Abnormal #> 3779 class_attribute Abnormal #> 3780 class_attribute Abnormal #> 3781 class_attribute Abnormal #> 3782 class_attribute Abnormal #> 3783 class_attribute Abnormal #> 3784 class_attribute Abnormal #> 3785 class_attribute Abnormal #> 3786 class_attribute Abnormal #> 3787 class_attribute Abnormal #> 3788 class_attribute Abnormal #> 3789 class_attribute Abnormal #> 3790 class_attribute Abnormal #> 3791 class_attribute Abnormal #> 3792 class_attribute Abnormal #> 3793 class_attribute Abnormal #> 3794 class_attribute Abnormal #> 3795 class_attribute Abnormal #> 3796 class_attribute Abnormal #> 3797 class_attribute Abnormal #> 3798 class_attribute Abnormal #> 3799 class_attribute Abnormal #> 3800 class_attribute Abnormal #> 3801 class_attribute Abnormal #> 3802 class_attribute Abnormal #> 3803 class_attribute Abnormal #> 3804 class_attribute Abnormal #> 3805 class_attribute Abnormal #> 3806 class_attribute Abnormal #> 3807 class_attribute Abnormal #> 3808 class_attribute Abnormal #> 3809 class_attribute Abnormal #> 3810 class_attribute Abnormal #> 3811 class_attribute Abnormal #> 3812 class_attribute Abnormal #> 3813 class_attribute Abnormal #> 3814 class_attribute Abnormal #> 3815 class_attribute Abnormal #> 3816 class_attribute Abnormal #> 3817 class_attribute Abnormal #> 3818 class_attribute Abnormal #> 3819 class_attribute Abnormal #> 3820 class_attribute Abnormal #> 3821 class_attribute Abnormal #> 3822 class_attribute Abnormal #> 3823 class_attribute Abnormal #> 3824 class_attribute Abnormal #> 3825 class_attribute Abnormal #> 3826 class_attribute Abnormal #> 3827 class_attribute Abnormal #> 3828 class_attribute Abnormal #> 3829 class_attribute Abnormal #> 3830 class_attribute Abnormal #> 3831 class_attribute Abnormal #> 3832 class_attribute Abnormal #> 3833 class_attribute Abnormal #> 3834 class_attribute Abnormal #> 3835 class_attribute Abnormal #> 3836 class_attribute Abnormal #> 3837 class_attribute Abnormal #> 3838 class_attribute Abnormal #> 3839 class_attribute Abnormal #> 3840 class_attribute Abnormal #> 3841 class_attribute Abnormal #> 3842 class_attribute Abnormal #> 3843 class_attribute Abnormal #> 3844 class_attribute Abnormal #> 3845 class_attribute Abnormal #> 3846 class_attribute Abnormal #> 3847 class_attribute Abnormal #> 3848 class_attribute Abnormal #> 3849 class_attribute Abnormal #> 3850 class_attribute Abnormal #> 3851 class_attribute Abnormal #> 3852 class_attribute Abnormal #> 3853 class_attribute Abnormal #> 3854 class_attribute Abnormal #> 3855 class_attribute Abnormal #> 3856 class_attribute Abnormal #> 3857 class_attribute Abnormal #> 3858 class_attribute Abnormal #> 3859 class_attribute Abnormal #> 3860 class_attribute Abnormal #> 3861 class_attribute Abnormal #> 3862 class_attribute Abnormal #> 3863 class_attribute Abnormal #> 3864 class_attribute Abnormal #> 3865 class_attribute Abnormal #> 3866 class_attribute Abnormal #> 3867 class_attribute Abnormal #> 3868 class_attribute Abnormal #> 3869 class_attribute Abnormal #> 3870 class_attribute Abnormal #> 3871 class_attribute Abnormal #> 3872 class_attribute Abnormal #> 3873 class_attribute Abnormal #> 3874 class_attribute Abnormal #> 3875 class_attribute Abnormal #> 3876 class_attribute Abnormal #> 3877 class_attribute Abnormal #> 3878 class_attribute Abnormal #> 3879 class_attribute Abnormal #> 3880 class_attribute Abnormal #> 3881 class_attribute Abnormal #> 3882 class_attribute Abnormal #> 3883 class_attribute Abnormal #> 3884 class_attribute Abnormal #> 3885 class_attribute Abnormal #> 3886 class_attribute Abnormal #> 3887 class_attribute Abnormal #> 3888 class_attribute Abnormal #> 3889 class_attribute Abnormal #> 3890 class_attribute Abnormal #> 3891 class_attribute Abnormal #> 3892 class_attribute Abnormal #> 3893 class_attribute Abnormal #> 3894 class_attribute Abnormal #> 3895 class_attribute Abnormal #> 3896 class_attribute Abnormal #> 3897 class_attribute Abnormal #> 3898 class_attribute Abnormal #> 3899 class_attribute Abnormal #> 3900 class_attribute Abnormal #> 3901 class_attribute Abnormal #> 3902 class_attribute Abnormal #> 3903 class_attribute Abnormal #> 3904 class_attribute Abnormal #> 3905 class_attribute Abnormal #> 3906 class_attribute Abnormal #> 3907 class_attribute Abnormal #> 3908 class_attribute Abnormal #> 3909 class_attribute Abnormal #> 3910 class_attribute Abnormal #> 3911 class_attribute Abnormal #> 3912 class_attribute Abnormal #> 3913 class_attribute Abnormal #> 3914 class_attribute Abnormal #> 3915 class_attribute Abnormal #> 3916 class_attribute Abnormal #> 3917 class_attribute Abnormal #> 3918 class_attribute Abnormal #> 3919 class_attribute Abnormal #> 3920 class_attribute Abnormal #> 3921 class_attribute Abnormal #> 3922 class_attribute Abnormal #> 3923 class_attribute Abnormal #> 3924 class_attribute Abnormal #> 3925 class_attribute Abnormal #> 3926 class_attribute Abnormal #> 3927 class_attribute Abnormal #> 3928 class_attribute Abnormal #> 3929 class_attribute Abnormal #> 3930 class_attribute Abnormal #> 3931 class_attribute Normal #> 3932 class_attribute Normal #> 3933 class_attribute Normal #> 3934 class_attribute Normal #> 3935 class_attribute Normal #> 3936 class_attribute Normal #> 3937 class_attribute Normal #> 3938 class_attribute Normal #> 3939 class_attribute Normal #> 3940 class_attribute Normal #> 3941 class_attribute Normal #> 3942 class_attribute Normal #> 3943 class_attribute Normal #> 3944 class_attribute Normal #> 3945 class_attribute Normal #> 3946 class_attribute Normal #> 3947 class_attribute Normal #> 3948 class_attribute Normal #> 3949 class_attribute Normal #> 3950 class_attribute Normal #> 3951 class_attribute Normal #> 3952 class_attribute Normal #> 3953 class_attribute Normal #> 3954 class_attribute Normal #> 3955 class_attribute Normal #> 3956 class_attribute Normal #> 3957 class_attribute Normal #> 3958 class_attribute Normal #> 3959 class_attribute Normal #> 3960 class_attribute Normal #> 3961 class_attribute Normal #> 3962 class_attribute Normal #> 3963 class_attribute Normal #> 3964 class_attribute Normal #> 3965 class_attribute Normal #> 3966 class_attribute Normal #> 3967 class_attribute Normal #> 3968 class_attribute Normal #> 3969 class_attribute Normal #> 3970 class_attribute Normal #> 3971 class_attribute Normal #> 3972 class_attribute Normal #> 3973 class_attribute Normal #> 3974 class_attribute Normal #> 3975 class_attribute Normal #> 3976 class_attribute Normal #> 3977 class_attribute Normal #> 3978 class_attribute Normal #> 3979 class_attribute Normal #> 3980 class_attribute Normal #> 3981 class_attribute Normal #> 3982 class_attribute Normal #> 3983 class_attribute Normal #> 3984 class_attribute Normal #> 3985 class_attribute Normal #> 3986 class_attribute Normal #> 3987 class_attribute Normal #> 3988 class_attribute Normal #> 3989 class_attribute Normal #> 3990 class_attribute Normal #> 3991 class_attribute Normal #> 3992 class_attribute Normal #> 3993 class_attribute Normal #> 3994 class_attribute Normal #> 3995 class_attribute Normal #> 3996 class_attribute Normal #> 3997 class_attribute Normal #> 3998 class_attribute Normal #> 3999 class_attribute Normal #> 4000 class_attribute Normal #> 4001 class_attribute Normal #> 4002 class_attribute Normal #> 4003 class_attribute Normal #> 4004 class_attribute Normal #> 4005 class_attribute Normal #> 4006 class_attribute Normal #> 4007 class_attribute Normal #> 4008 class_attribute Normal #> 4009 class_attribute Normal #> 4010 class_attribute Normal #> 4011 class_attribute Normal #> 4012 class_attribute Normal #> 4013 class_attribute Normal #> 4014 class_attribute Normal #> 4015 class_attribute Normal #> 4016 class_attribute Normal #> 4017 class_attribute Normal #> 4018 class_attribute Normal #> 4019 class_attribute Normal #> 4020 class_attribute Normal #> 4021 class_attribute Normal #> 4022 class_attribute Normal #> 4023 class_attribute Normal #> 4024 class_attribute Normal #> 4025 class_attribute Normal #> 4026 class_attribute Normal #> 4027 class_attribute Normal #> 4028 class_attribute Normal #> 4029 class_attribute Normal #> 4030 class_attribute Normal ``` ] .pull-right[ The `gather` function turns this wide dataset to a long dataset, stacking all the variables on top of each other ] --- ## Manipulating data for plotting We would like to get density plots of all the variables. .pull-left[ ```r dat_spine %>% select(pelvic_incidence:sacral_slope) %>% tidyr::gather(variable, value) %>% ggplot(aes(x = value)) + geom_density() + facet_wrap(~variable) + labs(x = '') ``` 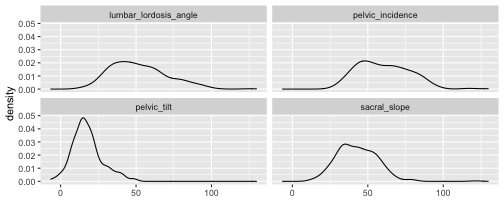<!-- --> ] .pull-right[ This is one of my most used tricks for getting facetted plots from wide data ] --- ## Re-ordering factors ```r beaches %>% ggplot(aes(x = season_name, y = temperature)) + geom_boxplot() + scale_y_continuous(labels = scales::unit_format(unit = "\u00B0C")) + labs(x = 'Season', y = 'Temperature') + theme_bw() + theme(axis.title = element_text(size = 16), axis.text = element_text(size = 14)) ``` 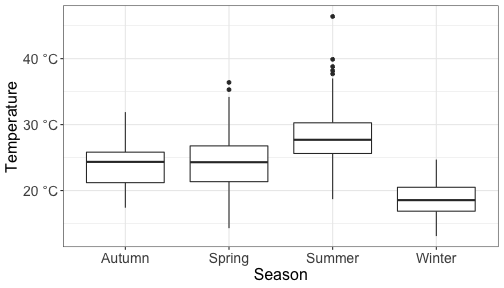<!-- --> --- ## Re-ordering factors ```r beaches %>% mutate(season_name = fct_relevel(season_name, * 'Autumn','Winter','Spring','Summer')) %>% ggplot(aes(x = season_name, y = temperature)) + geom_boxplot() + scale_y_continuous(labels = scales::unit_format(unit = "\u00B0C")) + labs(x = 'Season', y = 'Temperature') + theme_bw() + theme(axis.title = element_text(size = 16), axis.text = element_text(size = 14)) ``` 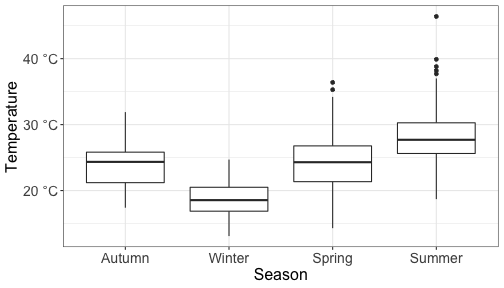<!-- -->